How to Fix Error List Object Not Callable in Python
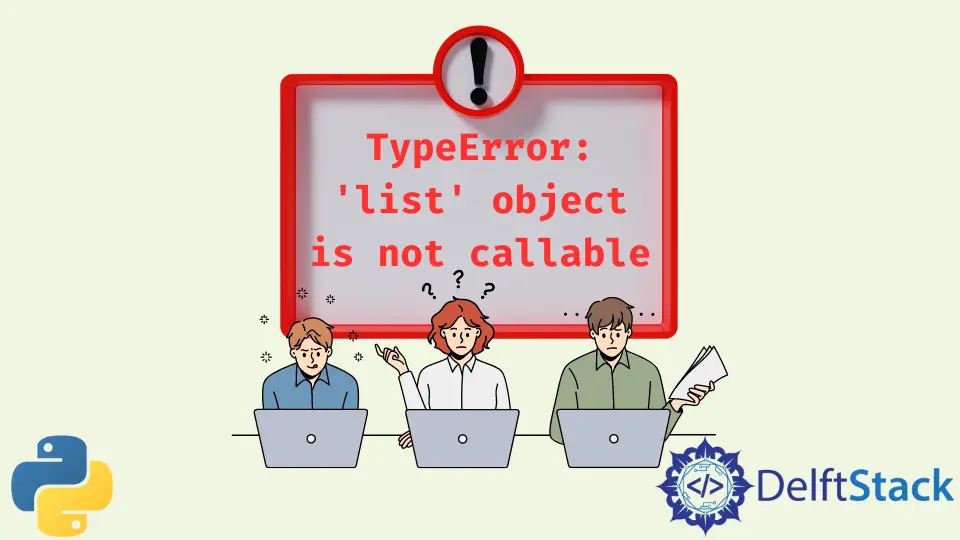
Types errors are some of the common standard exceptions in Python programs. They often result from failure to adhere to the correct syntax or an operation on an unsupported data type.
This error often arises when one attempts to call a non-callable object just like we would call a normal function object. The code snippet below returns a TypeError due to the violation of the correct python syntax.
nums = [23, 34, 56, 67]
nums()
Output:
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: 'list' object is not callable
An object is considered callable if a trailing pair of parentheses can trigger its execution, as with functions.
Fortunately, the Python standard also provides the callable()
function that returns True
if an object appears callable and False
if an object is not callable.
In the example above the list, the object is not callable, and therefore we would certainly get a False.
nums = [23, 34, 56, 67]
print(callable(nums))
Output:
False
Functions, methods, and classes are callables in Python.
This is because their execution can be invoked using the execution operator. The list of callable objects in Python includes lambda functions and custom-defined callable objects.
Data types such as tuples, lists, and dictionaries, on the other hand, are not callable. Therefore, any attempt to execute them as normal functions or methods in Python would result in a TypeError: object is not callable
.
The TypeError list object not callable
may also arise when indexing elements from lists using parentheses instead of square brackets.
In most programming languages, square brackets are considered the default indexing operators. However, in writing programs, we may often find ourselves using parentheses in place of square brackets due to their close resemblance.
The code snippet below is meant to return the element at index two in the list.
cars = ["Mazda", "Toyota", "BMW", "Tesla", "Hyundai"]
print(cars(2))
Output:
Traceback (most recent call last):
File "<string>", line 2, in <module>
TypeError: 'list' object is not callable
Although novices often conduct this mistake, it is also a common syntactical error committed by even experienced developers. This error can be resolved by simply using square brackets to index elements instead of parentheses, as shown below.
cars = ["Mazda", "Toyota", "BMW", "Tesla", "Hyundai"]
print(cars[2])
Output:
BMW
Using parentheses to perform indexing instead of square brackets is likely to occur when using list comprehension to shorten the Python syntax. This is because list comprehensions involve a combination of multiple square brackets and parentheses as opposed to the usual Python syntax.
In the example below, parentheses have been misused in constructing the list comprehension.
top_companies = [
["microsoft", "apple", "ibm"],
["tesla", "lucid", "nikola"],
["foxcon", "huawei", "tencent"],
]
result = [[row(index).upper() for index in range(len(row))] for row in top_companies]
print(result)
Output:
Traceback (most recent call last):
File "<string>", line 2, in <module>
File "<string>", line 2, in <listcomp>
File "<string>", line 2, in <listcomp>
TypeError: 'list' object is not callable
The example above intends to iterate through the nested lists using the indexes of the elements and convert its elements to uppercase. To resolve the error, we need to inspect the code and make sure that we use square brackets to index, as shown below.
top_companies = [
["microsoft", "apple", "ibm"],
["tesla", "lucid", "nikola"],
["foxcon", "huawei", "tencent"],
]
result = [[row[index].upper() for index in range(len(row))] for row in top_companies]
print(result)
Output:
[['MICROSOFT', 'APPLE', 'IBM'], ['TESLA', 'LUCID', 'NIKOLA'], ['FOXCON', 'HUAWEI', 'TENCENT']]
TypeError: list object is not callable
can also be encountered when a predefined name is used to name a variable. Some of the most commonly misused built-in names that could bring up an error of such a kind include; str
, dict
, list
, and range
.
In Python, the list constructor list()
is used to create new lists. Since this is a predefined built-in name and a class object representing a Python list, it isn’t good to use the name list as a variable name.
Using the name list to name variable could result in the error list object is not callable
as shown in the example below.
list = [24, 24, 25, 26, 28, 56]
nums_range = list(range(20, 40))
for number in list:
if number in nums_range:
print(number, "is the range")
else:
print(number, "number is not in range")
Output:
Traceback (most recent call last):
File "<string>", line 3, in <module>
TypeError: 'list' object is not callable
We used the predefined name list as a variable name in the example above. We attempt to use the same name as a constructor to create a new list in the second line.
Since we had already used this name as a variable name, Python interprets the second line as an attempt to call the list object, resulting in an error.
The above error can be resolved by renaming the list object to a different name. The new name should not be a keyword since it ensures that the list()
constructor retains its functional properties.
nums = [23, 24, 25, 28, 27, 35, 78]
nums_range = list(range(20, 40))
for number in nums:
if number in nums_range:
print(number, "is the range")
else:
print(number, "is not in the range")
Output:
23 is the range
24 is the range
25 is the range
28 is the range
27 is the range
35 is the range
78 is not in the range
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python
Related Article - Python Object
- Anonymous Objects in Python
- How to Add Attribute to Object in Python
- How to Check if a Python Object Has Attributes
- How to Fix Object Has No Attribute Error in Python
- How to Fix Object Is Not Subscriptable Error in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python