How to Add Attribute to Object in Python
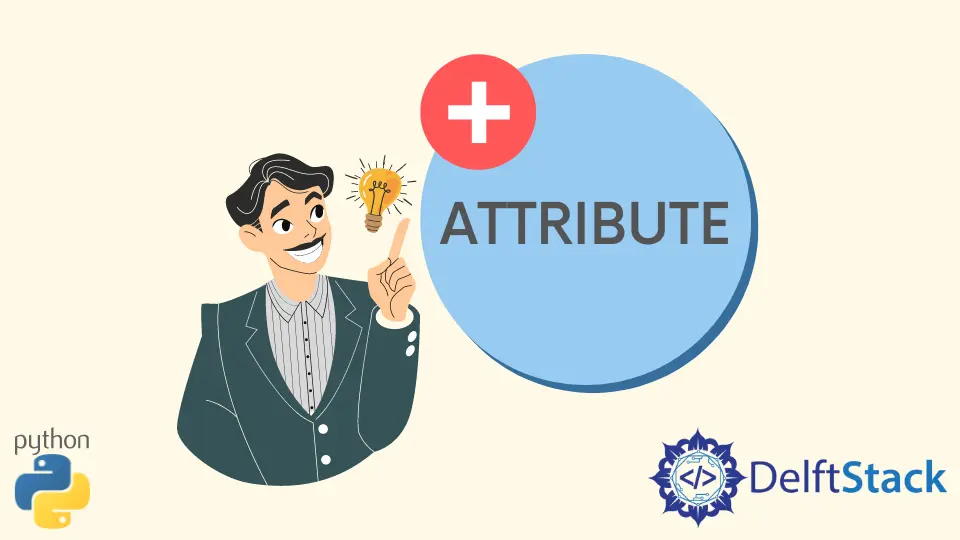
We will introduce how to add an attribute to an object in Python. We will also introduce how we can change the attributes of an object in Python with examples.
Add Attribute to Object in Python
In Python, we work with objects now and then because Python is an Object-oriented language. Objects make our coding reusable and easy to implement complex structures.
The main part of objects is their attributes. Attributes define what the properties of a certain object are.
While working with objects, there may be many situations where we need to add a new attribute to an object in the middle of the program.
Python provides a function setattr()
that can easily set the new attribute of an object. This function can even replace the value of the attribute.
It is a function with the help of which we can assign the value of attributes of the object.
This method will provide us with many ways to allocate values to variables by certain constructors and object functions. By using this function, we will also be able to have other substitutive ways to assign value.
Now, let’s discuss the structure of this setattr()
function. The structure which constructs a setattr()
is shown below.
# python
setattr(object, name, value)
As you can see from the syntax of this function, we pass three arguments into the function, and it will then allow us to set the attributes of an object.
There are three parameters which are stated as:
Object
- We will pass the object’s name we created and want to set attributes for.Name
- It will be the name of the attribute of that object that we want to assign value to.Value
- We will pass the attribute value here.
Let’s go through an example in which we will create a class of students. We will create a new student and assign it some attributes, as shown below.
# python
class Students:
name = "Rana Hasnain"
roll_no = "BC140402269"
cgpa = 3.5
new_student = Students()
setattr(new_student, "name", "James Bond")
setattr(new_student, "roll_no", "007")
setattr(new_student, "cgpa", 4)
print("New Student Name:", new_student.name)
print("New Student Roll #:", new_student.roll_no)
print("New Student Cgpa:", new_student.cgpa)
Output:
As you can see from the above example, it was quite easy to set the object’s attributes that we created. Now, let’s discuss a different scenario.
Let’s suppose we have a new object and want to set an attribute missing from the class.
In some cases, there are no attributes, or all attributes are not created in a class. When this happens, we assign a new attribute and can set a value for it.
But to make it happen, the object should implement the __dict__()
method. Let’s go through an example and try to assign values to an attribute that doesn’t exist.
We will use the above example and try to assign a new attribute, degree
, as shown below.
# python
class Students:
name = "Rana Hasnain"
roll_no = "BC140402269"
cgpa = 3.5
new_student = Students()
setattr(new_student, "name", "James Bond")
setattr(new_student, "roll_no", "007")
setattr(new_student, "cgpa", 4)
setattr(new_student, "degree", "BSCS")
print("New Student Name:", new_student.name)
print("New Student Roll #:", new_student.roll_no)
print("New Student Cgpa:", new_student.cgpa)
print("New Student Degree:", new_student.degree)
Output:
As you can see from the above example, this function can also create new attributes that don’t exist and assign values to them.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn