How to Get File Size in Java
-
Get File Size Using the
File
Class From Java IO -
Get File Size Using the
Files
Class From Java NIO -
Get File Size Using the
FileUtils
Class From Apache Commons IO
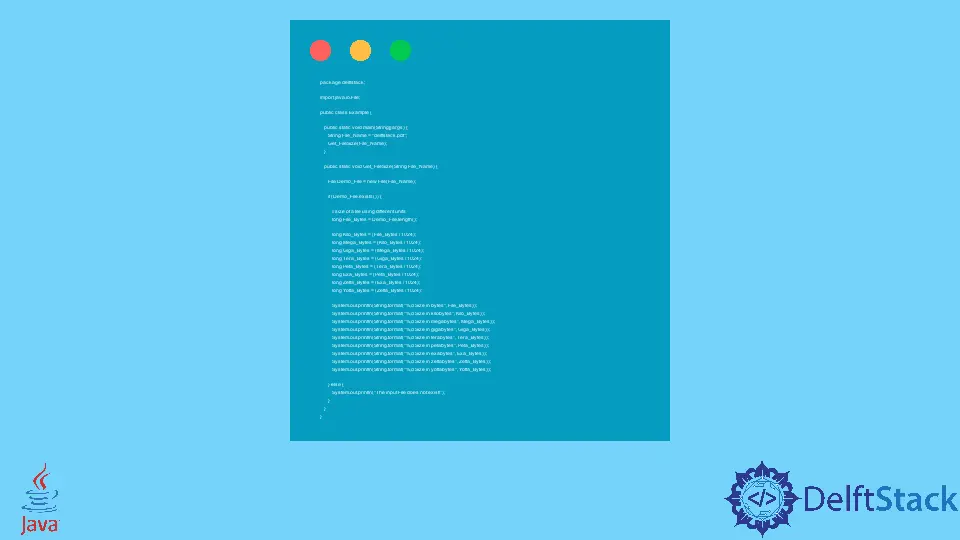
Java provides different methods to get the size of a file in bytes.
This tutorial demonstrates different methods to get file size in Java.
Get File Size Using the File
Class From Java IO
The File
class of the Java IO package provides the functionality to get the size of a file in bytes. The File
class has a method length that returns the size of a file in bytes.
If the File
is a directory, then the length()
method will return an unspecified value. So before checking the size, make sure the file exists and is not a directory.
We have selected a file delftstack.pdf
with a size of 240 kilobytes. Let’s try an example to check the size of this file using the File
class of Java:
package delftstack;
import java.io.File;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
if (Demo_File.exists()) {
// size of a file using different units
long File_Bytes = Demo_File.length();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} else {
System.out.println("The input File does not exist!");
}
}
}
The code above will first calculate the size of the given file in bytes using Java IO and then convert it to other units. See output:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Get File Size Using the Files
Class From Java NIO
The Java NIO package class Files
has a method size()
to get the size of a file. The size()
method will return the file size in bytes.
Let’s try an example with the same file:
package delftstack;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
try {
// size of a file using different units
long File_Bytes = Files.size(File_Path);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above uses the Files.size()
method from the Java NIO package to get the size of a file in Java. See output:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
The NIO package also has another class, FileChannel
, which also can be used to get the size of a file in Java. It has a similar method size()
to get the size of a file in Java:
package delftstack;
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.nio.file.Path;
import java.nio.file.Paths;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
Path File_Path = Paths.get(File_Name);
FileChannel File_Channel;
try {
// size of a file using different units
File_Channel = FileChannel.open(File_Path);
long File_Bytes = File_Channel.size();
File_Channel.close();
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above uses the FileChannel
class from the NIO package to get the size in Bytes. See output:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Get File Size Using the FileUtils
Class From Apache Commons IO
The Apache Commons IO
provides a lot of functionalities, and FileUtils
is one of them used to handle the file operations in Java. The FileUtils
class of Apache Commons has a method Sizeof()
, which can get the size of a file in bytes.
Let’s try an example using Apache Commons IO to get the size of a file in Java:
package delftstack;
import java.io.File;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) {
String File_Name = "delftstack.pdf";
Get_FileSize(File_Name);
}
public static void Get_FileSize(String File_Name) {
File Demo_File = new File(File_Name);
try {
// size of a file using different units
long File_Bytes = FileUtils.sizeOf(Demo_File);
long Kilo_Bytes = (File_Bytes / 1024);
long Mega_Bytes = (Kilo_Bytes / 1024);
long Giga_Bytes = (Mega_Bytes / 1024);
long Tera_Bytes = (Giga_Bytes / 1024);
long Peta_Bytes = (Tera_Bytes / 1024);
long Exa_Bytes = (Peta_Bytes / 1024);
long Zetta_Bytes = (Exa_Bytes / 1024);
long Yotta_Bytes = (Zetta_Bytes / 1024);
System.out.println(String.format("%,d Size in bytes", File_Bytes));
System.out.println(String.format("%,d Size in kilobytes", Kilo_Bytes));
System.out.println(String.format("%,d Size in megabytes", Mega_Bytes));
System.out.println(String.format("%,d Size in gigabytes", Giga_Bytes));
System.out.println(String.format("%,d Size in terabytes", Tera_Bytes));
System.out.println(String.format("%,d Size in petabytes", Peta_Bytes));
System.out.println(String.format("%,d Size in exabytes", Exa_Bytes));
System.out.println(String.format("%,d Size in zettabytes", Zetta_Bytes));
System.out.println(String.format("%,d Size in yottabytes", Yotta_Bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above uses the FileUtils
class of Java to get the size of the given file. See output:
245,681 Size in bytes
239 Size in kilobytes
0 Size in megabytes
0 Size in gigabytes
0 Size in terabytes
0 Size in petabytes
0 Size in exabytes
0 Size in zettabytes
0 Size in yottabytes
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook