How to Fix Object Is Not Subscriptable Error in Python
- Understanding the Error
- Common Causes of the Error
- Method 1: Check Your Variable Types
- Method 2: Ensure Functions Return the Correct Type
- Method 3: Avoid Overwriting Variables
- Conclusion
- FAQ
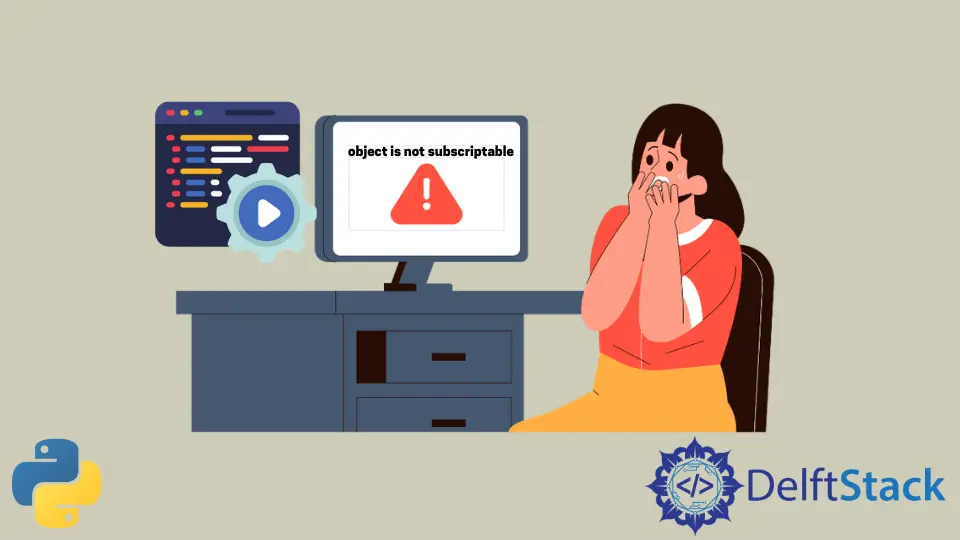
When coding in Python, encountering errors is a common experience, and one of the more perplexing ones is the “Object is not subscriptable” error. This error typically arises when you attempt to access an element of an object that does not support indexing or subscripting. Understanding why this happens and how to fix it can save you a lot of frustration.
In this article, we will explore the causes of this error and provide practical solutions with code examples. Whether you’re a beginner or an experienced developer, this guide will help you troubleshoot and resolve the “Object is not subscriptable” error effectively.
Understanding the Error
Before diving into solutions, it’s crucial to understand what the “Object is not subscriptable” error means. In Python, subscripting is a way to access elements in data structures like lists, tuples, and dictionaries using square brackets. When you try to index into an object that doesn’t support this operation—like an integer or a NoneType—you’ll encounter this error.
For instance, if you mistakenly treat an integer as a list, Python will raise an error. The message typically looks like this:
TypeError: 'int' object is not subscriptable
This error can occur in various scenarios, and knowing how to identify and fix it is essential for smooth coding.
Common Causes of the Error
The “Object is not subscriptable” error can arise from several common mistakes:
- Using an unsupported type: Attempting to index into a non-iterable type like an integer or None.
- Incorrect variable assignment: Overwriting a list with a non-iterable type, which leads to confusion in later code.
- Function returns: Functions that return None instead of a list or dictionary.
By recognizing these causes, you can better troubleshoot the error when it arises in your code.
Method 1: Check Your Variable Types
One of the first steps in resolving the “Object is not subscriptable” error is to check the types of the variables you are working with. You can use the type()
function to determine the data type of your variables. This is particularly useful if you’re unsure whether a variable is a list, tuple, or something else entirely.
Here’s an example:
my_variable = 5
print(type(my_variable))
print(my_variable[0])
Output:
<class 'int'>
TypeError: 'int' object is not subscriptable
In this case, my_variable
is an integer. When you try to access the first element using my_variable[0]
, Python raises a TypeError because integers are not subscriptable.
To fix this, ensure that you are working with a subscriptable type. If you intended my_variable
to be a list, you should initialize it as such:
my_variable = [5]
print(my_variable[0])
Output:
5
By checking the variable type before attempting to subscript, you can avoid this common error.
Method 2: Ensure Functions Return the Correct Type
Another common cause of the “Object is not subscriptable” error is when a function does not return the expected type. If you are relying on a function to return a list or dictionary but it inadvertently returns None, you will encounter this error when you try to subscript the result.
Consider the following example:
def get_data():
return None
data = get_data()
print(data[0])
Output:
TypeError: 'NoneType' object is not subscriptable
In this example, the function get_data()
returns None. When you attempt to access the first element with data[0]
, Python raises a TypeError.
To resolve this, ensure that your function returns the correct type. You can modify the function to return a list, for example:
def get_data():
return [1, 2, 3]
data = get_data()
print(data[0])
Output:
1
This change ensures that data
is a list, allowing you to successfully access its elements without encountering the error.
Method 3: Avoid Overwriting Variables
Sometimes, the “Object is not subscriptable” error occurs when you accidentally overwrite a variable that was previously a list or dictionary with a non-subscriptable type. This can happen in larger codebases where variable names are reused.
For example:
my_list = [1, 2, 3]
my_list = 10
print(my_list[0])
Output:
TypeError: 'int' object is not subscriptable
In this case, my_list
starts as a list, but it gets overwritten by an integer. When you try to access its first element, Python raises the error.
To fix this, be mindful of how you manage your variables. Use descriptive names and avoid reassigning them to different types. Here’s a better approach:
my_list = [1, 2, 3]
print(my_list[0])
Output:
1
By keeping your variable assignments clear and consistent, you can prevent this error from occurring.
Conclusion
The “Object is not subscriptable” error in Python can be frustrating, but understanding its causes and solutions can make it easier to handle. By checking variable types, ensuring functions return the correct types, and avoiding unintentional variable overwrites, you can effectively troubleshoot and fix this error. Remember, coding is a learning process, and each error presents an opportunity to deepen your understanding of Python.
FAQ
-
What does the “Object is not subscriptable” error mean?
It means you are trying to access an element of an object that does not support indexing, like an integer or None. -
How can I check the type of a variable in Python?
You can use thetype()
function to check the data type of a variable. -
Can a function return None and cause this error?
Yes, if a function is expected to return a list or dictionary but returns None, you will encounter this error when trying to subscript the result. -
What should I do if I accidentally overwrite a list variable?
Ensure you use descriptive variable names and avoid reassigning them to incompatible types to prevent this issue. -
Are there any tools to help debug this error in Python?
Yes, using an Integrated Development Environment (IDE) with debugging features can help you identify variable types and catch these errors early.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python