在 Java 中將錯誤日誌寫入檔案
Sheeraz Gul
2024年2月15日
Java
Java File
Java Log
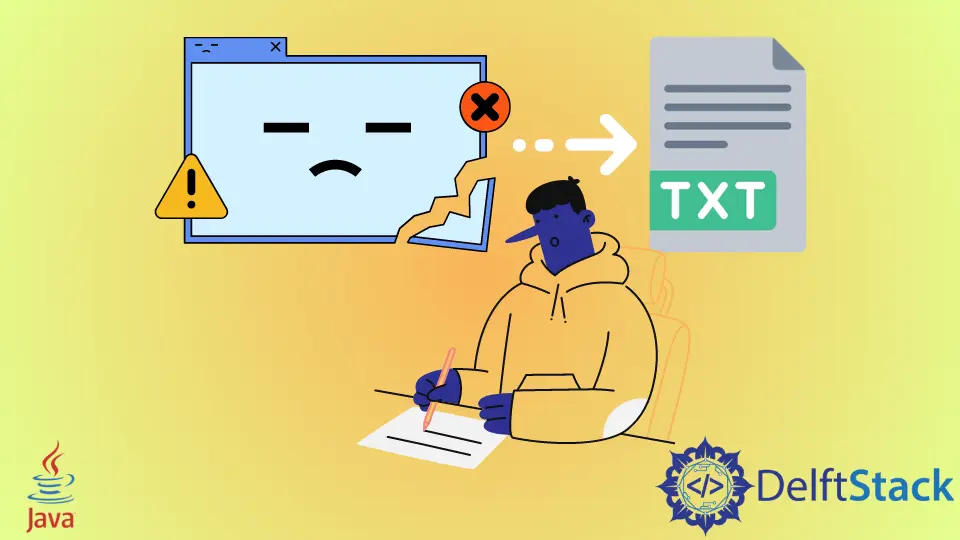
在 Java 中儲存錯誤日誌的最直接方法是將異常寫入檔案中。我們可以使用 try
和 catch
塊將錯誤寫入使用 FileWriter
、BufferedWriter
和 PrintWriter
的文字檔案。
本教程將演示如何在 Java 中儲存錯誤日誌。
在 Java 中使用 FileWriter
將錯誤日誌寫入檔案
FileWriter
、BufferedWriter
和 PrintWriter
是 Java 中用於建立和寫入檔案的內建功能。請參閱下面的示例,瞭解如何使用 Java 中的異常將錯誤寫入檔案。
package delftstack;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.PrintWriter;
public class Log_error {
public static void main(String[] args) {
try {
int[] Demo_Numbers = {1, 2, 3, 4, 5, 6, 7, 8};
System.out.println(Demo_Numbers[10]); // ArrayIndexOutOfBoundException
} catch (Exception e) {
appendToFile(e);
}
try {
Object reference = null;
reference.toString(); // NullPointerException
} catch (Exception e) {
appendToFile(e);
}
try {
int Number = 100 / 0; // ArithmeticException
} catch (Exception e) {
appendToFile(e);
}
try {
String demo = "abc";
int number = Integer.parseInt(demo); // NumberFormatException
} catch (Exception e) {
appendToFile(e);
}
}
public static void appendToFile(Exception e) {
try {
FileWriter New_File = new FileWriter("Error-log.txt", true);
BufferedWriter Buff_File = new BufferedWriter(New_File);
PrintWriter Print_File = new PrintWriter(Buff_File, true);
e.printStackTrace(Print_File);
} catch (Exception ie) {
throw new RuntimeException("Cannot write the Exception to file", ie);
}
}
}
上面的程式碼執行具有不同異常或錯誤的程式碼,然後將它們寫入文字檔案。
輸出:
java.lang.ArrayIndexOutOfBoundsException: Index 10 out of bounds for length 8
at delftstack.Log_error.main(Log_error.java:11)
java.lang.NullPointerException: Cannot invoke "Object.toString()" because "reference" is null
at delftstack.Log_error.main(Log_error.java:19)
java.lang.ArithmeticException: / by zero
at delftstack.Log_error.main(Log_error.java:25)
java.lang.NumberFormatException: For input string: "abc"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67)
at java.base/java.lang.Integer.parseInt(Integer.java:668)
at java.base/java.lang.Integer.parseInt(Integer.java:786)
at delftstack.Log_error.main(Log_error.java:32)
上面的輸出被寫入 Error-log.txt
檔案:
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook