Anonymous Objects in Python
-
Create Anonymous Objects With the
namedtuple
Class in Python -
Create Anonymous Objects With the
type()
Function in Python
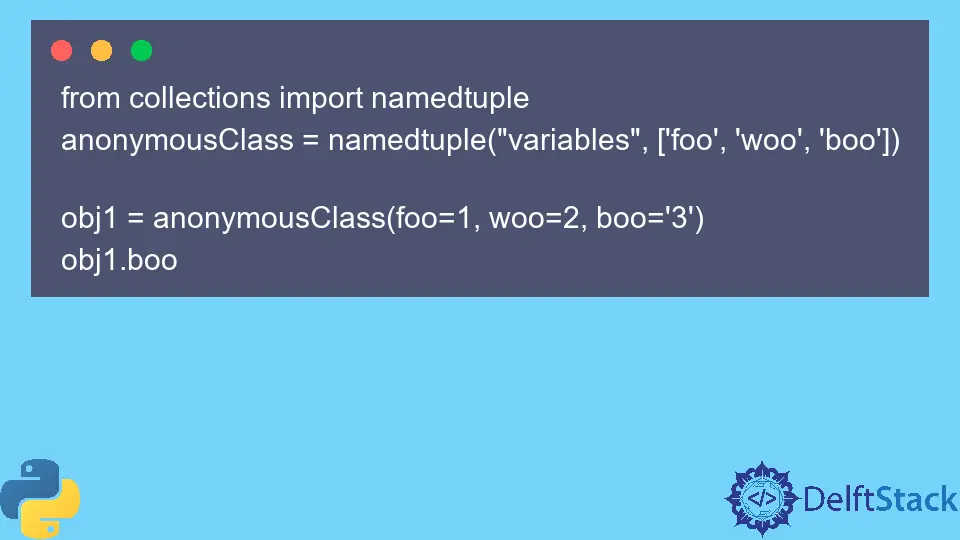
This tutorial will discuss the methods to create anonymous objects in Python.
Create Anonymous Objects With the namedtuple
Class in Python
An anonymous object is just a value without a real name. Hence, it is called Anonymous.
Sometimes it is easier to create an anonymous object for holding values than to define a whole new class.
By default, the Python programming language, unlike C# and Java, doesn’t natively support Anonymous classes. But, with a little creativity, we can find a workaround and mimic the functionality of anonymous objects.
For starters, we can utilize the namedtuple
class inside the collections
module of Python to mimic anonymous objects. The following code demonstrates how we can create anonymous objects using Python’s namedtuple
class.
from collections import namedtuple
anonymousClass = namedtuple("variables", ["foo", "woo", "boo"])
obj1 = anonymousClass(foo=1, woo=2, boo="3")
obj1.boo
Output:
'3'
We first initialized the named tuple anonymousClass
with namedtuple("variables", ['foo', 'woo', 'boo']
. In the constructor, we passed a key/value pair where the value is a list of variable names in which we’ll end up storing our temporary values.
In our use case, whatever we pass as the key of the constructor doesn’t have much effect on our outcome. We can now use this anonymousClass
object to create anonymous objects with obj1 = anonymousClass(foo=1, woo=2, boo='3')
.
The good thing about this method is that the anonymousClass
object is reusable, and we can create as many anonymous objects as we like. The main disadvantage of this approach is that we need to know the number of variables while initializing the namedtuple
.
Create Anonymous Objects With the type()
Function in Python
Another way of declaring an anonymous object is with the type()
function in Python. The type()
function takes 3 parameters; the name of the new data type, the data type of the values in it, and a set of values.
The following code snippet demonstrates how we can mimic the behavior of anonymous objects with the type()
function in Python.
obj = type("", (object,), {"foo": 1, "woo": 2, "boo": "3"})()
obj.boo
Output:
'3'
In the above code, we passed an empty string to the first parameter of the type()
function, which corresponds to the name of the new data type. The second parameter is the data type specified as object
.
The third parameter is a set of values given in key/value pairs where the key is the variable’s name, and the value is its corresponding value. Compared to our previous approach, this approach has its advantages and disadvantages.
The obvious disadvantage here is that we can’t reuse the obj
to initialize more anonymous objects, unlike the anonymousClass
in the previous example. The advantage of this approach is that we can specify more variables without declaring them first.
This type()
function can be modified to incorporate new values without specifying their name first by using dict
instead of object
data type. The following code snippet shows us the working of this method.
obj3 = type("", (dict,), {"foo": 1, "woob": 2})()
obj3.boo = 123
obj3.boo
Output:
123
In the above code, we replaced the object
from the previous example with dict
.
Both methods discussed above have their advantages and disadvantages over each other. None of them is a clear winner, and which one depends on our use case.
If we already know the number of values we’ll end up storing, but you need multiple anonymous objects, the namedtuple
method would work better. If we don’t know the number of values but only need one or two anonymous objects, the type()
method would be better.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn