How to Read All Files of a Folder in Java
- How to Read All the Files of a Folder in Java
-
Read All Files of a Folder Using
Files
Class in Java -
Read All Files From a Folder Using
newDirectoryStream()
Method in Java -
Read All Files of a Folder Using the
walkFileTree()
Method in Java
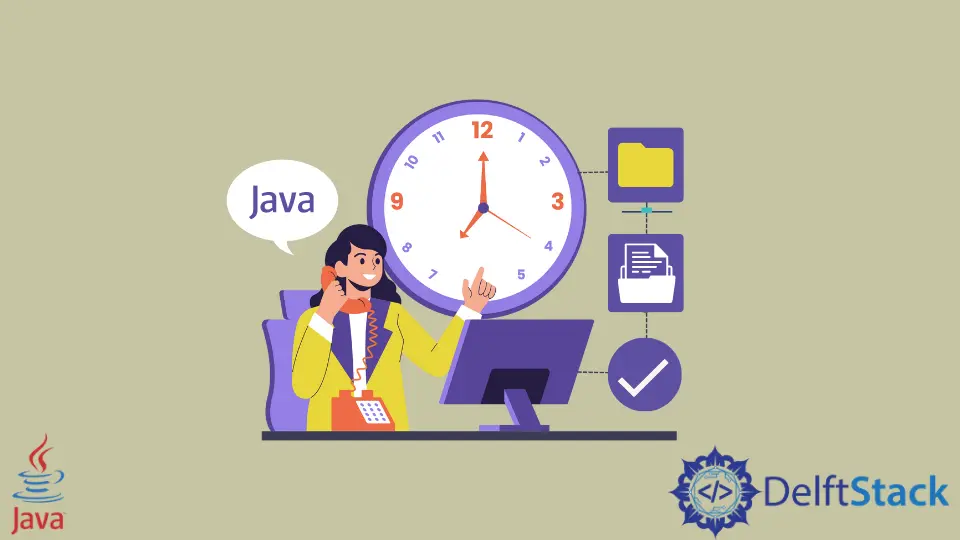
This tutorial introduces how to read all the files of a folder in Java and lists some example codes to understand it.
There are several ways to get all the files of a folder. Here we could use File
, Files
and DirectoryStream
classes, and many more. Let’s see the examples.
How to Read All the Files of a Folder in Java
Here, we use the File
class to collect all the files and folder in the source directory and then use the isDirectory()
method to check whether it is a file or folder. See the example below.
import java.io.File;
import java.text.ParseException;
public class SimpleTesting {
public static void findAllFilesInFolder(File folder) {
for (File file : folder.listFiles()) {
if (!file.isDirectory()) {
System.out.println(file.getName());
} else {
findAllFilesInFolder(file);
}
}
}
public static void main(String[] args) throws ParseException {
File folder = new File("/home/folder/src");
findAllFilesInFolder(folder);
}
}
Read All Files of a Folder Using Files
Class in Java
If you want to use stream, use the walk()
method of Files
class that returns a Path’s stream
. After that, we use the filter()
method to collect files only and use forEach()
to print them.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
try (Stream<Path> paths = Files.walk(Paths.get("/home/folder/src"))) {
paths.filter(Files::isRegularFile).forEach(System.out::println);
}
}
}
Read All Files From a Folder Using newDirectoryStream()
Method in Java
Here, we use the Files
class and its newDirectoryStream()
method that returns a stream of Path
. After that, we use a for-each
loop to iterate the list of files and print the file name.
import java.io.IOException;
import java.nio.file.DirectoryIteratorException;
import java.nio.file.DirectoryStream;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
try (DirectoryStream<Path> stream = Files.newDirectoryStream(Paths.get("/home/folder/src/"))) {
for (Path file : stream) {
System.out.println(file.getFileName());
}
} catch (IOException | DirectoryIteratorException ex) {
System.err.println(ex);
}
}
}
Read All Files of a Folder Using the walkFileTree()
Method in Java
Here, we use the walkFileTree()
method of the Files
class that takes two arguments: the source folder and the SimpleFileVisitor
reference. See the example below.
import java.io.IOException;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.attribute.BasicFileAttributes;
public class SimpleTesting {
public static void main(String[] args) throws IOException {
SimpleFileVisitor<Path> file = new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path filePath, BasicFileAttributes attrs)
throws IOException {
System.out.println(filePath);
return FileVisitResult.CONTINUE;
}
};
Files.walkFileTree(Paths.get("/home/folder/src"), file);
}
}
Related Article - Java File
- How to Remove Line Breaks Form a File in Java
- FileFilter in Java
- File Separator in Java
- How to Get File Size in Java
- How to Read Bytes From a File in Java
- How to Delete Files in a Directory Using Java