How to Convert Inputstream to Byte Array in Java
-
Convert InputStream to Byte Array Using the
readAllBytes()
Method in Java -
Convert InputStream to Byte Array Using the
toByteArray()
Method in Java -
Convert InputStream to Byte Array Using the
write()
Method in Java -
Convert InputStream to Byte Array Using the
readAllBytes()
Method in Java -
Convert InputStream to Byte Array Using the
readFully()
Method in Java -
Convert InputStream to Byte Array Using the
getBytes()
Method in Java - Convert InputStream to Byte Array Using the Customized Code in Java
-
Convert InputStream to Byte Array Using the
toByteArray()
Method in Java
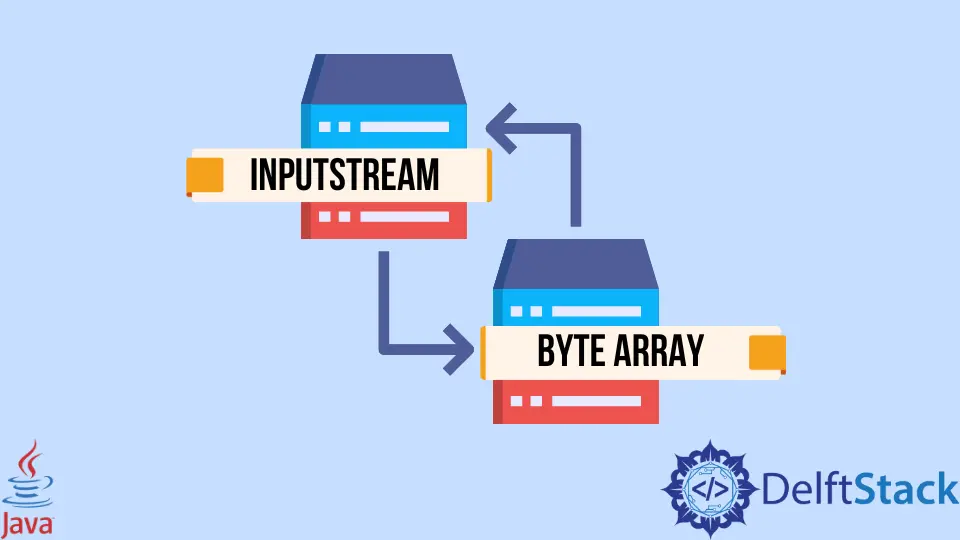
This tutorial introduces how to convert inputstream to byte array in Java and lists some example codes to understand the topic.
InputStream
is an abstract class and superclass of all the classes that represent an input stream of bytes. Java uses an input stream to read data from any source like file, array, etc. We will then see how to convert this stream to byte array by using some built-in methods and custom code in Java.
In this article, we will use several built-in methods such as toByteArray()
, readAllBytes()
, readFully()
, getBytes()
, write()
, etc and a text file abc.txt
is used to read data. This text file contains a single sentence, Welcome to Delfstack
, that we will be read and converted into a byte array, which further can be converted into a string to check the desired result.
Convert InputStream to Byte Array Using the readAllBytes()
Method in Java
We can use the readAllBytes()
method to get all the data into a byte array. This method returns a byte array that can be passed further into the String
constructor to print textual data.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
InputStream ins = new FileInputStream("abc.txt");
byte[] byteArray = ins.readAllBytes();
System.out.println(new String(byteArray));
ins.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delfstack
Convert InputStream to Byte Array Using the toByteArray()
Method in Java
If you work the Apache library, you can use the toByteArray()
method of IOUtils
class to get all the data into the byte array. This method returns a byte array that can be passed further into the String
constructor to print the textual data.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import org.apache.commons.io.IOUtils;
public class SimpleTesting {
public static void main(String[] args) {
try {
InputStream ins = new FileInputStream("abc.txt");
byte[] bytes = IOUtils.toByteArray(ins);
System.out.println(new String(bytes));
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delftstack
Convert InputStream to Byte Array Using the write()
Method in Java
We can use the write()
method of the ByteArrayOutputStream
class to get all the data into a byte array. This method returns a byte array that can be passed into String
constructor to print textual data.
