How to Delete Files in a Directory Using Java
-
Delete Files in a Directory Using
delete()
ofFile
Class in Java - Delete Files in a Directory Using Java 8 Streams and NIO2
- Delete Files in a Directory Using Apache Common IO
- Conclusion
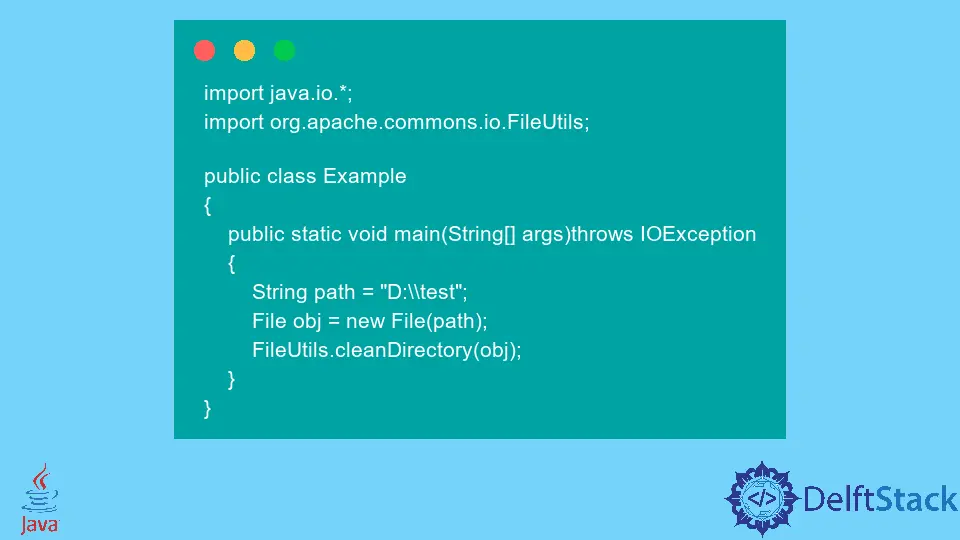
In this article, we will learn how to delete files present inside the folder without deleting the folder itself.
There are multiple ways to do this. Let’s look at them one by one.
Delete Files in a Directory Using delete()
of File
Class in Java
In Java, we have the java.io.File
class, which contains a method called delete()
used to delete files and empty directories.
Syntax:
file_object.delete()
Let’s assume in our system’s D:
drive a directory with a name as test
exists, and let’s say it contains some text files and some sub-folders. Now, let’s see how to delete this using the delete()
method.
Example Code:
import java.io.File;
public class Example {
public static void main(String[] args) {
String path = "D:\\test";
File obj = new File(path);
for (File temp : Objects.requireNonNull(obj.listFiles())) {
if (!temp.isDirectory()) {
temp.delete();
}
}
}
}
When the above code is executed, we can see that all the files inside the test
are deleted, but the main folder test
and the sub-folders are untouched.
In the above, we have created a string variable that stores the path
of the directory. Then we used this path to create our file object obj
.
Then we used the listFiles()
method to list the contents present at that path.
Using the if
condition, we check if it’s a directory or a file. If it is a file, we delete it; else, we do nothing.
Delete Files in a Directory Using Java 8 Streams and NIO2
In this method, we can use the Files.walk(Path)
method that returns Stream<Path>
, which contains all the files and sub-folders present in that path
.
Then we check if it’s a directory or a file using the if
condition. If it is a file, we delete it; else, we do nothing.
Example Code:
import java.io.*;
import java.nio.file.*;
import java.util.*;
public class Demo {
public static void main(String[] args) throws IOException {
Path path = Paths.get("D:\\test");
Files.walk(path).sorted(Comparator.reverseOrder()).forEach(data -> {
try {
if (!Files.isDirectory(data)) {
System.out.println("deleting: " + data);
Files.delete(data);
}
} catch (IOException obj) {
obj.printStackTrace();
}
});
}
}
Output:
deleting: D:\test\subfolder 2\file4.txt
deleting: D:\test\subfolder 1\file3.txt
deleting: D:\test\file2.txt
deleting: D:\test\file1.txt
When the above code is executed, it deletes all the files of the directory and sub-directory files in a Depth First Search fashion.
We can observe that the directory test
and the sub-directories subfolder 1
and subfolder 2
remained intact.
Delete Files in a Directory Using Apache Common IO
So far, all the methods we have seen are plain old Java methods that use some concepts of recursion along with file
and stream
methods. But we can use Apache Common IO FileUtils.cleanDirectory()
to recursively delete all the files and the sub-directories within the main directory without deleting the main directory itself.
The main advantage of using this over primitive Java methods is that the line of codes (LOC) is significantly less, making it an easy and more efficient way of writing.
To use Apache common IO, we must first add the dependencies in our pom.xml
file.
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.11.0</version>
</dependency>
Example Code:
import java.io.*;
import org.apache.commons.io.FileUtils;
public class Example {
public static void main(String[] args) throws IOException {
String path = "D:\\test";
File obj = new File(path);
FileUtils.cleanDirectory(obj);
}
}
Conclusion
This article has shown different ways of deleting directories using Java. We understood how to use the delete()
method and Java 8 streams and how using Apache commons IO could be more efficient and time-saving where LOC (line of codes) greatly affects the performance of our project.