How to Write Error Log Into Files in Java
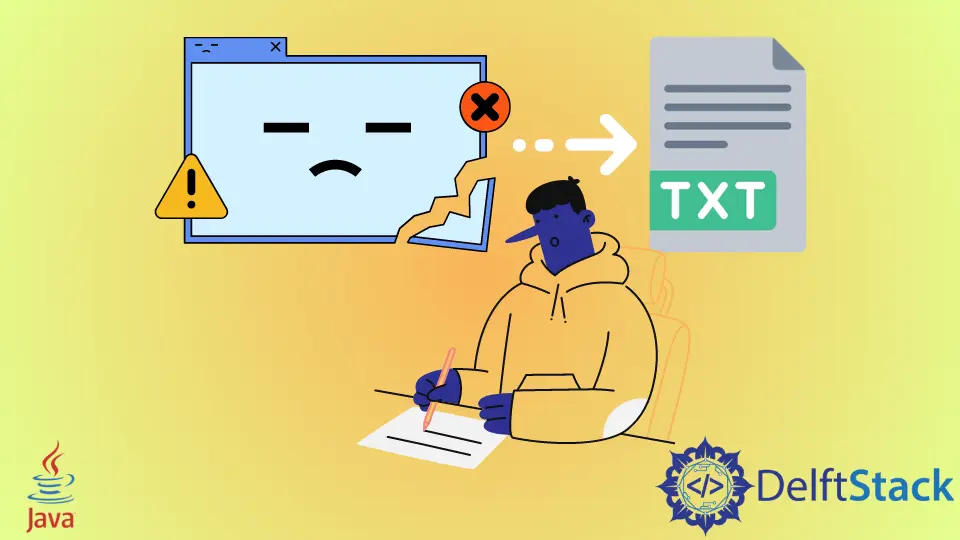
The most straightforward way to keep the log of errors in Java is to write the exceptions in files. We may use try
and catch
blocks to write the errors into text files using FileWriter
, BufferedWriter
, and PrintWriter
.
This tutorial will demonstrate how to keep a log of errors in Java.
Use FileWriter
to Write Error Log Into Files in Java
FileWriter
, BufferedWriter
, and PrintWriter
are the built-in functionalities in Java to create and write into files. See the example below on how to write errors into files using exceptions in Java.
package delftstack;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.PrintWriter;
public class Log_error {
public static void main(String[] args) {
try {
int[] Demo_Numbers = {1, 2, 3, 4, 5, 6, 7, 8};
System.out.println(Demo_Numbers[10]); // ArrayIndexOutOfBoundException
} catch (Exception e) {
appendToFile(e);
}
try {
Object reference = null;
reference.toString(); // NullPointerException
} catch (Exception e) {
appendToFile(e);
}
try {
int Number = 100 / 0; // ArithmeticException
} catch (Exception e) {
appendToFile(e);
}
try {
String demo = "abc";
int number = Integer.parseInt(demo); // NumberFormatException
} catch (Exception e) {
appendToFile(e);
}
}
public static void appendToFile(Exception e) {
try {
FileWriter New_File = new FileWriter("Error-log.txt", true);
BufferedWriter Buff_File = new BufferedWriter(New_File);
PrintWriter Print_File = new PrintWriter(Buff_File, true);
e.printStackTrace(Print_File);
} catch (Exception ie) {
throw new RuntimeException("Cannot write the Exception to file", ie);
}
}
}
The code above runs codes with different exceptions or errors and then writes them into a text file.
Output:
java.lang.ArrayIndexOutOfBoundsException: Index 10 out of bounds for length 8
at delftstack.Log_error.main(Log_error.java:11)
java.lang.NullPointerException: Cannot invoke "Object.toString()" because "reference" is null
at delftstack.Log_error.main(Log_error.java:19)
java.lang.ArithmeticException: / by zero
at delftstack.Log_error.main(Log_error.java:25)
java.lang.NumberFormatException: For input string: "abc"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67)
at java.base/java.lang.Integer.parseInt(Integer.java:668)
at java.base/java.lang.Integer.parseInt(Integer.java:786)
at delftstack.Log_error.main(Log_error.java:32)
The output above is written to the Error-log.txt
file:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook