File Separator in Java
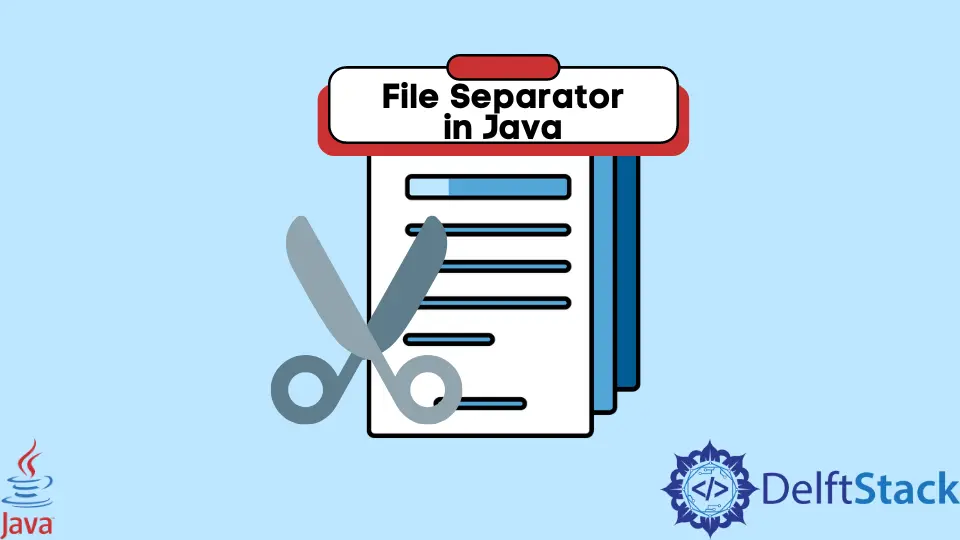
This tutorial demonstrates the file separator in Java.
File Separator in Java
The File Separator is a character used to separate directories; for example, Unix uses /
, and Windows uses \
as the file separator. Different Operating system uses different file separators, so we must handle them properly.
Java provides methods to properly handle the files and paths so the code can be used on a different platform. This tutorial demonstrates different methods for the file separator in Java.
File Separator with System Property
To get the file separator, Java has a system property, file.separator
. If we use this property on Unix, it will return /,
and on Windows, it will return \
.
Let’s try an example:
package delftstack;
public class Example {
public static void main(String[] args) {
// windows \, unix /
String File_Separator = System.getProperty("file.separator");
System.out.println("The file separator used for this system is: " + File_Separator);
}
}
The code above will get the file separator and print it using the System Properties. See output:
The file separator used for this system is: \
File Separator with Java IO
The Java IO package also has the functionality to get the file separator. The method File.separator
from the File
class will return the file separator.
Let’s try an example of a file separator with Java IO:
package delftstack;
import java.io.File;
public class Example {
public static void main(String[] args) {
// windows \, unix /
String File_Separator = File.separator;
System.out.println("The file separator used for this system is: " + File_Separator);
}
}
The code above will get the file separator and print it using the Java NIO package. See output:
The file separator used for this system is: \
The file separator with system property can be overridden using the System.setProperty()
. And the file separator with both IO and NIO packages cannot be overridden; it will always return the corresponding file separator.
Difference Between File Separator and Path Separator
The file separator can only be the two characters /
or \
, and the path separator can be these or some other characters. Both of them have differences in uses:
- The File Separator is one of the characters
/
or\
, which is used to split a path to a specific file. For example, on Windows,C:\Users\Sheeraz
. - The Path separator separates an individual file path from a full list of file paths. For example, when setting the environment variable
PATH
, we use;
as a path separator for different paths, so the path separator there is;
.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook