Leer bytes de un archivo en Java
-
Use
FileInputStream
para leer bytes de un archivo en Java -
Utilice el método
Files
ClassreadAllBytes()
para leer bytes de un archivo en Java - Use Apache Commons-IO para leer bytes de un archivo en Java
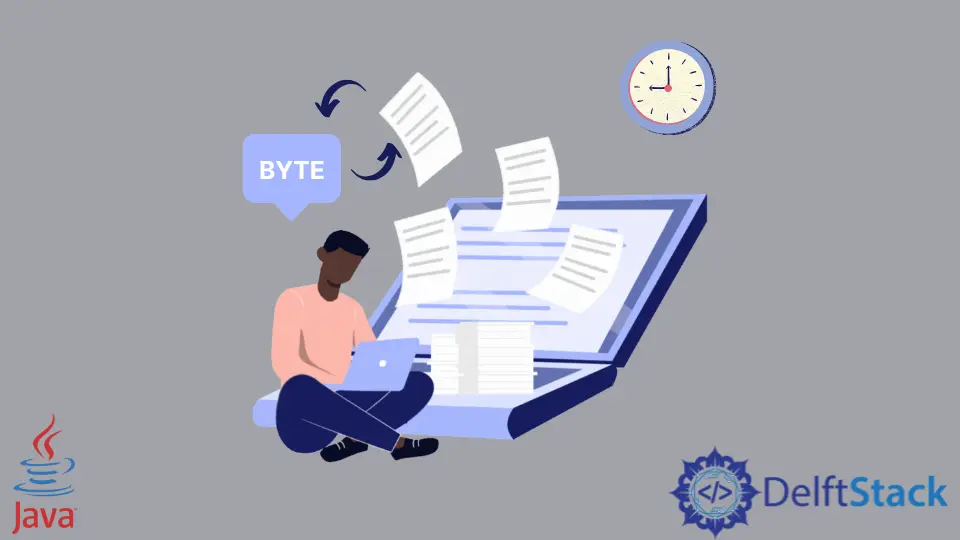
Hay varios métodos en Java para leer bytes de un archivo o para convertir un archivo en bytes o en una matriz de bytes. Este tutorial demuestra diferentes métodos para leer bytes de un archivo en Java.
Use FileInputStream
para leer bytes de un archivo en Java
El FileInputStream
puede leer datos del archivo dado usando bytes. Podemos usar el método FileInputStream.read()
de esta clase para leer los bytes de un archivo en Java.
Siga los pasos a continuación para leer bytes de un archivo utilizando la clase FileInputStream
.
- Primero, crea una instancia de la clase
FileInputStream
. - Ahora, cree una matriz de bytes. La longitud debe ser la misma que la del archivo de entrada.
- Lea los bytes de
FileInputStream
utilizando el métodoread()
. - Imprima la matriz de bytes.
- Cierre la instancia.
Intentemos implementar un ejemplo basado en los pasos anteriores.
Nuestro archivo de entrada está en Formato de texto enriquecido
.
Hi, This is delftstack.com! The Best tutorial site.
La implementación de Java:
package delftstack;
import java.io.*;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
File File_Path = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Instance of the FileInputStream
FileInputStream File_Input_Stream = new FileInputStream(File_Path);
// Create a byte array
byte[] Demo_Array = new byte[(int) File_Path.length()];
// Read file content to byte array
File_Input_Stream.read(Demo_Array);
// Close the instance
File_Input_Stream.close();
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
El código anterior leerá los bytes del archivo dado en una matriz. Ver salida:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115, 32, 100, 101,
108, 102, 116, 115, 116, 97, 99, 107, 46, 99, 111, 109, 33, 32,
84, 104, 101, 32, 66, 101, 115, 116, 32, 116, 117, 116, 111,
114, 105, 97, 108, 32, 115, 105, 116, 101, 46]
Utilice el método Files
Class readAllBytes()
para leer bytes de un archivo en Java
El método readAllBytes()
lee todos los bytes de un archivo en Java. Siga los pasos a continuación para leer bytes de un archivo usando la clase Files
.
- Obtenga la ruta usando el método
Paths.get()
. - Lea los bytes del archivo dado usando
Files.readAllBytes()
en una matriz. - Imprima la matriz de bytes.
Ver el ejemplo:
package delftstack;
import java.io.*;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Arrays;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
Path File_Path = Paths.get("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = Files.readAllBytes(File_Path);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
El código leerá los bytes del archivo dado usando el método Files.readAllBytes()
. Ver salida:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Use Apache Commons-IO para leer bytes de un archivo en Java
El Apache Commons-IO
tiene un paquete, FileUtils
, que se puede utilizar para leer todos los bytes de un archivo en Java. Asegúrese de agregar Apache Commons-IO
a su entorno Java.
Aquí está la dependencia maven para Apache Commons-IO
; añádelo a tu pom.xml
.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.7</version>
</dependency>
Siga los pasos a continuación para leer los bytes de un archivo usando Apache Commons-IO
.
- Cree un archivo usando la clase
Archivo
con la ruta dada. - Lea los Bytes utilizando
FileUtils.readFileToByteArray()
con el nombre del archivo como entrada. - Imprima la matriz de bytes.
Aquí está la implementación de Java para este ejemplo.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.util.Arrays;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) throws IOException {
// Create the path
File Demo_File = new File("C:\\Users\\Sheeraz\\delftstack.rtf");
// Read Bytes
byte[] Demo_Array = FileUtils.readFileToByteArray(Demo_File);
// Print the above byte array
System.out.print(Arrays.toString(Demo_Array));
}
}
El código leerá los bytes del archivo dado usando la biblioteca Apache Commons-IO
. Ver salida:
[72, 105, 44, 32, 84, 104, 105, 115, 32, 105, 115,
32, 100, 101, 108, 102, 116, 115, 116, 97, 99, 107,
46, 99, 111, 109, 33, 32, 84, 104, 101, 32, 66, 101,
115, 116, 32, 116, 117, 116, 111, 114, 105, 97, 108,
32, 115, 105, 116, 101, 46]
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook