Java 中的檔案路徑
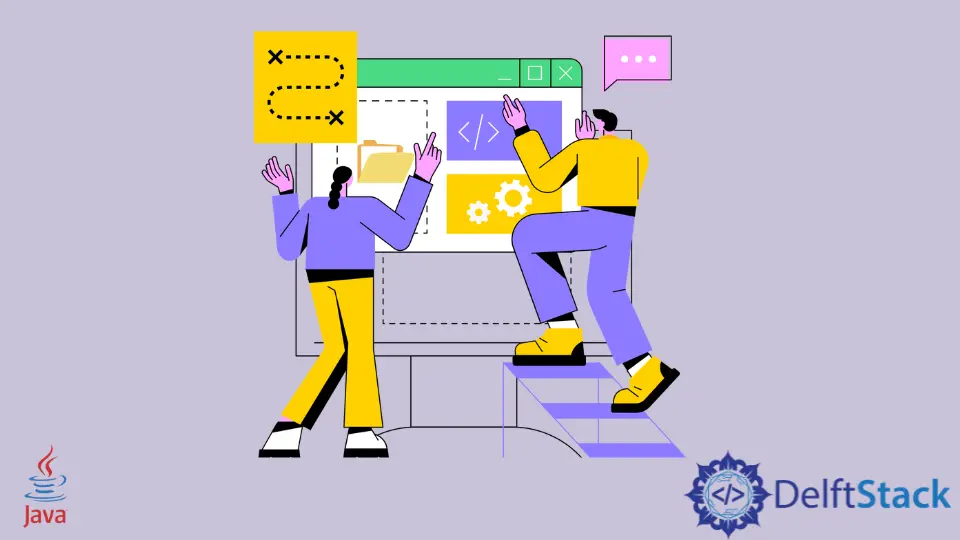
在 Java 中處理檔案時,指定正確的檔名以及正確的路徑和副檔名非常重要。
我們正在使用的檔案可能存在於同一目錄或另一個目錄中。根據檔案的位置,路徑名會發生變化。
本文將討論如何在 Java 中訪問和指定檔案路徑。
讀取 Java 檔案
讀取檔案意味著獲取檔案的內容。我們使用 Java Scanner
類來讀取檔案。讓我們通過一個例子來理解這一點。
我們將首先編寫一個 Java 程式來建立一個新檔案。然後我們將編寫另一個 Java 程式來為這個新建立的檔案新增一些內容。
最後,我們將讀取這個檔案的內容。請注意,本文中的所有程式都不會在線上編譯器上執行(使用正確設定路徑的離線編譯器)。
建立檔案
import java.io.File;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
// Try catch block
try {
// Creating a file with the name demofile.txt
File myFile = new File("demofile.txt");
if (myFile.createNewFile()) {
System.out.println("The file is created with the name: " + myFile.getName());
} else {
System.out.println("The file already exists.");
}
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
輸出:
The file is created with the name: demofile.txt
該檔案在 Java 檔案所在的同一目錄中建立。所有 Java 程式檔案都在 C 目錄中。
向檔案中新增一些內容
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
try {
// create an object
FileWriter writeInFile = new FileWriter("demofile.txt");
// Adding content to this file
writeInFile.write("We are learning about paths in Java.");
writeInFile.close();
System.out.println("Successfully done!");
} catch (IOException x) {
System.out.println("An error is encountered.");
x.printStackTrace();
}
}
}
輸出:
Successfully done!
讀取檔案
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner; //scanner class for reading the file
public class Main {
public static void main(String[] args) {
try {
File myFile = new File("demofile.txt");
// create the scanner object
Scanner readFile = new Scanner(myFile);
while (readFile.hasNextLine()) {
String data = readFile.nextLine();
System.out.println(data);
}
readFile.close();
} catch (FileNotFoundException x) {
System.out.println("An error occurred.");
x.printStackTrace();
}
}
}
輸出:
We are learning about paths in Java.
請注意,在這裡,在 File()
方法中,我們只傳遞檔名及其副檔名。
我們沒有指定 demofile.txt
的完整路徑。demofile.txt
存在於我們儲存所有這些 Java 程式檔案的同一目錄和資料夾中。
但是,如果 demofile.txt
存在於另一個目錄或資料夾中,則讀取該檔案並不是那麼簡單。在這種情況下,我們指定檔案的完整路徑。
要獲取有關 Java 檔案系統的更多詳細資訊,請參閱此文件。
在 Java 中如何指定檔案路徑
為了指定檔案的路徑,我們在 File()
方法中傳遞檔名及其副檔名。我們這樣做。
new File("demofile.txt")
請注意,僅當檔案與工作專案的目錄位於同一資料夾中時,檔名才足夠。否則,我們必須指定檔案的完整路徑。
如果你不知道檔名,請使用 list()
方法。此方法返回當前目錄中所有檔案的列表。
在 java.io
庫的 File
類中,list()
方法以陣列的形式返回所有檔案和目錄的列表。它返回的輸出基於抽象路徑名定義的當前目錄。
如果抽象路徑名不表示目錄,則 list()
方法返回 null
。有時,我們不知道檔案的位置。
此外,當我們必須讀取的檔案不在當前工作目錄中時,就會發生錯誤。使用檔案的完整路徑可以更好地避免這些問題。
要獲取檔案的確切路徑,請使用下面給出的方法。
在 Java 中如何獲取檔案路徑
當我們不知道檔案的路徑時,可以使用 Java 的一些方法來查詢檔案的路徑。然後,我們可以將此路徑名指定為引數。
Java 提供三種型別的檔案路徑 - absolute
、canonical
和 abstract
。java.io.file
類具有三種查詢檔案路徑的方法。
在 Java 中使用 getPath()
方法獲取檔案路徑
getPath()
方法屬於 Java 的 File 類。它以字串形式返回抽象檔案路徑。
抽象路徑名是 java.io.file
的物件,它引用磁碟上的檔案。
語法:
file.getPath()
例子:
import java.io.*;
public class Main {
public static void main(String args[]) {
try {
// create an object
File myFile = new File("demo.txt");
// call the getPath() method
String path = myFile.getPath();
System.out.println("The path of this file is: " + path);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
輸出:
The path of this file is: demo.txt
正如你在上面的輸出中看到的,只有帶有副檔名的檔名是輸出。這表明該檔案存在於 Java 程式檔案所在的同一資料夾中。
在 Java 中使用 getAbsolutePath()
方法獲取檔案路徑
getAbsolutePath()
方法返回一個字串作為檔案的絕對路徑。如果我們使用絕對路徑名建立檔案,那麼 getAbsolutePath()
方法會返回路徑名。
但是,如果我們使用相對路徑建立物件,getAbsolutePath()
方法會根據系統解析路徑名。它存在於 Java 的 File 類中。
語法:
file.getAbsolutePath()
請注意,絕對路徑是給出系統中存在的檔案的完整 URL 的路徑,與它所在的目錄無關。另一方面,相對路徑給出檔案到當前目錄的路徑。
例子:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("pathdemo.txt");
// call the getAbsolutePath() method
String absolutePath = myFile.getAbsolutePath();
System.out.println("The Absolute path of the file is: " + absolutePath);
} catch (Exception e) {
System.err.println(e.getMessage());
}
}
}
輸出:
The Absolute path of the file is: C:\Users\PC\pathdemo.txt
請注意,這次我們獲得了完整的工作路徑,從當前工作目錄開始到檔案所在的當前資料夾。
因此,當你不知道任何檔案的位置時,請使用絕對路徑方法來查詢檔案所在的位置。然後,你可以在必須讀取該檔案時指定該路徑。
這樣,很容易找到要讀取的檔案。
在 Java 中使用 getCanonicalPath()
方法獲取檔案路徑
Path
類中的存在返回檔案的規範路徑。如果路徑名是規範的,則 getCanonicalPath()
方法返回檔案的路徑。
規範路徑始終是唯一且絕對的。此外,此方法刪除任何。
或路徑中的 ..
。
語法:
file.getCanonicalPath()
例子:
// import the java.io library
import java.io.*;
public class Main {
public static void main(String args[]) {
// try catch block
try {
// create the file object
File myFile = new File("C:\\Users");
// call the getCanonicalPath() method
String canonical = myFile.getCanonicalPath();
System.out.println("The Canonical path is : " + canonical);
} catch (Exception x) {
System.err.println(x.getMessage());
}
}
}
輸出:
The Canonical path is:C:\Users
要閱讀有關 Java 中路徑的更多資訊,請參閱此文件。
まとめ
在本文中,我們瞭解瞭如何在必須讀取檔案時指定檔案的路徑。我們還研究了 Java 提供的各種方法,如 getPath()
、getAbsolutePath()
和 getCanonical()
路徑來獲取檔案的路徑。
此外,我們看到了路徑如何根據檔案和當前專案的目錄和資料夾而變化。我們還建立並讀取了一些檔案作為演示。
但是,這些程式不會在線上編譯器上執行。