Relative Path in Java
- Define a Relative Path to Locate File in Java
- Define Relative Path for Parent Directory in Java
- Define Relative Path in Current Directory in Java
-
Define Relative Path Using the
../../
Prefix in Java
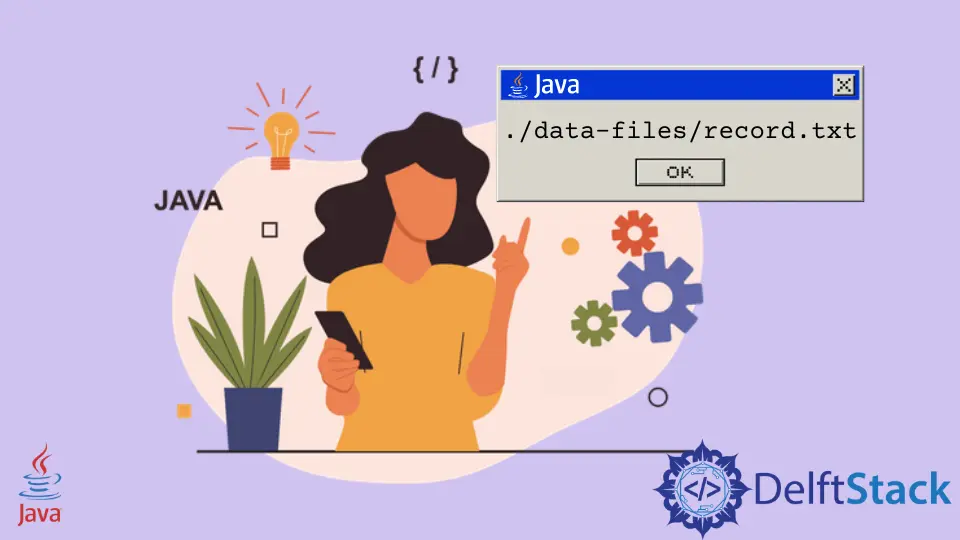
This tutorial introduces how to define a relative path in Java.
A relative path is an incomplete path (absence of root directory) and combined with the current directory path to access the resource file. The relative path doesn’t start with the root element of the file system.
We use the relative path to locate a file in the current directory or parent directory, or the same hierarchy.
There are several ways to define a relative path, such as ./
to refer to current directory path, ../
to immediate parent directory path, etc. Let’s see some examples.
Define a Relative Path to Locate File in Java
We can use the relative path to locate a file resource in the current working directory. See the example below.
import java.io.File;
public class SimpleTesting {
public static void main(String[] args) {
String filePath = "files/record.txt";
File file = new File(filePath);
String path = file.getPath();
System.out.println(path);
}
}
Output:
files/record.txt
Define Relative Path for Parent Directory in Java
We can use the ../
prefix with the file path to locate a file in the parent directory. This is the relative path for accessing a file in the parent directory. See the example below.
import java.io.File;
public class SimpleTesting {
public static void main(String[] args) {
String filePath = "../files/record.txt";
File file = new File(filePath);
String path = file.getPath();
System.out.println(path);
}
}
Output:
../files/record.txt
Define Relative Path in Current Directory in Java
If the file resource is located in the current directory, we can use the ./
prefix with the path to create a relative file path. See the example below.
import java.io.File;
public class SimpleTesting {
public static void main(String[] args) {
String filePath = "./data-files/record.txt";
File file = new File(filePath);
String path = file.getPath();
System.out.println(path);
}
}
Output:
./data-files/record.txt
Define Relative Path Using the ../../
Prefix in Java
If the file is located in two levels upper in the directory structure, use the ../../
prefix with the file path. See the below example.
import java.io.File;
public class SimpleTesting {
public static void main(String[] args) {
String filePath = "../../data-files/record.txt";
File file = new File(filePath);
String path = file.getPath();
System.out.println(path);
String absPath = file.getAbsolutePath();
System.out.println(absPath);
}
}
Output:
../../data-files/record.txt