How to Convert Bytes to Unsigned Bytes in Java
-
Convert Bytes to Unsigned Bytes Using Bitwise
AND
With0xff
-
Convert Bytes to Unsigned Bytes Using
Integer.parseInt
WithRadix16
-
Convert Bytes to Unsigned Bytes Using
Byte.toUnsignedInt
- Conclusion
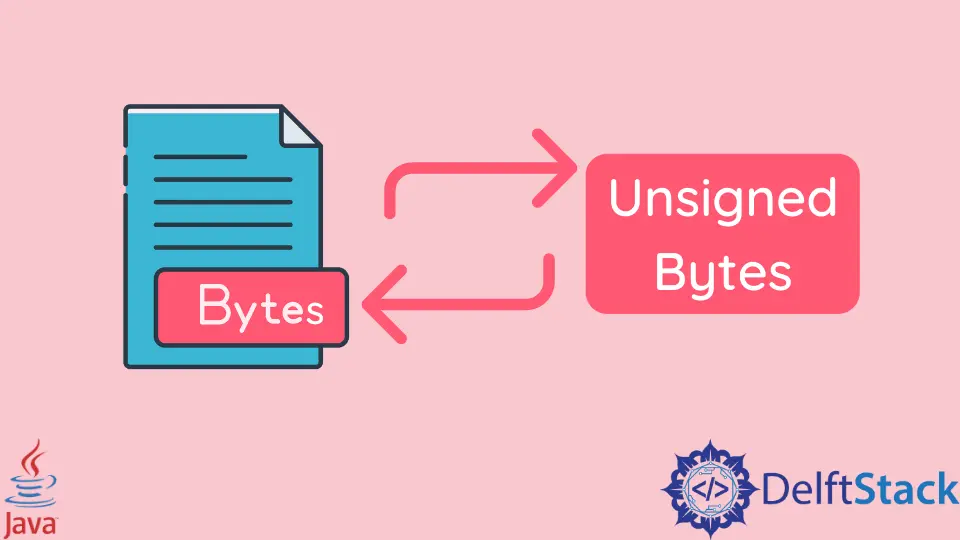
In Java programming, various methods exist to convert bytes to unsigned bytes, offering developers flexible approaches to interpret and manipulate byte data accurately in scenarios where unsigned values are essential.
Converting bytes to unsigned bytes is crucial for accurately representing values from 0
to 255
instead of the default -128
to 127
in Java.
This reinterpretation is vital in scenarios like binary file handling and network protocols, ensuring precise data processing and preventing unintended errors when working with byte data.
In Java, the byte
data type is signed and ranges from -128
to 127
. But, there are situations where we need to interpret a byte
as an unsigned value
, especially when dealing with binary data or low-level operations.
When working with bytes in Java, it’s crucial to understand that the byte
type is signed, meaning it can represent both positive and negative values. However, in many scenarios, especially when dealing with binary data or network communication, we need to treat bytes as unsigned values to ensure accurate representation.
Convert Bytes to Unsigned Bytes Using Bitwise AND
With 0xff
The bitwise AND
operation with 0xFF
allows us to perform this conversion effectively. This method is widely used and is essential for tasks such as reading and writing binary files, processing image data, and various other low-level operations.
Bitwise AND
with 0xFF
is preferred for simplicity and efficiency. It directly extracts the last 8 bits, ensuring a clean conversion to unsigned bytes.
Other methods involve additional operations (String formatting or method calls) that can introduce unnecessary complexity and overhead, making the bitwise AND
approach more straightforward for byte-to-unsigned-byte conversion in Java.
Let’s dive into a complete working example that demonstrates how to convert bytes to unsigned bytes using the bitwise AND
with 0xFF
method:
public class ByteConversionExample {
public static int byteToUnsigned(byte b) {
return b & 0xFF;
}
public static void main(String[] args) {
byte signedByte = (byte) 0xAB; // Example signed byte (-85 in decimal)
int unsignedByte = byteToUnsigned(signedByte);
System.out.println("Signed Byte: " + signedByte);
System.out.println("Unsigned Byte: " + unsignedByte);
}
}
In the method definition, we create a static function called byteToUnsigned
, designed to convert a signed byte into an unsigned integer.
In the byteToUnsigned
method, the input signed byte b
undergoes a bitwise AND
operation with the hexadecimal value 0xFF
.
This operation retains the last 8 bits of the byte, effectively discarding the sign bit and treating the byte as an unsigned value. In the main
method, an example signed byte 0xAB
(representing -85
in decimal) is passed to the byteToUnsigned
method, resulting in the variable unsignedByte
holding the correct unsigned value, which is 171
in this case (0xAB
in hexadecimal).
Output:
Signed Byte: -85
Unsigned Byte: 171
The results, including the original signed byte and the transformed unsigned byte, are then printed to demonstrate the effectiveness of the conversion process.
Convert Bytes to Unsigned Bytes Using Integer.parseInt
With Radix16
Integer.parseInt
with radix 16
interprets bytes as hexadecimal strings, providing a straightforward conversion method. It’s advantageous when bytes need conversion to unsigned bytes in a string format.
The choice between methods depends on the specific data representation and conversion needs.
Let’s consider a byte with a value of -1
(represented as 0xFF
in hexadecimal) and see how this method aids in obtaining the correct unsigned representation.
public class ByteConversionExample {
public static int byteToUnsigned(byte b) {
return Integer.parseInt(String.format("%02X", b), 16);
}
public static void main(String[] args) {
byte signedByte = (byte) -1; // Example signed byte (-1 in decimal)
int unsignedByte = byteToUnsigned(signedByte);
System.out.println("Signed Byte: " + signedByte);
System.out.println("Unsigned Byte: " + unsignedByte);
}
}
In the method definition, we introduce a static method called byteToUnsigned
. This method takes a signed byte as input, converts it to a hexadecimal string using %02X
formatting, and then parses it into an integer with Radix 16
using Integer.parseInt
.
Moving on to the main method, we initialize a signed byte with a value of -1
. This specific byte, when interpreted as unsigned, should be represented as 255
in decimal (0xFF
in hexadecimal).
Concluding with the print results, we showcase the original signed byte and the converted unsigned byte, allowing us to observe the seamless transformation achieved through the specified method.
Output:
Signed Byte: -1
Unsigned Byte: 255
With the provided code, the output demonstrates the effectiveness of using Integer.parseInt
with Radix 16
to convert a signed byte, resulting in the correct representation of the unsigned byte, where -1
is successfully transformed into 255
.
Convert Bytes to Unsigned Bytes Using Byte.toUnsignedInt
Byte.toUnsignedInt
is preferred for simplicity and readability. It’s specifically designed for converting bytes to unsigned integers, offering a concise and direct method.
Byte.toUnsignedInt
is often favored for its clarity and purposefulness.
To illustrate the effectiveness of this method, let’s consider a byte with a value of -127
and see how Byte.toUnsignedInt
aids in obtaining the correct unsigned representation.
public class ByteConversionExample {
public static void main(String[] args) {
byte signedByte = (byte) -127; // Example signed byte (-127 in decimal)
int unsignedByte = Byte.toUnsignedInt(signedByte);
System.out.println("Signed Byte: " + signedByte);
System.out.println("Unsigned Byte: " + unsignedByte);
}
}
In the main method, a signed byte with a value of -127
is declared. When treated as unsigned, this byte should be represented as 129
in decimal.
This code utilizes the Byte.toUnsignedInt
method to convert a signed byte into an unsigned byte. The signedByte
variable, representing a byte with potential negative values, is passed as an argument to Byte.toUnsignedInt
.
This method specifically handles the conversion, treating the signed byte as an unsigned integer and providing the correct representation in the unsignedByte
variable. Essentially, Byte.toUnsignedInt
ensures that the byte’s value is interpreted as non-negative, producing an accurate unsigned byte representation.
The Byte.toUnsignedInt
method is then employed to convert this signed byte to its corresponding unsigned integer representation.
The results, including the original signed byte and the converted unsigned byte, are printed to facilitate observation of the seamless transformation achieved through the application of the Byte.toUnsignedInt
method.
Output:
Signed Byte: -127
Unsigned Byte: 129
This showcases the effectiveness of the Byte.toUnsignedInt
method in accurately handling the transformation and ensuring the proper interpretation of byte values in a Java program.
Conclusion
The bitwise AND
with 0xFF
method efficiently converts signed bytes to unsigned bytes, crucial for accurate processing of binary data in Java applications, whether in file I/O, network communication, or image processing.
Using Integer.parseInt
with Radix 16
offers a valuable approach to converting signed bytes into unsigned bytes, ensuring precision in handling binary or hexadecimal data in Java applications. This method enhances reliability in low-level operations involving byte manipulation.
The Byte.toUnsignedInt
method simplifies the conversion of signed bytes to their unsigned representation, providing a clean and readable solution.
Essential for low-level operations, understanding and applying the Byte.toUnsignedInt
technique ensures accuracy and precision when handling byte data in Java development. The method’s built-in nature enhances code readability and supports efficient development practices as Java evolves.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn