How to Fix Object Has No Attribute Error in Python
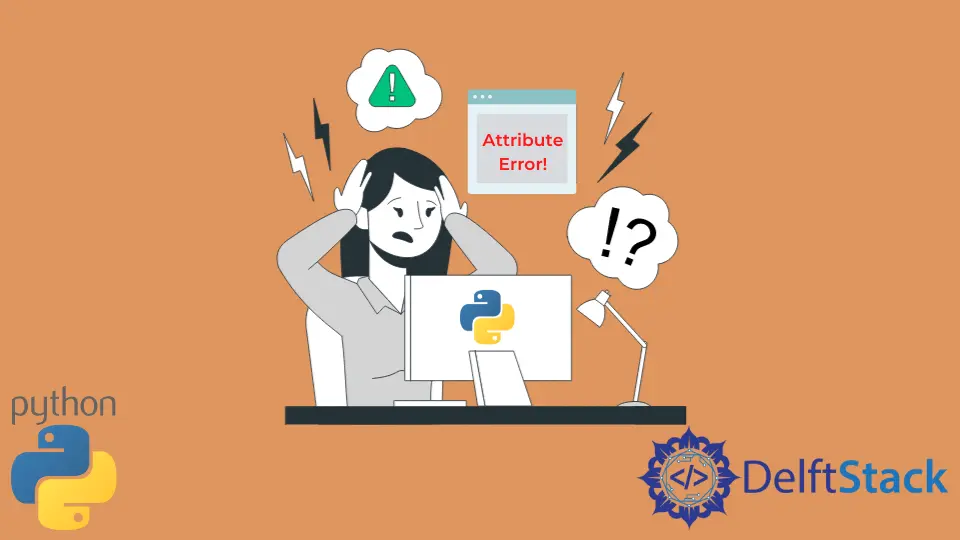
Attributes are functions or properties associated with an object of a class. Everything in Python is an object, and all these objects have a class with some attributes. We can access such properties using the .
operator.
This tutorial will discuss the object has no attribute python
error in Python. This error belongs to the AttributeError
type.
We encounter this error when trying to access an object’s unavailable attribute. For example, the NumPy
arrays in Python have an attribute called size
that returns the size of the array. However, this is not present with lists, so if we use this attribute with a list, we will get this AttributeError
.
See the code below.
import numpy as np
arr1 = np.array([8, 4, 3])
lst = [8, 4, 3]
print(arr1.size)
print(lst.size)
Output:
3
AttributeError: 'list' object has no attribute 'size'
The code above returns the size
of the NumPy
array, but it doesn’t work with lists and returns the AttributeError
.
Here is another example with user-defined classes.
class A:
def show():
print("Class A attribute only")
class B:
def disp():
print("Class B attribute only")
a = A()
b = B()
b.show()
Output:
AttributeError: 'B' object has no attribute 'show'
In the example above, two classes were initiated with similar functions to display messages. The error shows because the function called is not associated with the B
class.
We can tackle this error in different ways. The dir()
function can be used to view all the associated attributes of an object. However, this method may miss attributes inherited via a metaclass.
We can also update our object to the type that supports the required attribute. However, this is not a good method and may lead to other unwanted errors.
We can also use the hasattr()
function. This function returns True if an attribute belongs to the given object. Otherwise, it will return False.
See the code below.
class A:
def show():
print("Class A attribute only")
class B:
def disp():
print("Class B attribute only")
a = A()
b = B()
lst = [5, 6, 3]
print(hasattr(b, "disp"))
print(hasattr(lst, "size"))
Output:
True
False
In the example above, object b
has the attribute disp
, so the hasattr()
function returns True. The list doesn’t have an attribute size
, so it returns False.
If we want an attribute to return a default value, we can use the setattr()
function. This function is used to create any missing attribute with the given value.
See this example.
class B:
def disp():
print("Class B attribute only")
b = B()
setattr(b, "show", 58)
print(b.show)
Output:
58
The code above attaches an attribute called show
with the object b
with a value of 58
.
We can also have a code where we are unsure about the associated attributes in a try
and except
block to avoid any error.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn