File Path in Java
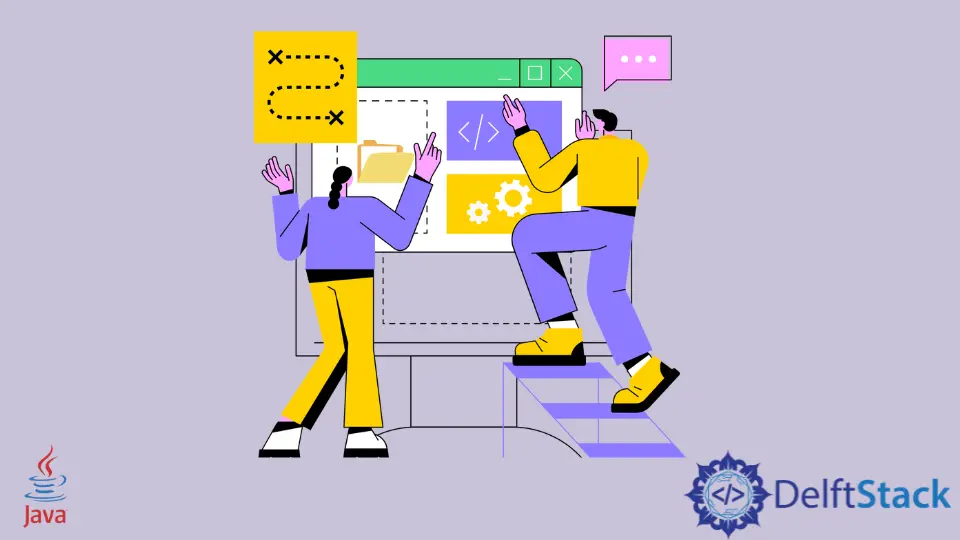
While working with files in Java, it is important to specify the correct file name and the correct path and extension.
The files we are working with might be present in the same or another directory. Depending on the location of the file, the pathname changes.
This article will discuss how to access and specify file paths in Java.
Reading a Java File
Reading a file means getting the content of a file. We use the Java Scanner
class to read a file. Let us understand this through an example.
We will first write a Java program to create a new file. Then we will write another Java program to add some content to this newly created file.
Finally, we will read the contents of this file. Note that none of the programs in this article will run on an online compiler (use an offline compiler with the path correctly set).
Create a File
import java.io.File;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
// Try catch block
try {
// Creating a file with the name demofile.txt
File myFile = new File("demofile.txt");
if (myFile.createNewFile()) {
System.out.println("The file is created with the name: " + myFile.getName());
} else {
System.out.println("The file already exists.");
}
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
Output:
The file is created with the name: demofile.txt
This file gets created in the same directory where the Java files are present. All the Java program files are in the C directory.
Add Some Content to the File
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
// create an object
FileWriter writeInFile = new FileWriter("demofile.txt");
// Adding content to this file
writeInFile.write("We are learning about paths in Java.");
writeInFile.close();
System.out.println("Successfully done!");
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
Output:
Successfully done!
Read the File
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner; //scanner class for reading the file
public class Main {
public static void main(String[] args) {
try {
File myFile = new File("demofile.txt");
// create the scanner object
Scanner readFile = new Scanner(myFile);
while (readFile.hasNextLine()) {
String data = readFile.nextLine();
System.out.println(data);
}
readFile.close();
} catch (FileNotFoundException x) {
System.out.println("An error occurred.");
x.printStackTrace();
}
}
}
Output:
We are learning about paths in Java.
Note that here, inside the File()
method, we only pass the file’s name with its extension.
We are not specifying the complete path of the demofile.txt
. The demofile.txt
is present in the same directory and folder where we save all these Java program files.
However, if the demofile.txt
is present in another directory or folder, reading this file is not that simple. In such cases, we specify the complete path of the file.
To get more details about the file system in Java, refer to this documentation.
