How to Read Bytes From a File in Java
-
Use
FileInputStream
to Read Bytes From a File in Java -
Use the
Files
ClassreadAllBytes()
Method to Read Bytes From a File in Java - Use Apache Commons-IO to Read Bytes From a File in Java
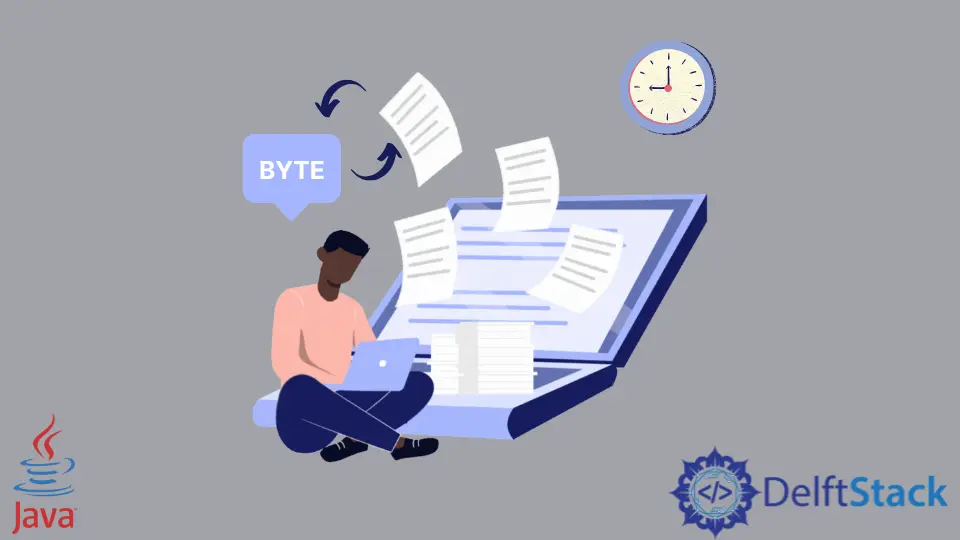
There are several methods in Java to read bytes from a file or to convert a file into bytes or bytes array.
This tutorial demonstrates different methods to read bytes from a file in Java.
Use FileInputStream
to Read Bytes From a File in Java
The FileInputStream
can read data from the given file using bytes. We can use this class’s FileInputStream.read()
method to read the bytes from a file in Java.
Follow the steps below to read bytes from a file using the FileInputStream
class.
- First, create an instance of the
FileInputStream
class. - Now, create a byte array. The length should be the same as the input file.
- Read the bytes from
FileInputStream
usingread()
method. - Print the byte array.
- Close the instance.
Let’s try to implement an example based on the above steps.
Our input file is in Rich Text Format
.
Hi, This is delftstack.com! The Best tutorial site.
The Java implementation:
package delftstack;
import java.io.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
File File_Path = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Instance of the FileInputStream
FileInputStream File_Input_Stream = new FileInputStream(File_Path);
// Create a byte array
byte[] Demo_Array = new byte[(int) File_Path.length()];
// Read file content to byte array
File_Input_Stream.read(Demo_Array);
// Close the instance
File_Input_Stream.close();
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
The code above will read the bytes from the given file into an array. See output:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100, 101,
108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 33, 32,
84, 104, 101, 32, 66, 101, 115, 116, 32, 116, 117, 116, 111,
114, 105, 97, 108, 32, 115, 105, 116, 101, 46]
Use the Files
Class readAllBytes()
Method to Read Bytes From a File in Java
The readAllBytes()
method reads all the bytes from a file in Java. Follow the steps below to read bytes from a file using the Files
class.
- Get the path using the
Paths.get()
method. - Read the bytes from the given file using
Files.readAllBytes()
into an array. - Print the byte array.
See the example:
package delftstack;
import java.io.*;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
Path File_Path = Paths.get("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = Files.readAllBytes(File_Path);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
The code will read the bytes from the given file using the Files.readAllBytes()
method. See output:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Use Apache Commons-IO to Read Bytes From a File in Java
The Apache Commons-IO
has a package, FileUtils
, which can be used to read all the bytes from a file in Java. Make sure Apache Commons-IO
is added to your Java environment.
Here is the maven dependency for Apache Commons-IO
; add it to your pom.xml
.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
Follow the steps below to read the bytes from a file using Apache Commons-IO
.
- Create a file using the
File
class with the given path. - Read the Bytes using the
FileUtils.readFileToByteArray()
with the file name as an input. - Print the byte array.
Here is the Java implementation for this example.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
File Demo_File = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = FileUtils.readFileToByteArray(Demo_File);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
The code will read the bytes from the given file using the Apache Commons-IO
library. See output:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook