Java의 파일에서 바이트 읽기
-
FileInputStream
을 사용하여 Java의 파일에서 바이트 읽기 -
파일
클래스readAllBytes()
메서드를 사용하여 Java의 파일에서 바이트 읽기 - Apache Commons-IO를 사용하여 Java의 파일에서 바이트 읽기
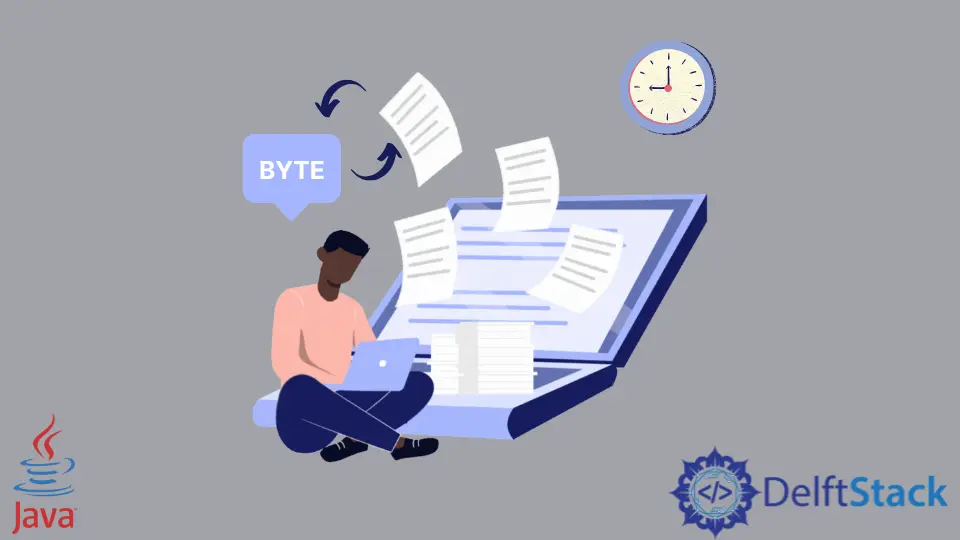
Java에는 파일에서 바이트를 읽거나 파일을 바이트 또는 바이트 배열로 변환하는 여러 가지 방법이 있습니다. 이 자습서는 Java에서 파일의 바이트를 읽는 다양한 방법을 보여줍니다.
FileInputStream
을 사용하여 Java의 파일에서 바이트 읽기
FileInputStream
은 바이트를 사용하여 주어진 파일에서 데이터를 읽을 수 있습니다. 이 클래스의 FileInputStream.read()
메서드를 사용하여 Java의 파일에서 바이트를 읽을 수 있습니다.
FileInputStream
클래스를 사용하여 파일에서 바이트를 읽으려면 아래 단계를 따르십시오.
- 먼저
FileInputStream
클래스의 인스턴스를 만듭니다. - 이제 바이트 배열을 만듭니다. 길이는 입력 파일과 동일해야 합니다.
read()
메서드를 사용하여FileInputStream
에서 바이트를 읽습니다.- 바이트 배열을 인쇄합니다.
- 인스턴스를 닫습니다.
위의 단계를 기반으로 예제를 구현해 보겠습니다.
입력 파일은 서식 있는 텍스트 형식
입니다.
Hi, This is delftstack.com! The Best tutorial site.
자바 구현:
package delftstack;
import java.io.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
File File_Path = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Instance of the FileInputStream
FileInputStream File_Input_Stream = new FileInputStream(File_Path);
// Create a byte array
byte[] Demo_Array = new byte[(int) File_Path.length()];
// Read file content to byte array
File_Input_Stream.read(Demo_Array);
// Close the instance
File_Input_Stream.close();
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
위의 코드는 주어진 파일의 바이트를 배열로 읽습니다. 출력 참조:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100, 101,
108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 33, 32,
84, 104, 101, 32, 66, 101, 115, 116, 32, 116, 117, 116, 111,
114, 105, 97, 108, 32, 115, 105, 116, 101, 46]
파일
클래스 readAllBytes()
메서드를 사용하여 Java의 파일에서 바이트 읽기
readAllBytes()
메서드는 Java의 파일에서 모든 바이트를 읽습니다. Files
클래스를 사용하여 파일에서 바이트를 읽으려면 아래 단계를 따르십시오.
Paths.get()
메서드를 사용하여 경로를 가져옵니다.Files.readAllBytes()
를 사용하여 주어진 파일에서 바이트를 배열로 읽습니다.- 바이트 배열을 인쇄합니다.
예를 참조하십시오.
package delftstack;
import java.io.*;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
Path File_Path = Paths.get("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = Files.readAllBytes(File_Path);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
코드는 Files.readAllBytes()
메서드를 사용하여 주어진 파일에서 바이트를 읽습니다. 출력 참조:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Apache Commons-IO를 사용하여 Java의 파일에서 바이트 읽기
Apache Commons-IO
에는 Java의 파일에서 모든 바이트를 읽는 데 사용할 수 있는 FileUtils
패키지가 있습니다. Apache Commons-IO
가 Java 환경에 추가되었는지 확인하십시오.
다음은 Apache Commons-IO
에 대한 maven 종속성입니다. pom.xml
에 추가하십시오.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
Apache Commons-IO
를 사용하여 파일에서 바이트를 읽으려면 아래 단계를 따르십시오.
- 주어진 경로로
File
클래스를 사용하여 파일을 만듭니다. - 파일 이름을 입력으로 사용하여
FileUtils.readFileToByteArray()
를 사용하여 바이트를 읽습니다. - 바이트 배열을 인쇄합니다.
다음은 이 예제에 대한 Java 구현입니다.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
File Demo_File = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = FileUtils.readFileToByteArray(Demo_File);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
코드는 Apache Commons-IO
라이브러리를 사용하여 주어진 파일에서 바이트를 읽습니다. 출력 참조:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook