How to Check if a Python Object Has Attributes
-
Check for Attributes Using the
hasattr()
Function in Python -
Check for Attributes Using the
getattr()
Function in Python - Conclusion
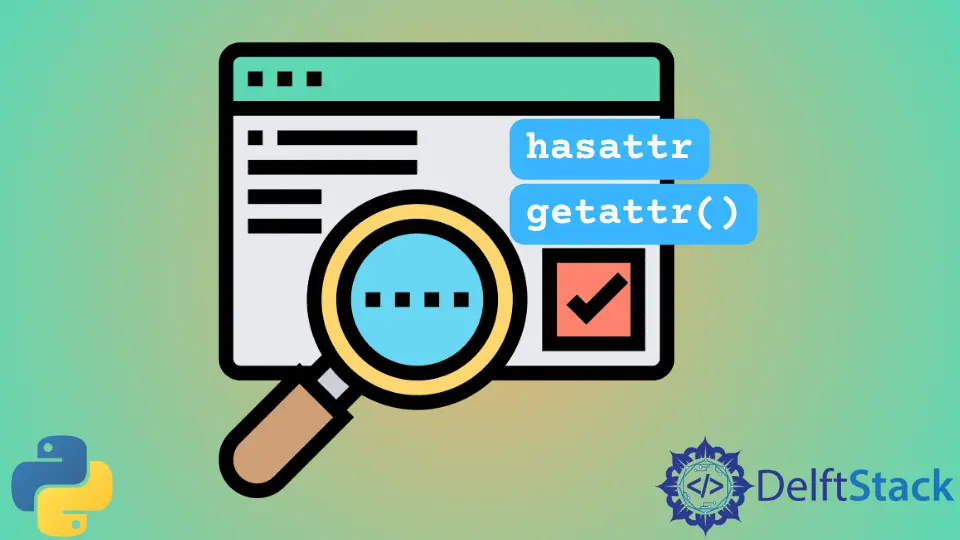
One of the fundamental aspects of programming is working with objects and their attributes. In Python, objects can have various attributes, which are essentially variables associated with the object.
These attributes provide essential information and behavior to the object, enabling us to effectively manipulate and interact with it. However, there may be scenarios where we must determine whether an object possesses a particular attribute before accessing or using it. This can be crucial for ensuring the correctness and robustness of our code.
To illustrate this concept, let’s consider a scenario where we have a class named Person
representing individuals. Each person
object can have attributes such as name, age, and occupation.
We want to perform certain actions based on whether a person
object possesses an attribute called occupation
. We can determine whether an object has a specific attribute without actually writing any code by utilizing the available tools and techniques in Python.
This article will show the different ways to check the attributes of an object.
Check for Attributes Using the hasattr()
Function in Python
The hasattr()
function in Python provides a convenient way to check if a Python object has specific attributes.
Syntax:
hasattr(object_name, attribute_name)
It takes two input parameters: the object to be checked and the attribute name as a string. The function returns a boolean value, True
if the object has the specified attribute, and False
otherwise.
By using hasattr()
, we can dynamically determine if an object possesses a particular attribute before accessing or manipulating it. This allows us to gracefully handle different cases in our code and avoid potential errors.
The following code segment demonstrates how we can utilize the hasattr()
function to determine whether an object has a certain attribute in Python.
class Cake:
best_cake = "Choco Lava"
best_flavor = "Vanilla"
cost = 2500
cake_object = Cake()
print(hasattr(Cake, "best_cake"))
Output:
In the given code, we have a class named Cake
with three attributes: best_cake
, best_flavor
, and cost
. The best_cake
attribute is set to "Choco Lava"
, the best_flavor
attribute is set to "Vanilla"
, and the cost
attribute is set to 2500
.
Next, an instance of the Cake
class is created using the Cake()
constructor, and the resulting object is assigned to the variable cake_object
.
To check if the Cake
class has the attribute best_cake
, the hasattr()
function is used. This function takes two arguments: the object or class to be checked, in this case, Cake
, and the attribute name as a string, in this case, best_cake
.
The hasattr()
function returns True
if the specified attribute is found in the object or class and False
otherwise.
Finally, the result of hasattr(Cake, 'best_cake')
is printed, which will output True
since the Cake
class indeed has the attribute best_cake
defined.
