在 Java 中将错误日志写入文件
Sheeraz Gul
2024年2月15日
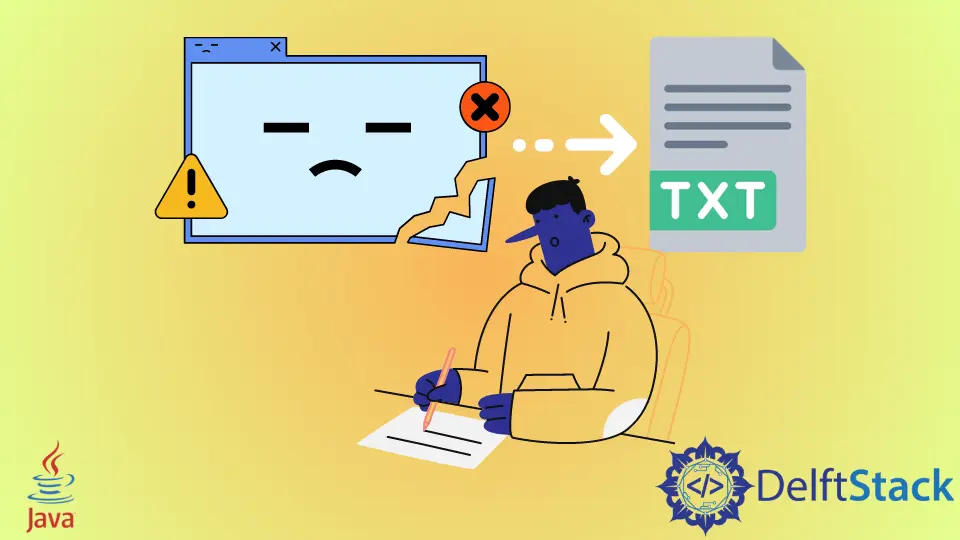
在 Java 中保存错误日志的最直接方法是将异常写入文件中。我们可以使用 try
和 catch
块将错误写入使用 FileWriter
、BufferedWriter
和 PrintWriter
的文本文件。
本教程将演示如何在 Java 中保存错误日志。
在 Java 中使用 FileWriter
将错误日志写入文件
FileWriter
、BufferedWriter
和 PrintWriter
是 Java 中用于创建和写入文件的内置功能。请参阅下面的示例,了解如何使用 Java 中的异常将错误写入文件。
package delftstack;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.PrintWriter;
public class Log_error {
public static void main(String[] args) {
try {
int[] Demo_Numbers = {1, 2, 3, 4, 5, 6, 7, 8};
System.out.println(Demo_Numbers[10]); // ArrayIndexOutOfBoundException
} catch (Exception e) {
appendToFile(e);
}
try {
Object reference = null;
reference.toString(); // NullPointerException
} catch (Exception e) {
appendToFile(e);
}
try {
int Number = 100 / 0; // ArithmeticException
} catch (Exception e) {
appendToFile(e);
}
try {
String demo = "abc";
int number = Integer.parseInt(demo); // NumberFormatException
} catch (Exception e) {
appendToFile(e);
}
}
public static void appendToFile(Exception e) {
try {
FileWriter New_File = new FileWriter("Error-log.txt", true);
BufferedWriter Buff_File = new BufferedWriter(New_File);
PrintWriter Print_File = new PrintWriter(Buff_File, true);
e.printStackTrace(Print_File);
} catch (Exception ie) {
throw new RuntimeException("Cannot write the Exception to file", ie);
}
}
}
上面的代码运行具有不同异常或错误的代码,然后将它们写入文本文件。
输出:
java.lang.ArrayIndexOutOfBoundsException: Index 10 out of bounds for length 8
at delftstack.Log_error.main(Log_error.java:11)
java.lang.NullPointerException: Cannot invoke "Object.toString()" because "reference" is null
at delftstack.Log_error.main(Log_error.java:19)
java.lang.ArithmeticException: / by zero
at delftstack.Log_error.main(Log_error.java:25)
java.lang.NumberFormatException: For input string: "abc"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67)
at java.base/java.lang.Integer.parseInt(Integer.java:668)
at java.base/java.lang.Integer.parseInt(Integer.java:786)
at delftstack.Log_error.main(Log_error.java:32)
上面的输出被写入 Error-log.txt
文件:
作者: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook