Obtener tamaño de archivo en Java
-
Obtenga el tamaño del archivo usando la clase
Archivo
de Java IO -
Obtenga el tamaño del archivo usando la clase
Files
de Java NIO -
Obtenga el tamaño del archivo usando la clase
FileUtils
de Apache Commons IO
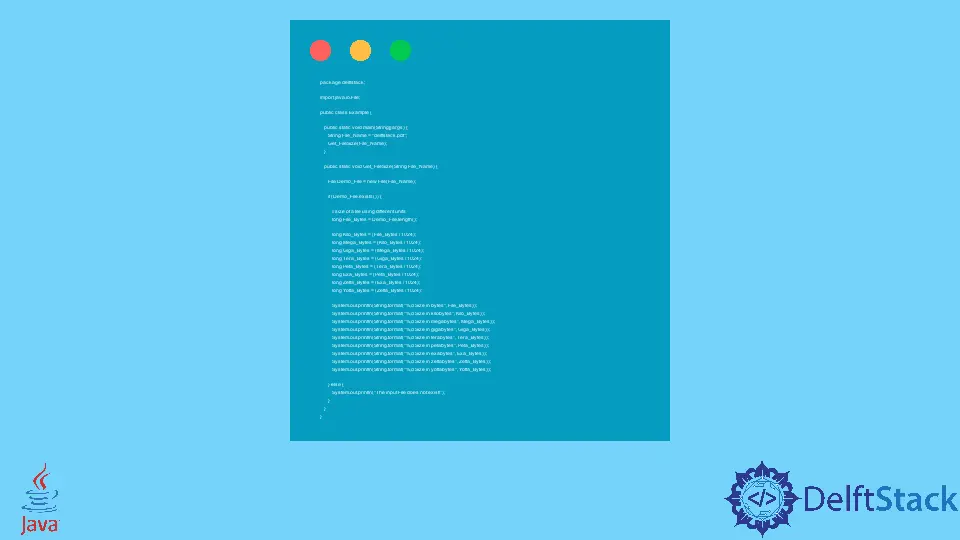
Java proporciona diferentes métodos para obtener el tamaño de un archivo en bytes. Este tutorial demuestra diferentes métodos para obtener el tamaño de archivo en Java.
Obtenga el tamaño del archivo usando la clase Archivo
de Java IO
La clase File
del paquete Java IO proporciona la funcionalidad para obtener el tamaño de un archivo en bytes. La clase File
tiene una longitud de método que devuelve el tamaño de un archivo en bytes.
Si el Archivo
es un directorio, entonces el método longitud()
devolverá un valor no especificado. Entonces, antes de verificar el tamaño, asegúrese de que el archivo exista y no sea un directorio.
Hemos seleccionado un archivo delftstack.pdf
con un tamaño de 240 kilobytes. Probemos un ejemplo para verificar el tamaño de este archivo usando la clase File
de Java:
package delftstack;
import java.io.File;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
if (Demo_File.exists()) {
// size of a file using different units
long File_Bytes = Demo_File.length();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} else {
System.out.println("The input File does not exist!");
}
}
}
El código anterior primero calculará el tamaño del archivo dado en bytes usando Java IO y luego lo convertirá a otras unidades. Ver salida:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Obtenga el tamaño del archivo usando la clase Files
de Java NIO
La clase del paquete Java NIO Files
tiene un método size()
para obtener el tamaño de un archivo. El método size()
devolverá el tamaño del archivo en bytes.
Probemos un ejemplo con el mismo archivo:
package delftstack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
try {
// size of a file using different units
long File_Bytes = Files.size(File_Path);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
El código anterior utiliza el método Files.size()
del paquete Java NIO para obtener el tamaño de un archivo en Java. Ver salida:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
El paquete NIO también tiene otra clase, FileChannel
, que también se puede usar para obtener el tamaño de un archivo en Java. Tiene un método similar size()
para obtener el tamaño de un archivo en Java:
package delftstack;
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
FileChannel File_Channel;
try {
// size of a file using different units
File_Channel = FileChannel.open(File_Path);
long File_Bytes = File_Channel.size();
File_Channel.close();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
El código anterior usa la clase FileChannel
del paquete NIO para obtener el tamaño en bytes. Ver salida:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Obtenga el tamaño del archivo usando la clase FileUtils
de Apache Commons IO
El Apache Commons IO
proporciona muchas funcionalidades, y FileUtils
es una de ellas que se usa para manejar las operaciones de archivos en Java. La clase FileUtils
de Apache Commons tiene un método Sizeof()
, que puede obtener el tamaño de un archivo en bytes.
Probemos un ejemplo usando Apache Commons IO para obtener el tamaño de un archivo en Java:
package delftstack;
import java.io.File;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
try {
// size of a file using different units
long File_Bytes = FileUtils.sizeOf(Demo_File);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
El código anterior usa la clase FileUtils
de Java para obtener el tamaño del archivo dado. Ver salida:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook