How to Append Text to a Text File in Java
-
Append Text to a Text File Using the
FileOutputStream
Class in Java -
Append Text to a Text File Using the
FileWriter
Class in Java -
Append Text to a Text File Using the
BufferedWriter
Class in Java -
Append Text to a Text File Using the
Files
Class in Java - Append Text to a Text File in Java
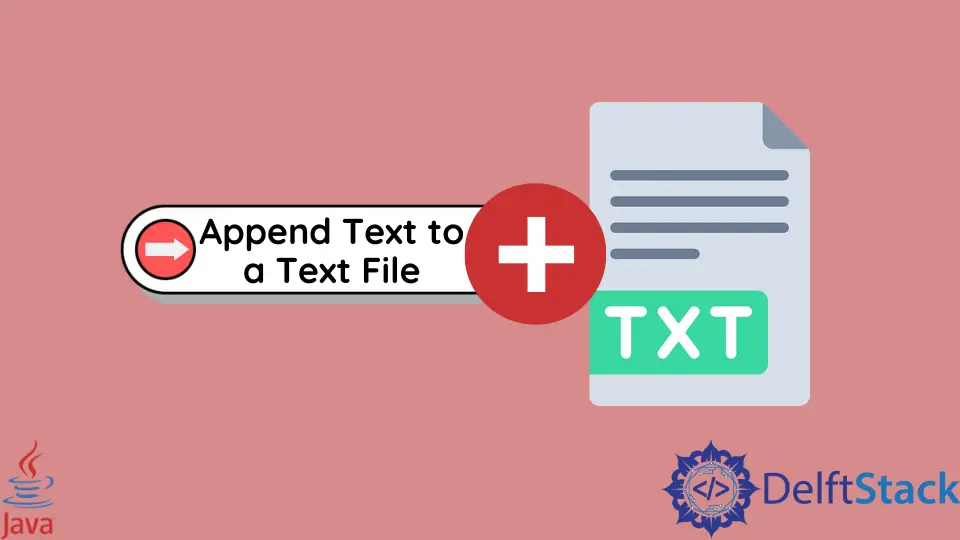
This tutorial introduces how to append text to an existing text file in Java. We’ve also listed some example codes to guide you better.
Reading and writing are two of the most basic operations you can do when working with files. There are several ways of writing or appending data to a file in Java. In this article, we’ll learn about the four different ways to append to a text file.
Append Text to a Text File Using the FileOutputStream
Class in Java
The FileOutputStream
class is used to write binary data to files. It is mostly preferred for primitive data types like int
or float
, but it can also be used for writing character-oriented data.
The string first needs to be converted to an array of bytes before writing; you can use the getBytes()
method for this purpose. We also need to pass true
as a second argument to its constructor. This second argument is for the Boolean append flag, and it’s done to indicate that we’re trying to append to an existing file.
If it is ignored, then the FileOutputStream
class will overwrite the contents of the file. It will create a new file if the file is not already present at the mentioned location.
Here is an example where we try to append the sentence The quick brown fox jumps over the lazy dog
to an existing file. The \r
and \n
are used to insert the text at the beginning of a new line.
import java.io.FileOutputStream;
public class Main {
public static void main(String args[]) {
try {
String filePath = "C:\\Users\\Lenovo\\Desktop\\demo.txt";
FileOutputStream f = new FileOutputStream(filePath, true);
String lineToAppend = "\r\nThe quick brown fox jumps over the lazy dog";
byte[] byteArr = lineToAppend.getBytes(); // converting string into byte array
f.write(byteArr);
f.close();
} catch (Exception e) {
System.out.println(e);
}
}
}
Append Text to a Text File Using the FileWriter
Class in Java
The Java FileWriter
class is designed to write character streams to a file. We can use it to append characters or strings to an existing file or a new file. If the file is not present at the mentioned path, then a new file is created, and data is written to this new file.
