Java에서 파일 크기 얻기
-
Java IO에서
파일
클래스를 사용하여 파일 크기 가져오기 -
Java NIO에서
파일
클래스를 사용하여 파일 크기 가져오기 -
Apache Commons IO에서
FileUtils
클래스를 사용하여 파일 크기 가져오기
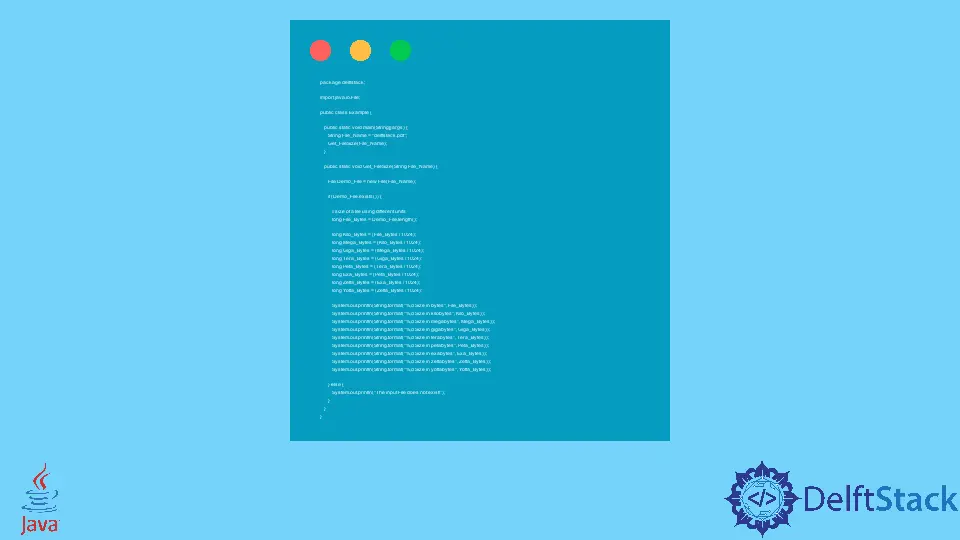
Java는 파일 크기를 바이트 단위로 가져오는 다양한 방법을 제공합니다. 이 자습서는 Java에서 파일 크기를 가져오는 다양한 방법을 보여줍니다.
Java IO에서 파일
클래스를 사용하여 파일 크기 가져오기
Java IO 패키지의 File
클래스는 파일 크기를 바이트 단위로 가져오는 기능을 제공합니다. File
클래스에는 파일 크기를 바이트 단위로 반환하는 메서드 길이가 있습니다.
File
이 디렉토리인 경우 length()
메서드는 지정되지 않은 값을 반환합니다. 따라서 크기를 확인하기 전에 파일이 존재하고 디렉토리가 아닌지 확인하십시오.
크기가 240킬로바이트인 delftstack.pdf
파일을 선택했습니다. Java의 File
클래스를 사용하여 이 파일의 크기를 확인하는 예제를 시도해 보겠습니다.
package delftstack;
import java.io.File;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
if (Demo_File.exists()) {
// size of a file using different units
long File_Bytes = Demo_File.length();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} else {
System.out.println("The input File does not exist!");
}
}
}
위의 코드는 먼저 Java IO를 사용하여 주어진 파일의 크기를 바이트 단위로 계산한 다음 다른 단위로 변환합니다. 출력 참조:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Java NIO에서 파일
클래스를 사용하여 파일 크기 가져오기
Java NIO 패키지 클래스 Files
에는 파일 크기를 가져오는 size()
메서드가 있습니다. size()
메서드는 파일 크기를 바이트 단위로 반환합니다.
동일한 파일로 예제를 시도해 보겠습니다.
package delftstack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
try {
// size of a file using different units
long File_Bytes = Files.size(File_Path);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
위의 코드는 Java NIO 패키지의 Files.size()
메서드를 사용하여 Java에서 파일 크기를 가져옵니다. 출력 참조:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
NIO 패키지에는 Java에서 파일 크기를 가져오는 데 사용할 수 있는 또 다른 클래스인 FileChannel
도 있습니다. Java에서 파일 크기를 가져오는 비슷한 메서드 size()
가 있습니다.
package delftstack;
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
FileChannel File_Channel;
try {
// size of a file using different units
File_Channel = FileChannel.open(File_Path);
long File_Bytes = File_Channel.size();
File_Channel.close();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
위의 코드는 NIO 패키지의 FileChannel
클래스를 사용하여 크기를 바이트 단위로 가져옵니다. 출력 참조:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Apache Commons IO에서 FileUtils
클래스를 사용하여 파일 크기 가져오기
Apache Commons IO
는 많은 기능을 제공하며 FileUtils
는 Java에서 파일 작업을 처리하는 데 사용되는 기능 중 하나입니다. Apache Commons의 FileUtils
클래스에는 파일 크기를 바이트 단위로 가져올 수 있는 Sizeof()
메서드가 있습니다.
Apache Commons IO를 사용하여 Java에서 파일 크기를 가져오는 예를 살펴보겠습니다.
package delftstack;
import java.io.File;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
try {
// size of a file using different units
long File_Bytes = FileUtils.sizeOf(Demo_File);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
위의 코드는 Java의 FileUtils
클래스를 사용하여 주어진 파일의 크기를 가져옵니다. 출력 참조:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook