Invalid Syntax in Python
- Invalid Syntax in Python
- Incorrect Indentation in Python
- Missing Parenthesis in Python
- Missing Colon in Python
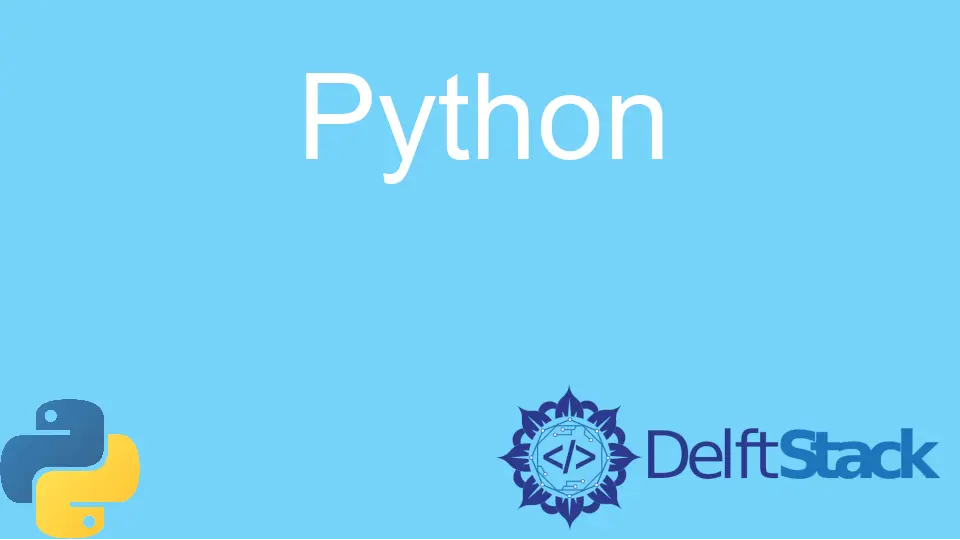
We will introduce reasons that could cause an invalid syntax error message in Python with the help of examples.
Invalid Syntax in Python
One common error in Python is invalid syntax errors, and misplaced symbols, syntax rule violations, or incorrect indentation mostly cause them. Let’s discuss some common mistakes that can lead to invalid syntax error messages in Python.
Incorrect Indentation in Python
Python is an indent-sensitive language that relies heavily on correct indentation; if there is an incorrect indentation, Python will receive an error message.
Let’s have an example of an incorrect indentation that can lead to error messages.
# Python
def print_message():
print("Message Printed!")
print_message()
Output:
From the result above, our code threw an error because when defining a function in Python, there should be an indent in the next line after the colon.
Let’s add the correct indent and check whether it resolves the error.
# Python
def print_message():
print("Message Printed!")
print_message()
Output:
By adding the correct indentation, we can avoid syntax error messages in Python.
Missing Parenthesis in Python
Another reason for getting invalid syntax could be that we could have forgotten to close any parenthesis in our code. This can only occur if we use too many parentheses and forget to close any of them, but if we only use one or two parentheses, it will likely not happen.
Let’s go through an example and generate the invalid syntax error message.
# Python
def multiple_of_10(num):
new_num = 5
return num * new_num * (new_num + 5
result=multiple_of_10(5)
print(result)
Output:
From the above example, we forgot to close one of the parentheses. Now, let’s resolve this error and check the results below.
# Python
def multiple_of_10(num):
new_num = 5
return num * new_num * (new_num + 5)
result = multiple_of_10(5)
print(result)
Output:
From the above example, we can avoid syntax error messages in Python by correctly closing the parenthesis.
Missing Colon in Python
Another reason for getting invalid syntax could be that we could have forgotten to add a colon after declaring our function name in our code. There are rare chances, but sometimes we can make mistakes and must remember to add a colon.
Let’s have an example and generate the invalid syntax error message as shown below.
# Python
def multiple_of_10(num)
new_num = 5
return num * new_num * (new_num + 5
result=multiple_of_10(5)
print(result)
Output:
We should have added a colon after our function declaration. Now, let’s resolve this error and check the results below.
# Python
def multiple_of_10(num):
new_num = 5
return num * new_num * (new_num + 5)
result = multiple_of_10(5)
print(result)
Output:
With just little mistakes, we can get invalid syntax error messages. It is common, and we can easily point out the mistakes with the help of error messages shown by the compiler.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python