Salvar uma string em um arquivo em Java
-
Grave uma string em um arquivo usando a classe
PrintWriter
em Java -
Grave uma string em um arquivo usando a classe Java7
Files
-
Grave uma string em um arquivo usando a classe
FileWriter
em Java
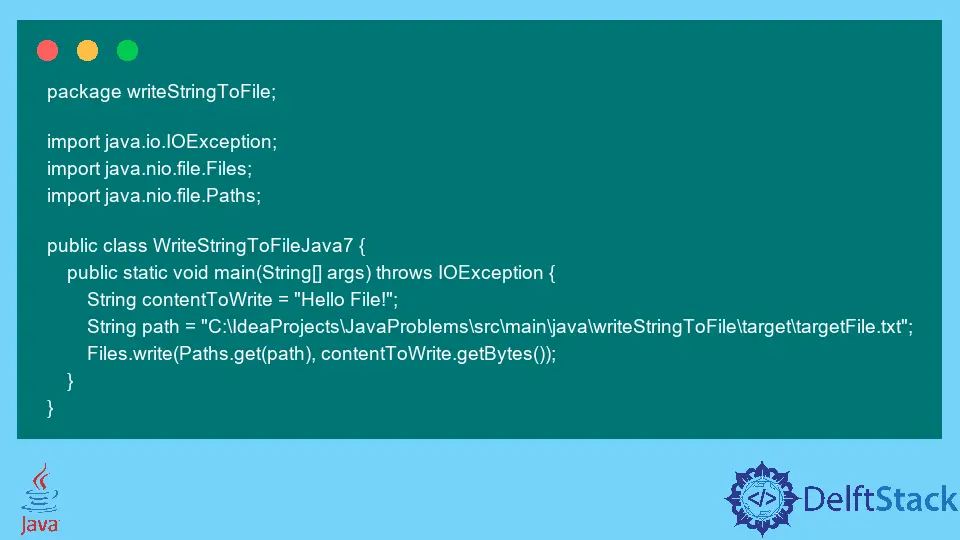
Este artigo apresentará métodos para gravar uma string em um arquivo em Java.
Grave uma string em um arquivo usando a classe PrintWriter
em Java
Para escrever uma string em um arquivo, podemos usar a classe PrintWriter
. O construtor da classe cria um arquivo com o nome especificado fornecido como parâmetro.
O construtor lança FileNotFoundException
se a string não existe, ou se não podemos criar o arquivo, ou se algum outro erro ocorre ao abrir ou criar o arquivo.
A função println()
imprimirá a string no arquivo e encerrará uma linha.
O método close()
fechará o fluxo e liberará os recursos do sistema associados a ele.
package writeStringToFile;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.Objects;
public class WriteStringToFile {
public static void main(String[] args) {
PrintWriter printWriter = null;
String textToBeWritten = "Hello";
{
try {
printWriter = new PrintWriter("writerFile.txt");
} catch (FileNotFoundException e) {
System.out.println("Unable to locate the fileName: " + e.getMessage());
}
Objects.requireNonNull(printWriter).println(textToBeWritten);
printWriter.close();
}
}
}
Grave uma string em um arquivo usando a classe Java7 Files
A classe Files
consiste exclusivamente em métodos estáticos que operam em arquivos, diretórios ou outros tipos de arquivos. O método write()
grava bytes em um arquivo. Os parâmetros de opções especificam como o arquivo é criado ou aberto. Se nenhuma opção estiver presente, este método funciona como se as opções CREATE
, TRUNCATE_EXISTING
e WRITE
já estivessem lá.
O método leva dois parâmetros, path
e bytes
.
O path
especifica o caminho do arquivo de destino. O método getBytes()
converte a string no formato Byte.
O método lança IOException
se ocorrer um erro durante a gravação ou criação de um arquivo.
package writeStringToFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteStringToFileJava7 {
public static void main(String[] args) throws IOException {
String contentToWrite = "Hello File!";
String path =
"C:\IdeaProjects\JavaProblems\src\main\java\writeStringToFile\target\targetFile.txt";
Files.write(Paths.get(path), contentToWrite.getBytes());
}
}
Grave uma string em um arquivo usando a classe FileWriter
em Java
A classe BufferedWriter
cria um fluxo de saída de caracteres em buffer que usa um buffer de saída de tamanho padrão. Leva qualquer objeto de gravação como parâmetro. O construtor da classe FileWriter
obtém o nome do arquivo, que é o destino para armazenar a string. O método write
grava o texto no arquivo associado no objeto. Este método lança IOException
quando o arquivo não é localizável.
Agora, no bloco finally
, deve-se liberar os recursos para as operações de entrada e saída. O método close
lança ainda mais a classe IOException
, então devemos usar a função close
no bloco try-catch ou adicionar a cláusula throws
no método pai.
package writeStringToFile;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class WriteToFileUsingFileWriter {
public static void main(String[] args) throws IOException {
BufferedWriter bufferedWriter = null;
try {
bufferedWriter = new BufferedWriter(new FileWriter("targetFile.txt"));
bufferedWriter.write("Hey! Content to write in File");
} catch (IOException e) {
System.out.println("Exception occurred: " + e.getMessage());
} finally {
if (bufferedWriter != null)
bufferedWriter.close();
}
}
}
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedInArtigo relacionado - Java String
- Como converter a string Java em Byte
- Como converter Byte Array em Hex String em Java
- Como realizar a conversão de string em array de string em Java
- Como remover string de string em Java
- Comparar Strings em Java
- Gerar String Aleatória em Java