Use the hasattr()
Function With the if-Else
Block in Python
Another way to utilize the hasattr()
function is to use it with the if-else
block. The hasattr()
function returns True
when the attribute is present.
Thus, the if
block executes and prints the attribute’s value. But if the attribute is absent, then the value returned will be False
, and the else
block executes.
class Cake:
best_cake = "Choco Lava"
best_flavor = "Vanilla"
cost = 2500
cake_object = Cake()
if hasattr(Cake, "quantity"):
print(Cake.quantity)
else:
print("Whoops, no such attribute exists!")
Output:
In the above code, we use the hasattr()
function to check if the Cake
class has an attribute named quantity
. If it does, we print the value of Cake.quantity
. If the attribute doesn’t exist, we print the message Whoops, no such attribute exists!
.
This code demonstrates how to check if a Python class has a specific attribute using hasattr()
, allowing us to handle different attribute scenarios gracefully in our code.
Check for Attributes Using the getattr()
Function in Python
The getattr()
function in Python provides a way to dynamically retrieve the value of a specific attribute from an object or class.
Syntax
getattr(object_name, attribute_name)
It takes two input parameters: the object or class to be accessed and the attribute name as a string. The function returns the value of the attribute if it exists, and an optional default value is taken as the third parameter if the attribute is not found. If no default value is provided, the getattr()
function raises an AttributeError
exception.
By using getattr()
, we can programmatically check if a Python object or class has a particular attribute. This allows us to handle attribute-based operations and ensure the flexibility and robustness of our code. The getattr()
function is a valuable tool for checking Python attributes.
The following code segment demonstrates how we can utilize the getattr()
function to find out whether an object has a certain attribute in Python.
class Cake:
best_cake = "Choco Lava"
best_flavor = "Vanilla"
cost = 2500
cake_object = Cake()
print(getattr(Cake, "quantity"))
Output:
In the above code, we have a class called Cake
with three attributes: best_cake
, best_flavor
, and cost
. The best_cake
attribute is set to "Choco Lava"
, the best_flavor
attribute is set to "Vanilla"
, and the cost
attribute is set to 2500
.
An instance of the Cake
class is created using the Cake()
constructor and assigned to the variable cake_object
.
To check if the Cake
class has the attribute quantity
, the getattr()
function is used. This function takes two arguments: the object or class to be accessed, in this case, Cake
, and the attribute name as a string, in this case, quantity
.
Since the Cake
class does not have an attribute named quantity
, the getattr()
function will raise an AttributeError
. As a result, running the provided code will generate an error message indicating that the attribute does not exist.
Use the getattr()
Function With the Try-Except
Block
There is another way to use the getattr()
function; using the try-catch
block to check the presence of an attribute. The idea is to include the getattr()
function inside the try
block.
If the attribute is present, we will print its value. Otherwise, the AttributeError
will be thrown. We can handle this exception in the catch
block and print an error message using the print statement.
class Cake:
best_cake = "Choco Lava"
best_flavor = "Vanilla"
cost = 2500
cake_object = Cake()
try:
print(getattr(Cake, "quantity"))
except AttributeError:
print("Whoops, this attribute is not present!")
Output:
To check if the Cake
class has the attribute quantity
, the getattr()
function is used within a try-except block. The getattr()
function takes two arguments: the object or class to be accessed, in this case, Cake
, and the attribute name as a string, in this case, quantity
.
If the attribute exists, its value will be printed. If the attribute is not found, an AttributeError
will be raised, and the code will handle it by printing the message Whoops, this attribute is not present!
.
This code demonstrates how to use the getattr()
function to check if a Python object or class has specific attributes and handle potential attribute errors gracefully.
In contrast, we cannot use the hasattr()
function with the try-catch
block like the getattr()
function. This is because the hasattr()
function returns Boolean values and not the exceptions.
Conclusion
The hasattr()
focuses on checking the existence of an attribute, while the getattr()
focuses on retrieving the value of an attribute. The hasattr()
returns a boolean value, while getattr()
returns the attribute value or a default value.
In summary, hasattr()
is suitable for checking attribute existence, while getattr()
is useful for retrieving attribute values dynamically. Both methods serve different purposes but contribute to handling attribute-related tasks in Python effectively.