How to Convert String to ArrayList in Java
-
Convert a String Into
ArrayList
Using thecharAt()
andadd()
Methods in Java -
Convert a String Into
ArrayList
Using thetoCharArray()
Method in Java -
the
split()
Method in Java -
Convert a String Into
ArrayList
Using thesplit()
Method in Java -
Create an
ArrayList
Using thesplit()
Method in Java -
Convert User String Into
ArrayList
in Java -
Convert a String Array Into
ArrayList
in Java -
Convert a String Into
ArrayList
in Java
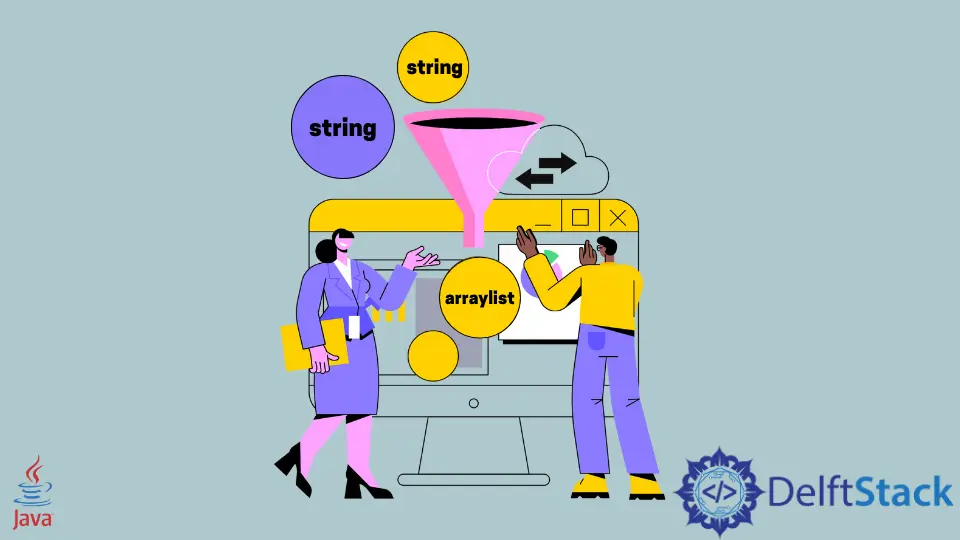
This tutorial introduces the conversion of String
to ArrayList
in Java and lists some example codes to understand the topic.
A string is defined as a sequence of characters, and an ArrayList
is used to store an ordered sequence of data. Converting a string to an ArrayList
requires us to take each character from the string and add it to the ArrayList
.
In this article, we’ll discuss the different ways by which we can do this.
Convert a String Into ArrayList
Using the charAt()
and add()
Methods in Java
A simple solution can be to iterate through each character of the string and add that character to the ArrayList
. We will use the charAt()
method to access the characters of the string, and then we can add them to the ArrayList
by using the add()
method.
The code for this approach is shown below.
import java.util.ArrayList;
public class SimpleTesting {
public static void main(String[] args) {
String s = "sample";
ArrayList<Character> list = new ArrayList<Character>();
for (int i = 0; i < s.length(); i++) {
char currentCharacter = s.charAt(i); // getting the character at current index
list.add(currentCharacter); // adding the character to the list
}
System.out.println("The String is: " + s);
System.out.print("The ArrayList is: " + list);
}
}
Output:
The String is: sample
The ArrayList is: [s, a, m, p, l, e]
We cannot use this approach if we wish to do something more advanced. For example, if we want to add just the words of a sentence to an ArrayList
and ignore the punctuations, further processing will be required.
Convert a String Into ArrayList
Using the toCharArray()
Method in Java
The toCharArray()
method can be used on a string to convert it into a character array. We can then iterate over this character array and add each character to the ArrayList
.
See the example below.
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
String s = "sample";
ArrayList<Character> list = new ArrayList<Character>();
char[] characterArray = s.toCharArray();
for (char c : characterArray) // iterating through the character array
list.add(c);
System.out.println("The String is: " + s);
System.out.print("The ArrayList is: " + list);
}
}
Output:
The String is: sample
The ArrayList is: [s, a, m, p, l, e]
This is a simple method that can be used if we don’t want to do anything complicated. However, just like the approach discussed in the previous section, we cannot use this if we’re going to do some processing on the string (like removing punctuations) before converting it to an ArrayList
.
the split()
Method in Java
The string split()
method takes a regular expression or pattern as a parameter and splits the string into an array of strings depending on the matching patterns. This method returns a string array.
For example, if we pass the string string of words
to the split()
method, and the pattern is single whitespace (denoted by //s+
), then it will return the array ["string", "of", "words"]
.
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String s = "string of words";
String[] strArr = s.split("\\s+"); // Splitting using whitespace
System.out.println("The String is: " + s);
System.out.print("The String Array after splitting is: " + Arrays.toString(strArr));
}
}
Output:
The String is: string of words
The String Array after splitting is: [string, of, words]
Convert a String Into ArrayList
Using the split()
Method in Java
We can create an ArrayList
from the returned array of strings by using the asList()
method of the Arrays
class. The following code demonstrates this.
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String s = "string of words";
String[] strArr = s.split("\\s+"); // Splitting using whitespace
ArrayList<String> list = new ArrayList<String>(Arrays.asList(strArr));
System.out.println("The String is: " + s);
System.out.print("The ArrayList is: " + list);
}
}
Output:
The String is: string of words
The ArrayList is: [string, of, words]
Create an ArrayList
Using the split()
Method in Java
The split()
method needs to be changed according to our needs. For example, if we want to create an ArrayList
of individual characters of the string sample
, then the split()
method needs a different regular expression.
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String s = "sample";
String[] strArr = s.split(""); // Splitting string into individual characters
ArrayList<String> list = new ArrayList<String>(Arrays.asList(strArr));
System.out.println("The String is: " + s);
System.out.print("The ArrayList is: " + list);
}
}
Output:
The String is: sample
The ArrayList is: [s, a, m, p, l, e]
Convert User String Into ArrayList
in Java
Suppose we take an input string from the user that contains comma-separated employee names, and we have to make an ArrayList
that includes each employee’s name.
We can use the split()
method to split the string into an array of employee names, and then we can simply convert it to an ArrayList
. The split()
method is perfect for this task as we need to remove the commas before creating the ArrayList
.
See the example below.
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
String employeeNames;
Scanner scanner = new Scanner(System.in);
System.out.println("Enter employee names separated by comma");
employeeNames = scanner.nextLine(); // taking user input
scanner.close();
String[] employeeNamesSplit = employeeNames.split(","); // Splitting names
ArrayList<String> list = new ArrayList<String>(Arrays.asList(employeeNamesSplit));
System.out.println("The String is: " + employeeNames);
System.out.print("The ArrayList is: " + list);
}
}
Output:
Enter employee names separated by comma
Justin, Jack, Jessica, Victor
The String is: Justin, Jack, Jessica, Victor
The ArrayList is: [Justin, Jack, Jessica, Victor]
Convert a String Array Into ArrayList
in Java
Arrays are great for storing data in an ordered fashion, but their limited size can restrict some important operations. We can simply convert a string array to an ArrayList
by using the asList()
method. It will simply copy all items of the array into the list.
The following code demonstrates this.
import java.util.ArrayList;
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[] strArr = {"Hello", "Hola", "Ola"};
ArrayList<String> strList = new ArrayList<String>(Arrays.asList(strArr));
System.out.println("The String Array is: " + Arrays.toString(strArr));
System.out.println("The Array List is: " + strList);
}
}
Output:
The String Array is: [Hello, Hola, Ola]
The Array List is: [Hello, Hola, Ola]
Convert a String Into ArrayList
in Java
Strings are commonly used for many different purposes, but they are immutable, and we cannot apply changes to them. An ArrayList
, on the other hand, provides a lot more flexibility. We can create an ArrayList
from the individual characters of the string, or if we need to do something more complicated (like creating an ArrayList
of names from a comma-separated string), we can use the split()
method.
Overall, the split()
method is the easiest and the most preferred way of converting a string to an ArrayList
.
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java
Related Article - Java ArrayList
- How to Sort Objects in ArrayList by Date in Java
- How to Find Unique Values in Java ArrayList
- Vector and ArrayList in Java
- Differences Between List and Arraylist in Java
- How to Compare ArrayLists in Java
- Merge Sort Using ArrayList in Java