Unlike the previous FileOutputStream
class, we don’t need to convert the strings into bytes before writing. However, we also need to pass true
as a second argument to the FileWriter
constructor, which indicates that we are trying to append to an existing file.
If omitted, it will overwrite the existing content of the file. The following code demonstrates how we can append a single line to an existing file using FileWriter
.
import java.io.FileWriter;
public class SimpleTesting {
public static void main(String args[]) {
try {
String filePath = "C:\\Users\\Lenovo\\Desktop\\demo.txt";
FileWriter fw = new FileWriter(filePath, true);
String lineToAppend = "\r\nThe quick brown fox jumps over the lazy dog";
fw.write(lineToAppend);
fw.close();
} catch (Exception e) {
System.out.println(e);
}
}
}
Append Text to a Text File Using the BufferedWriter
Class in Java
The FileWriter
class is often wrapped by other writers like the BufferedWriter
. This process is done because a BufferedWriter
is much more efficient than a FileWriter
.
The BufferedWriter
is preferred over the FileWriter
when we have multiple write operations. It uses an internal buffer memory to store the data that has to be appended to the file. When this buffer is full, or we no longer have anything else to append, the BufferedWriter
will write to the actual file.
It writes large chunks of data to the file from its buffer instead of frequently writing to it; his leads to lesser system calls. Therefore, it is much more efficient than a normal FileWriter
.
But if we have a single write operation, then the BufferedWriter
adds another step in the writing process. So, it would be better just to use the FileWriter
.
To use the BufferedWriter
, we first need a FileWriter
object which we will pass to the constructor of the BufferedWriter
. Make sure to use true
for the append flag.
The following code demonstrates the working of BufferedWriter
in Java.
import java.io.BufferedWriter;
import java.io.FileWriter;
public class Main {
public static void main(String args[]) {
try {
String filePath = "C:\\Users\\Lenovo\\Desktop\\demo.txt";
FileWriter fw = new FileWriter(filePath, true);
BufferedWriter bw = new BufferedWriter(fw);
String lineToAppend = "\r\nThe quick brown fox jumps over the lazy dog";
bw.write(lineToAppend);
bw.close();
} catch (Exception e) {
System.out.println(e);
}
}
}
Append Text to a Text File Using the Files
Class in Java
The Files
utility class was added in Java 7, and it provides another way of writing data to files; we use the Files.write()
method to do this. This method requires the file path and also needs the byte array of the string to be appended.
We also need to specify the StandardOpenOption.APPEND
to append text to an existing file. Ensure that the file is present at the given path; otherwise, it will return a NoSuchFileException
.
This method is not preferred if we have to write to the same file multiple times because the file will also be opened and closed several times, decreasing the overall performance.
The following code below demonstrates how the Files.write()
method works in Java I/O.
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class Main {
public static void main(String args[]) {
try {
String file = "C:\\Users\\Lenovo\\Desktop\\demo.txt";
String lineToAppend = "\r\nThe quick brown fox jumps over the lazy dog";
byte[] byteArr = lineToAppend.getBytes();
Files.write(Paths.get(file), byteArr, StandardOpenOption.APPEND);
} catch (Exception e) {
System.out.println(e);
}
}
}
Append Text to a Text File in Java
Java provides multiple options for file handling. We can append data to a file by using FileOutputStream
,FileWriter
, BufferedWriter
, or the Files
utility class.
For the FileOutputStream
and Files
utility classes, we first need to convert the string to a bytes array and then pass it to the write()
method.
The BufferedWriter
is preferred when we have to write a small amount of data multiple times to the same file. On the other hand, the FileWriter
and Files
utility classes will make multiple system calls for a large number of writes; this decreases their overall performance.
Related Article - Java File
- How to Remove Line Breaks Form a File in Java
- FileFilter in Java
- File Separator in Java
- How to Get File Size in Java
- How to Read Bytes From a File in Java
- How to Delete Files in a Directory Using Java