How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
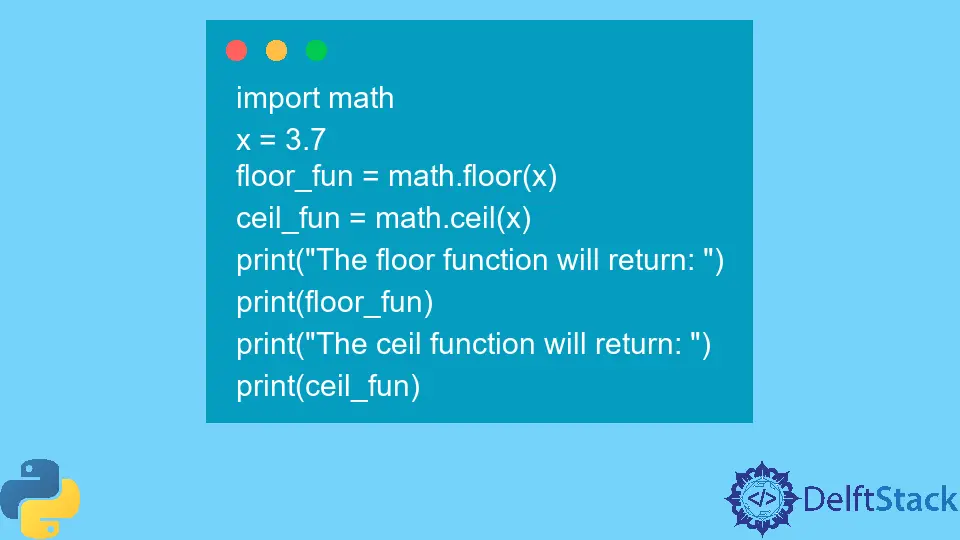
We will introduce why a TypeError: 'float' object cannot be interpreted as an integer
error occurs and how we can solve it in Python.
Fix the TypeError: 'float' object cannot be interpreted as an integer
in Python
Python is a programming language used widely in many fields, including scientific computing, data analysis, and machine learning. One of Python’s most common data types is the floating-point number, also known as float
.
However, when working with the float
data type in Python, it’s important to remember that we cannot interpret float
as an integer.
In this article, we’ll explore the reasons behind this limitation and how to work with float
and integer objects in Python. First, let’s start by understanding the difference between a float
and an integer.
An integer is a whole number without any decimal points, while a float
is a number that has a decimal point or a fraction.
For example, the number 7 is an integer, while 8.0 is a float
. Similarly, 9.6 is also a float
.
While both data types represent numbers, they are not interchangeable in Python. They can throw errors whenever they are interpreted as one another.
For example, we have a variable that should contain an integer but instead got a float
, as shown below.
x = 3.5
for a in range(x):
print(a)
This will throw an error, as shown below.
As seen from the example, the type of the variable in Python is determined automatically based on the assigned values. For example, if we assign 3 to a variable x
, it will be of data type integer; on the other hand, if we assign 3.5 to a variable y
, it will be a data type of float
, as shown below.
x = 3
y = 3.5
print(type(x))
print(type(y))
The output of the above code is shown below.
There are two possible solutions to get rid of this error in Python. The first solution is to ensure that we always pass the integer data type where an integer is required.
We can easily do that by using the built-in method of Python int()
, which converts any other data types to int
.
Let’s have an example where we will pass the float
variable to the int()
method, as shown below.
x = 3.5
y = int(x)
for i in range(y):
print(i)
The output of the above code will be as shown below.
As the above solution shows, we can easily convert any data type to an integer using a simple method, int()
. Another solution for this error is using the math.floor()
or math.ceil()
functions.
First, we must import the math
library into our project to use these functions.
The math
library is the standard library in Python installed with Python. If you are getting an error on the import math
line in the code, you can easily install it using the following command.
pip install math
Once the library is installed, we can import it into our project using the following line of code.
import math
Before we jump to the solution, it is important to know a little detail about the two functions we will use in this example, math.floor()
and math.ceil()
. The math.floor()
function is used to round the given floating point number down to the nearest integer that’s less than or equal to the provided number.
The math.ceil()
function is used to round the given floating point number up to the nearest integer greater than or equal to the provided number. We can use any of the above functions based on our needs.
Examples of these functions are shown below.
import math
x = 3.7
floor_fun = math.floor(x)
ceil_fun = math.ceil(x)
print("The floor function will return: ")
print(floor_fun)
print("The ceil function will return: ")
print(ceil_fun)
The output of the above code is shown below.
As seen from the example, the math.floor()
function rounds down the number; on the other hand, the math.ceil()
function rounds up the number to the nearest integer.
In conclusion, whenever we attempt to use a float
object as an integer in Python, it results in a TypeError: 'float' object cannot be interpreted as an integer
. It is important to be mindful of the data types used in our code and convert them properly as needed to avoid this error.
The math
library provides useful functions such as math.floor()
and math.ceil()
for rounding floating-point numbers to the nearest integer. By being aware of these issues and utilizing the appropriate methods, we can avoid common errors and ensure that our code runs smoothly.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn