FileFilter in Java
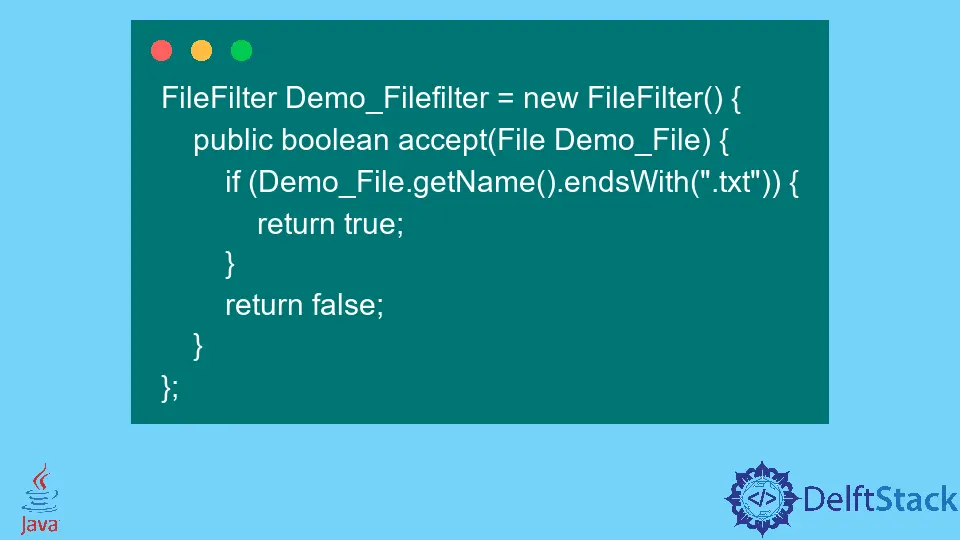
This tutorial demonstrates how to use FileFilter in Java.
FileFilter
is used to filter files with a particular extension. The Java built-in package IO and Apache Commons IO provide the classes and interfaces for FileFilter
to perform file filter operations.
FileFilter
From the IO Package in Java
The FileFilter
is a class from the Java IO package used as the filter for the files and subdirectories for the given path. The FileFilter
class takes File
objects and filters them based on the attributes like read-only, etc.
The FileFilter
has only one method, accept()
, which is used to check if the specified abstract should be included in the list or not. Here is an example of how to use the accept()
method:
FileFilter Demo_Filefilter = new FileFilter() {
public boolean accept(File Demo_File) {
if (Demo_File.getName().endsWith(".txt")) {
return true;
}
return false;
}
};
Now the best way to use the FileFilter
is to pass the FileFilter
to the listfiles()
method representing the directory location.
Let’s try a running example:
package delftstack;
import java.io.File;
import java.io.FileFilter;
public class Example {
public static void main(String[] args) {
File File_Directory = new File("C:\\Users\\Sheeraz\\eclipse-workspace\\Demos");
// check if the directory is valid directory
if (!(File_Directory.exists() && File_Directory.isDirectory())) {
System.out.println(String.format("This Directory does not exist", File_Directory));
return;
}
FileFilter Demo_Filefilter = new FileFilter() {
public boolean accept(File Demo_File) {
if (Demo_File.getName().endsWith(".txt")) {
return true;
}
return false;
}
};
File[] Text_Files = File_Directory.listFiles(Demo_Filefilter);
for (File Demo_File : Text_Files) {
System.out.println(Demo_File.getName());
}
}
}
The code above filters the files with the .txt
extension.
See output:
delftstack.txt
The FileFilter
can also be implemented using the lambda expression where the syntax is less and much easier.
See example:
package delftstack;
import java.io.File;
import java.io.FileFilter;
public class Example {
public static void main(String[] args) {
File File_Directory = new File("C:\\Users\\Sheeraz\\eclipse-workspace\\Demos");
// check if the directory is valid directory
if (!(File_Directory.exists() && File_Directory.isDirectory())) {
System.out.println(String.format("This Directory does not exist", File_Directory));
return;
}
FileFilter Demo_FileFilter = (Demo_File) -> {
return Demo_File.getName().endsWith(".txt");
};
File[] Text_Files = File_Directory.listFiles(Demo_FileFilter);
for (File Demo_File : Text_Files) {
System.out.println(Demo_File.getName());
}
}
}
The code above will work similarly and return the Files with the .txt
extension.
See output:
delftstack.txt
FileFilter
From the Apache Commons IO Package in Java
The FileFilter
package from Apache Commons-IO is used to perform the different file filter operations, which include choosing a file with a particular extension, a file starting or ending with a particular character, etc. The FileFilter
package from Apache Commons provides primitive and boolean filters, which means some of the filters will return a primitive value and some a boolean value.
See the tables below.
The primitive filters:
Method | Description |
---|---|
DirectoryFilter |
It will only accept directories. |
PrefixFileFilter |
It filters based on a prefix. |
SuffixFileFilter |
It filters based on a suffix. |
NameFileFilter |
It is used to filter based on a filename. |
WildcardFileFilter |
It is used to filter based on wildcards. |
AgeFileFilter |
It filters based on the last modified time of a file. |
SizeFileFilter |
It filters based on file size. |
The boolean filters:
Method | Description |
---|---|
TrueFileFilter |
It will accept all files. |
FalseFileFilter |
It will not accept files. |
NotFileFilter |
It will apply a logical NOT to an existing filter. |
AndFileFilter |
It will combine the two filters using a logical AND . |
OrFileFilter |
It will combine two filters using a logical OR . |
Let’s try to use a filter from the above methods in an example:
package delftstack;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.filefilter.SuffixFileFilter;
public class Example {
public static void main(String[] args) {
try {
Suffix_FileFilter();
} catch (IOException iox) {
System.out.println(iox.getMessage());
}
}
public static void Suffix_FileFilter() throws IOException {
// current directory
File Current_Directory = new File(".");
// all files and directories in the current directory
String[] All_Files = Current_Directory.list();
System.out.println("All files and directories :\n");
for (int i = 0; i < All_Files.length; i++) {
System.out.println(All_Files[i]);
}
System.out.println("\nThe Text Files: \n");
String[] Text_Files = Current_Directory.list(new SuffixFileFilter("txt"));
for (int i = 0; i < Text_Files.length; i++) {
System.out.println(Text_Files[i]);
}
}
}
The code above uses the SuffixFileFilter
to filter the text files from the given directory. It also lists all the files and subdirectories of the provided folder.
See the output:
All files and directories :
.classpath
.gitignore
.project
bin
delftstack.fxml
delftstack.fxml.bak
delftstack.pdf
Delftstack.png
delftstack.txt
delftstack.xml
delftstack.zip
DemoClassBytes.ser
employee.json
ExampleClassBytes.ser
Makefile
pom.xml
PythonFromJava.py
readme.md
src
student.json
The Text Files:
delftstack.txt
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook