Java 中的文件路径
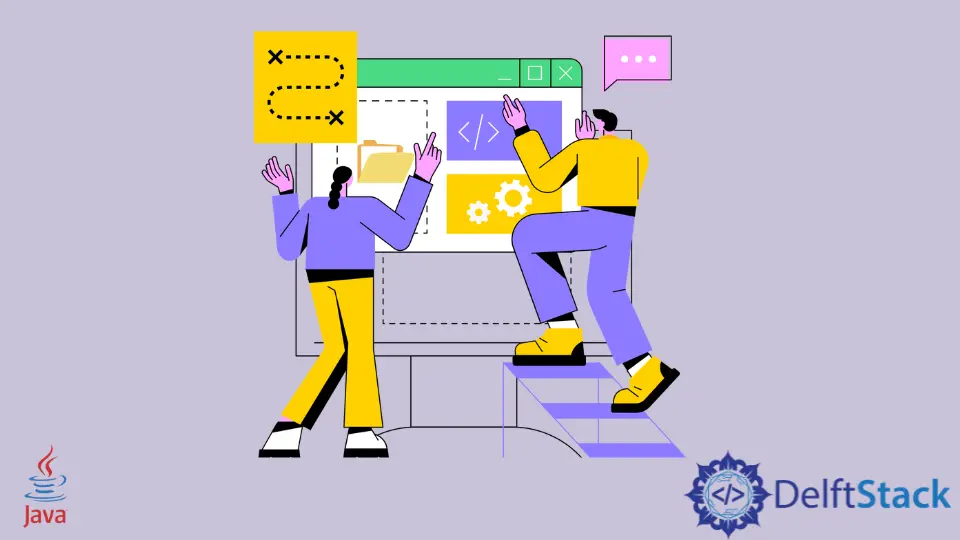
在 Java 中处理文件时,指定正确的文件名以及正确的路径和扩展名非常重要。
我们正在使用的文件可能存在于同一目录或另一个目录中。根据文件的位置,路径名会发生变化。
本文将讨论如何在 Java 中访问和指定文件路径。
读取 Java 文件
读取文件意味着获取文件的内容。我们使用 Java Scanner
类来读取文件。让我们通过一个例子来理解这一点。
我们将首先编写一个 Java 程序来创建一个新文件。然后我们将编写另一个 Java 程序来为这个新创建的文件添加一些内容。
最后,我们将读取这个文件的内容。请注意,本文中的所有程序都不会在在线编译器上运行(使用正确设置路径的离线编译器)。
创建文件
import java.io.File;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
// Try catch block
try {
// Creating a file with the name demofile.txt
File myFile = new File("demofile.txt");
if (myFile.createNewFile()) {
System.out.println("The file is created with the name: " + myFile.getName());
} else {
System.out.println("The file already exists.");
}
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
输出:
The file is created with the name: demofile.txt
该文件在 Java 文件所在的同一目录中创建。所有 Java 程序文件都在 C 目录中。
向文件中添加一些内容
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
// create an object
FileWriter writeInFile = new FileWriter("demofile.txt");
// Adding content to this file
writeInFile.write("We are learning about paths in Java.");
writeInFile.close();
System.out.println("Successfully done!");
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
输出:
Successfully done!
读取文件
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner; //scanner class for reading the file
public class Main {
public static void main(String[] args) {
try {
File myFile = new File("demofile.txt");
// create the scanner object
Scanner readFile = new Scanner(myFile);
while (readFile.hasNextLine()) {
String data = readFile.nextLine();
System.out.println(data);
}
readFile.close();
} catch (FileNotFoundException x) {
System.out.println("An error occurred.");
x.printStackTrace();
}
}
}
输出:
We are learning about paths in Java.
请注意,在这里,在 File()
方法中,我们只传递文件名及其扩展名。
我们没有指定 demofile.txt
的完整路径。demofile.txt
存在于我们保存所有这些 Java 程序文件的同一目录和文件夹中。
但是,如果 demofile.txt
存在于另一个目录或文件夹中,则读取该文件并不是那么简单。在这种情况下,我们指定文件的完整路径。
要获取有关 Java 文件系统的更多详细信息,请参阅此文档。
在 Java 中如何指定文件路径
为了指定文件的路径,我们在 File()
方法中传递文件名及其扩展名。我们这样做。
new File("demofile.txt")
请注意,仅当文件与工作项目的目录位于同一文件夹中时,文件名才足够。否则,我们必须指定文件的完整路径。
如果你不知道文件名,请使用 list()
方法。此方法返回当前目录中所有文件的列表。
在 java.io
库的 File
类中,list()
方法以数组的形式返回所有文件和目录的列表。它返回的输出基于抽象路径名定义的当前目录。
如果抽象路径名不表示目录,则 list()
方法返回 null
。有时,我们不知道文件的位置。
此外,当我们必须读取的文件不在当前工作目录中时,就会发生错误。使用文件的完整路径可以更好地避免这些问题。
要获取文件的确切路径,请使用下面给出的方法。
在 Java 中如何获取文件路径
当我们不知道文件的路径时,可以使用 Java 的一些方法来查找文件的路径。然后,我们可以将此路径名指定为参数。
Java 提供三种类型的文件路径 - absolute
、canonical
和 abstract
。java.io.file
类具有三种查找文件路径的方法。
在 Java 中使用 getPath()
方法获取文件路径
getPath()
方法属于 Java 的 File 类。它以字符串形式返回抽象文件路径。
抽象路径名是 java.io.file
的对象,它引用磁盘上的文件。
语法:
file.getPath()
例子:
import java.io.*;
public class Main {
public static void main(String args[]) {
try {
// create an object
File myFile = new File("demo.txt");
// call the getPath() method
String path = myFile.getPath();
System.out.println("The path of this file is: " + path);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
输出:
The path of this file is: demo.txt
正如你在上面的输出中看到的,只有带有扩展名的文件名是输出。这表明该文件存在于 Java 程序文件所在的同一文件夹中。
在 Java 中使用 getAbsolutePath()
方法获取文件路径
getAbsolutePath()
方法返回一个字符串作为文件的绝对路径。如果我们使用绝对路径名创建文件,那么 getAbsolutePath()
方法会返回路径名。
但是,如果我们使用相对路径创建对象,getAbsolutePath()
方法会根据系统解析路径名。它存在于 Java 的 File 类中。
语法:
file.getAbsolutePath()
请注意,绝对路径是给出系统中存在的文件的完整 URL 的路径,与它所在的目录无关。另一方面,相对路径给出文件到当前目录的路径。
例子:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("pathdemo.txt");
// call the getAbsolutePath() method
String absolutePath = myFile.getAbsolutePath();
System.out.println("The Absolute path of the file is: " + absolutePath);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
输出:
The Absolute path of the file is: C:\Users\PC\pathdemo.txt
请注意,这次我们获得了完整的工作路径,从当前工作目录开始到文件所在的当前文件夹。
因此,当你不知道任何文件的位置时,请使用绝对路径方法来查找文件所在的位置。然后,你可以在必须读取该文件时指定该路径。
这样,很容易找到要读取的文件。
在 Java 中使用 getCanonicalPath()
方法获取文件路径
Path
类中的存在返回文件的规范路径。如果路径名是规范的,则 getCanonicalPath()
方法返回文件的路径。
规范路径始终是唯一且绝对的。此外,此方法删除任何。
或路径中的 ..
。
语法:
file.getCanonicalPath()
例子:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("C:\\Users");
// call the getCanonicalPath() method
String canonical = myFile.getCanonicalPath();
System.out.println("The Canonical path is : " + canonical);
} catch (Exception x) {
System.err.println(x.getMessage());
}
}
}
输出:
The Canonical path is:C:\Users
要阅读有关 Java 中路径的更多信息,请参阅此文档。
结论
在本文中,我们了解了如何在必须读取文件时指定文件的路径。我们还研究了 Java 提供的各种方法,如 getPath()
、getAbsolutePath()
和 getCanonical()
路径来获取文件的路径。
此外,我们看到了路径如何根据文件和当前项目的目录和文件夹而变化。我们还创建并读取了一些文件作为演示。
但是,这些程序不会在在线编译器上运行。