在 Java 中將字串儲存到檔案
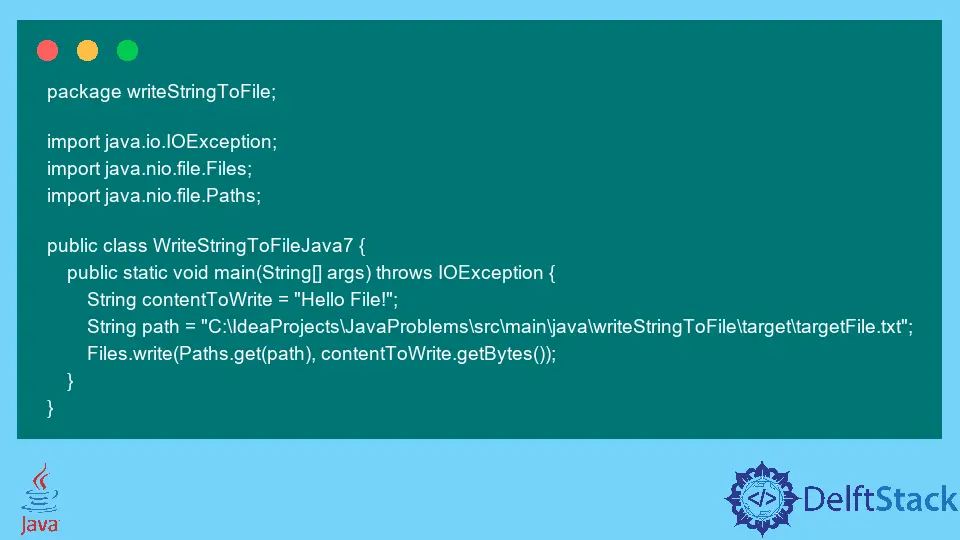
本文將介紹在 Java 中將字串寫入檔案的方法。
在 Java 中使用 PrintWriter
類將字串寫入到檔案中
要將字串寫入檔案,我們可以使用 PrintWriter
類。該類的建構函式建立一個具有指定名稱作為引數的檔案。
如果字串不存在,或者我們無法建立檔案,或者在開啟或建立檔案時發生其他錯誤,則建構函式將丟擲 FileNotFoundException
。
println()
函式將在檔案中列印字串並終止一行。
close()
方法將關閉流並釋放與之關聯的系統資源。
package writeStringToFile;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.Objects;
public class WriteStringToFile {
public static void main(String[] args) {
PrintWriter printWriter = null;
String textToBeWritten = "Hello";
{
try {
printWriter = new PrintWriter("writerFile.txt");
} catch (FileNotFoundException e) {
System.out.println("Unable to locate the fileName: " + e.getMessage());
}
Objects.requireNonNull(printWriter).println(textToBeWritten);
printWriter.close();
}
}
}
使用 Java7 的 Files
類將字串寫入檔案
Files
類僅包含對檔案,目錄或其他型別的檔案進行操作的靜態方法。write()
方法將位元組寫入檔案。選項引數指定如何建立或開啟檔案。如果不存在任何選項,則此方法的工作方式就像已經存在 CREATE
,TRUNCATE_EXISTING
和 WRITE
選項一樣。
該方法有兩個引數,path
和 byte
。
path
指定目標檔案的路徑。getBytes()
方法將字串轉換為 Byte
格式。
如果在寫入或建立檔案時發生錯誤,該方法將引發 IOException
。
package writeStringToFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteStringToFileJava7 {
public static void main(String[] args) throws IOException {
String contentToWrite = "Hello File!";
String path =
"C:\IdeaProjects\JavaProblems\src\main\java\writeStringToFile\target\targetFile.txt";
Files.write(Paths.get(path), contentToWrite.getBytes());
}
}
使用 Java 中的 FileWriter
類將字串寫入檔案
BufferedWriter
類建立一個使用預設大小的輸出緩衝區的緩衝區字元輸出流。它以任何 writer 物件為引數。FileWriter
類建構函式採用檔名,該檔名是儲存字串的目標。write
方法將文字寫入物件中的關聯檔案。當檔案不可放置時,此方法將引發 IOException
。
現在,在 finally
塊中,應該釋放用於輸入和輸出操作的資源。close
方法還會丟擲 IOException
類,因此我們應該在 try-catch 塊中使用 close
函式,或者在父方法中新增 throws
子句。
package writeStringToFile;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
public class WriteToFileUsingFileWriter {
public static void main(String[] args) throws IOException {
BufferedWriter bufferedWriter = null;
try {
bufferedWriter = new BufferedWriter(new FileWriter("targetFile.txt"));
bufferedWriter.write("Hey! Content to write in File");
} catch (IOException e) {
System.out.println("Exception occurred: " + e.getMessage());
} finally {
if (bufferedWriter != null)
bufferedWriter.close();
}
}
}
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn相關文章 - Java String
- 如何在 Java 中以十六進位制字串轉換位元組陣列
- 如何在 Java 中執行字串到字串陣列的轉換
- 如何將 Java 字串轉換為位元組
- 如何從 Java 中的字串中刪除子字串
- 用 Java 生成隨機字串
- Java 中的交換方法