How to Specify File Path in Java
To specify the path of a file, we pass the file’s name with its extension inside the File()
method. We do it like this.
new File("demofile.txt")
Note that the file name is sufficient only when the file is in the same folder as the working project’s directory. Otherwise, we have to specify the complete path of the file.
If you don’t know the file’s name, use the list()
method. This method returns the list of all the files in the current directory.
Present in the File
class of the java.io
library, the list()
method returns the list of all the files and directories as an array. The output it returns is based on the current directory defined by an abstract pathname.
If the abstract pathname does not denote a directory, the list()
method returns null
. Sometimes, we don’t know where the file is located.
Moreover, errors occur when the file we have to read is not present in the current working directory. Using the file’s complete path is better to avoid these issues.
To get the exact path of a file, use the methods given below.
How to Get File Path in Java
When we don’t know the path of a file, we can use some methods of Java to find the path of a file. Then, we can specify this pathname as an argument.
Java provides three types of file paths - absolute
, canonical
, and abstract
. The java.io.file
class has three methods to find the path of a file.
Get File Path Using getPath()
Method in Java
The getPath()
method belongs to the File class of Java. It returns the abstract file path as a string.
An abstract pathname is an object of java.io.file
, which references a file on the disk.
Syntax:
file.getPath()
Example:
import java.io.*;
public class Main {
public static void main(String args[]) {
try {
// create an object
File myFile = new File("demo.txt");
// call the getPath() method
String path = myFile.getPath();
System.out.println("The path of this file is: " + path);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
Output:
The path of this file is: demo.txt
As you can see in the output above, only the file’s name with the extension is the output. This shows that the file is present in the same folder where the Java program file is.
Get File Path Using getAbsolutePath()
Method in Java
The getAbsolutePath()
method returns a string as the file’s absolute path. If we create the file with an absolute pathname, then the getAbsolutePath()
method returns the pathname.
However, if we create the object using a relative path, the getAbsolutePath()
method resolves the pathname depending on the system. It is present in the File class of Java.
Syntax:
file.getAbsolutePath()
Note that the absolute path is the path that gives the complete URL of the file present in the system irrespective of the directory that it is in. On the other hand, the relative path gives the file’s path to the present directory.
Example:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("pathdemo.txt");
// call the getAbsolutePath() method
String absolutePath = myFile.getAbsolutePath();
System.out.println("The Absolute path of the file is: " + absolutePath);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
Output:
The Absolute path of the file is: C:\Users\PC\pathdemo.txt
Note that we get the complete working path this time, starting from the current working directory to the current folder in which the file is present.
So, whenever you don’t know the location of any file, use the absolute path method to find where the file is present. Then, you can specify that path whenever you have to read that file.
This way, the file to be read would be easily found.
Get the File Path Using getCanonicalPath()
Method in Java
Present in the Path
class returns the canonical path of a file. If the pathname is canonical, then the getCanonicalPath()
method returns the file’s path.
The canonical path is always unique and absolute. Moreover, this method removes any .
or ..
from the path.
Syntax:
file.getCanonicalPath()
Example:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("C:\\Users");
// call the getCanonicalPath() method
String canonical = myFile.getCanonicalPath();
System.out.println("The Canonical path is : " + canonical);
} catch (Exception x) {
System.err.println(x.getMessage());
}
}
}
Output:
The Canonical path is:C:\Users
To read more about paths in Java, refer to this documentation.
Conclusion
In this article, we saw how we could specify the path of a file if we have to read it. We also studied the various methods like getPath()
, getAbsolutePath()
, and getCanonical()
path that Java provides to get the path of a file.
Moreover, we saw how the path changes depending on the directory and folder of the file and current project. We also created and read some files as a demonstration.
These programs, however, will not run on online compilers.
Related Article - Java File
- How to Remove Line Breaks Form a File in Java
- FileFilter in Java
- File Separator in Java
- How to Get File Size in Java
- How to Read Bytes From a File in Java
- How to Delete Files in a Directory Using Java