Java에서 두 파일의 내용 비교
- Java에서 두 파일의 바이트 단위 비교
- Java에서 두 파일의 라인별 비교
-
Java에서
Files.mismatch()
를 사용하여 두 파일 비교 - Java에서 Apache Commons IO와 두 파일 비교
-
Java에서
Arrays.equals()
를 사용하여 두 파일 비교
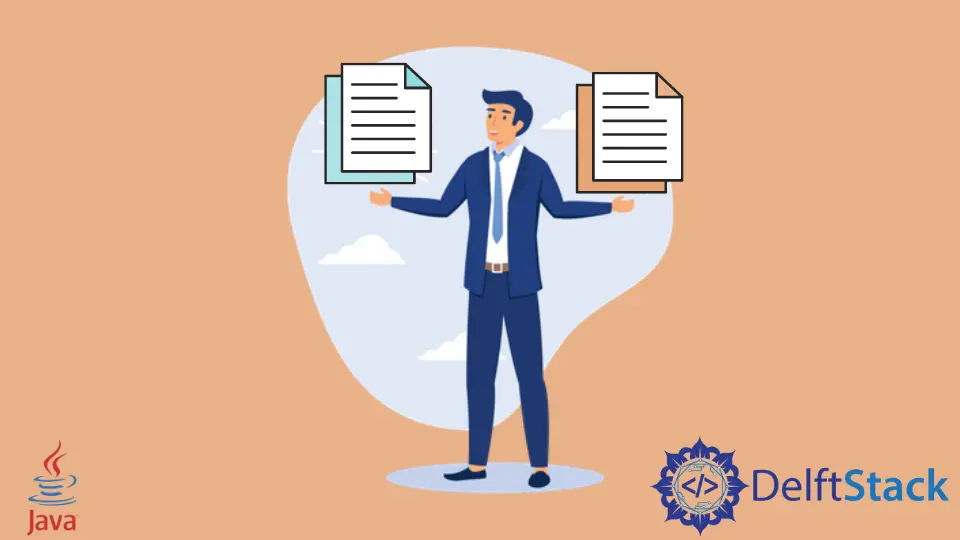
두 파일의 내용을 비교하는 방법에는 여러 가지가 있습니다. 이 자습서는 Java에서 두 파일을 비교하는 다양한 방법을 보여줍니다.
Java에서 두 파일의 바이트 단위 비교
BufferedInputStream
은 파일을 읽고 한 파일의 각 바이트를 다른 파일과 비교하는 데 사용됩니다. BufferedReader
의 read()
메서드를 사용하여 각 바이트를 읽고 비교합니다.
delftstack1
및 delftstack2
라는 이름이 다른 두 개의 유사한 텍스트 파일을 만들었으며 내용은 다음과 같습니다.
Hello This is delftstack.com
The best online platform for learning different programming languages.
두 파일을 바이트 단위로 비교하는 예제를 시도해 봅시다.
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFilesbyByte(Path File_One, Path File_Two) {
try {
long file_size = Files.size(File_One);
if (file_size != Files.size(File_Two)) {
return false;
}
if (file_size < 2048) {
return Arrays.equals(Files.readAllBytes(File_One), Files.readAllBytes(File_Two));
}
// Compare byte-by-byte
try (BufferedReader Content1 = Files.newBufferedReader(File_One);
BufferedReader Content2 = Files.newBufferedReader(File_Two)) {
int byt;
while ((byt = Content1.read()) != -1) {
if (byt != Content2.read()) {
return false;
}
}
}
return true;
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt"); // Path to file one
File File_Two = new File("delftstack2.txt"); // Path to file two
boolean Comparison = CompareFilesbyByte(File_One.toPath(), File_Two.toPath());
if (Comparison) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal");
}
}
}
코드는 파일을 바이트 단위로 비교합니다. 출력은 다음과 같습니다.
The Input files are equal.
Java에서 두 파일의 라인별 비교
마찬가지로 BufferedInputStream
은 파일을 읽고 한 파일의 각 줄을 다른 파일의 각 줄과 비교하는 데에도 사용됩니다. BufferedReader
의 readline()
메서드를 사용하여 각 줄을 읽고 비교합니다.
예를 보겠습니다.
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFilesbyByte(Path File_One, Path File_Two) {
try {
long file_size = Files.size(File_One);
if (file_size != Files.size(File_Two)) {
return false;
}
if (file_size < 2048) {
return Arrays.equals(Files.readAllBytes(File_One), Files.readAllBytes(File_Two));
}
// Compare byte-by-byte
try (BufferedReader Content1 = Files.newBufferedReader(File_One);
BufferedReader Content2 = Files.newBufferedReader(File_Two)) {
String line;
while ((line = Content1.readLine()) != null) {
if (line != Content2.readLine()) {
return false;
}
}
}
return true;
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt"); // Path to file one
File File_Two = new File("delftstack2.txt"); // Path to file two
boolean Comparison = CompareFilesbyByte(File_One.toPath(), File_Two.toPath());
if (Comparison) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal");
}
}
}
위의 코드는 두 파일을 한 줄씩 비교합니다. 파일의 내용이 동일하므로 출력은 다음과 같습니다.
The Input files are equal.
Java에서 Files.mismatch()
를 사용하여 두 파일 비교
최근 Java 12는 두 파일을 비교하기 위해 java.nio.file.Files
클래스에 Files.mismatch
메서드를 추가했습니다.
이 방법은 파일을 입력으로 사용하고 주어진 파일의 내용을 비교합니다. 두 파일이 같으면 -1
을 반환하고 그렇지 않으면 일치하지 않는 첫 번째 바이트의 위치를 반환합니다.
내용이 다른 세 번째 파일을 만들었습니다. 서로 다른 파일을 비교하는 예를 살펴보겠습니다.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
public class File_Compare {
public static void main(String[] args) throws IOException {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
File File_Three = new File("delftstack3.txt");
long Compare1 = Files.mismatch(File_One.toPath(), File_Two.toPath());
System.out.println(Compare1);
long Compare2 = Files.mismatch(File_Two.toPath(), File_Three.toPath());
System.out.println(Compare2);
}
}
위의 코드는 먼저 -1
을 반환해야 하는 두 개의 유사한 파일을 비교한 다음 일치하지 않는 첫 번째 바이트의 위치를 반환해야 하는 두 개의 다른 파일을 비교합니다. 출력 참조:
-1
34
Java에서 Apache Commons IO와 두 파일 비교
Apache Commons IO의 FileUtils
클래스는 파일 작업에 사용됩니다. contentEquals()
메서드는 두 파일의 내용이 동일하거나 두 파일이 모두 존재하지 않는 경우 true
를 반환하는 두 파일을 비교하는 데 사용할 수 있습니다.
예를 들어 보겠습니다.
package delftstack;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
public class File_Compare {
public static void main(String[] args) throws IOException {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
boolean Comapre_Result = FileUtils.contentEquals(File_One, File_Two);
if (Comapre_Result) {
System.out.println("The input files are equal.");
} else {
System.out.println("The input files are not equal.");
}
}
}
이 코드는 두 파일을 비교하고 Apache FileUtils
클래스를 사용하여 동일한지 여부를 확인합니다. 출력 참조:
The input files are equal.
Java에서 Arrays.equals()
를 사용하여 두 파일 비교
전체 파일을 바이트 배열로 읽은 다음 이러한 배열이 동일한지 비교할 수 있습니다. Files.readAllBytes()
를 사용하여 파일을 바이트 배열로 읽고 Arrays.equals()
를 사용하여 동등성을 확인할 수 있습니다.
이 방법은 큰 파일에는 권장되지 않습니다. 예를 참조하십시오:
package delftstack;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFileArrays(Path File_One, Path File_Two) {
try {
if (Files.size(File_One) != Files.size(File_Two)) {
return false;
}
byte[] First_File = Files.readAllBytes(File_One);
byte[] Second_File = Files.readAllBytes(File_Two);
return Arrays.equals(First_File, Second_File);
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
boolean Compare_Result = CompareFileArrays(File_One.toPath(), File_Two.toPath());
if (Compare_Result) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal.");
}
}
}
위의 코드는 파일을 바이트 배열로 읽은 다음 해당 배열을 비교합니다. 출력 참조:
The Input files are equal.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook