How to Remove Line Breaks Form a File in Java
-
Use the
replace()
Method to Remove Line Breaks From a File in Java -
line.separator
Method for Default Platform to Remove Line Breaks From a File in Java -
replaceAll()
Method for All Platform to Remove Line Breaks From a File in Java
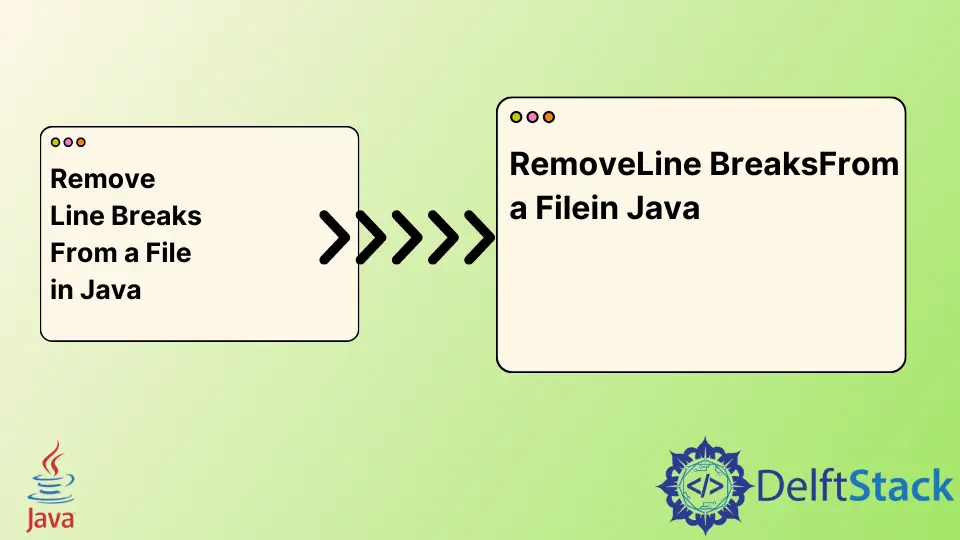
This tutorial mentions multiple methods to replace line breaks from any file in Java. We will be using the specified Java method with different results as per the requirement. We are using String instead of file to demonstrate those methods here. If you want to use it with file text, then replace String value with the file content.
Use the replace()
Method to Remove Line Breaks From a File in Java
The first method is replace()
for newline character removal. It is used with a condition where the user can pass all line breaks
of the file to be simply removed.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
String text = "abcdefghij\n"
+ "klmnopq\nzzzz";
System.out.println("**********Orignal Text**********");
System.out.println(text);
text = text.replace("\n", "");
System.out.println("*******************************");
System.out.println("**********Modified Text********");
System.out.println(text);
}
}
Output:
**********Orignal Text**********
abcdefghij
klmnopq
zzzz
*******************************
**********Modified Text********
abcdefghijklmnopqzzzz
line.separator
Method for Default Platform to Remove Line Breaks From a File in Java
Another approach is by using System.getProperty("line.separator")
inside replace()
method of Java. This method will process all the line separators (from Java property) and simply removes them.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
String text = "abcdefghij\n"
+ "klmnopq\nzzzz";
System.out.println("**********Orignal Text**********");
System.out.println(text);
text = text.replace(System.getProperty("line.separator").toString(), "");
System.out.println("*******************************");
System.out.println("**********Modified Text********");
System.out.println(text);
}
}
Output:
**********Orignal Text**********
abcdefghij
klmnopq
zzzz
*******************************
**********Modified Text********
abcdefghijklmnopqzzzz
replaceAll()
Method for All Platform to Remove Line Breaks From a File in Java
Another approach similar to the previous one is using the replaceAll()
method of Java. This method will process all the line separators used in any platform and simply removing these. This method is used if the requirement is to work on across all platforms like UNIX
, MAC OS
, Windows
, etc.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
String text = "abcdefghij\n"
+ "klmnopq\nzzzz";
System.out.println("**********Orignal Text**********");
System.out.println(text);
text = text.replaceAll("\\R", "");
System.out.println("*******************************");
System.out.println("**********Modified Text********");
System.out.println(text);
}
}
Output:
**********Orignal Text**********
abcdefghij
klmnopq
zzzz
*******************************
**********Modified Text********
abcdefghijklmnopqzzzz