package myjavaproject;
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
InputStream ins = new FileInputStream("abc.txt");
ByteArrayOutputStream buffer = new ByteArrayOutputStream();
int read;
byte[] data = new byte[16384];
while ((read = ins.read(data, 0, data.length)) != -1) {
buffer.write(data, 0, read);
}
System.out.println(new String(data));
ins.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delfstack
Convert InputStream to Byte Array Using the readAllBytes()
Method in Java
We can use the readAllBytes()
method of the DataInputStream
class to convert all the data into a byte array. This method returns a byte array that can be passed further into the String
constructor to print textual data.
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class SimpleTesting {
public static void main(String[] args) {
try {
String file = "abc.txt";
DataInputStream dis = new DataInputStream(new FileInputStream(file));
byte[] bytes = dis.readAllBytes();
System.out.println(new String(bytes));
dis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delftstack
Convert InputStream to Byte Array Using the readFully()
Method in Java
We can use the readFully()
method of the RandomAccessFile
class to convert all the data into a byte array. This method returns a byte array, which can be passed into the String
constructor to print textual data.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
public class SimpleTesting {
public static void main(String[] args) {
try {
String file = "abc.txt";
InputStream is = new FileInputStream(file);
RandomAccessFile raf = new RandomAccessFile(file, "r");
byte[] bytesData = new byte[(int) raf.length()];
raf.readFully(bytesData);
System.out.println(new String(bytesData));
is.close();
raf.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delfstack
Convert InputStream to Byte Array Using the getBytes()
Method in Java
We can use the getBytes()
method of the String
class to convert all the data into a byte array. This method returns a byte array that can be passed further into the String
constructor to print textual data.
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.stream.Collectors;
public class SimpleTesting {
public static void main(String[] args) {
try {
String file = "abc.txt";
byte[] bytesData = {};
InputStream is = new FileInputStream(file);
try (BufferedReader buffer = new BufferedReader(new InputStreamReader(is))) {
bytesData = buffer.lines().collect(Collectors.joining("\n")).getBytes();
}
System.out.println(new String(bytesData));
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delftstack
Convert InputStream to Byte Array Using the Customized Code in Java
If you don’t want to use any built-in method, use this customized code to convert inputstream into a byte array. This code returns a byte array that can be passed further into the String
constructor to print textual data.
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
String file = "abc.txt";
byte[] bytesData = {};
bytesData = getBytesFromFile(new File(file));
System.out.println(new String(bytesData));
} catch (IOException e) {
e.printStackTrace();
}
}
public static byte[] getBytesFromFile(File file) throws IOException {
InputStream is = new FileInputStream(file);
long length = file.length();
byte[] bytes = new byte[(int) length];
int offset = 0;
int numRead = 0;
while (
offset < bytes.length && (numRead = is.read(bytes, offset, bytes.length - offset)) >= 0) {
offset += numRead;
}
if (offset < bytes.length) {
throw new IOException("Could not read file " + file.getName());
}
return bytes;
}
}
Output:
Welcome to Delfstack
Convert InputStream to Byte Array Using the toByteArray()
Method in Java
We can use toByteArray()
method of ByteArrayOutputStream
class to convert all the data into a byte array. This method returns a byte array that can be passed further into the String
constructor to print textual data.
import java.io.ByteArrayOutputStream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class SimpleTesting {
public static void main(String[] args) {
try {
String file = "abc.txt";
InputStream is = new FileInputStream(file);
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
is.transferTo(byteArrayOutputStream);
byte[] bytesData = byteArrayOutputStream.toByteArray();
System.out.println(new String(bytesData));
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
Welcome to Delfstack