How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
-
Fix the
TypeError: Object of type 'int64' is not JSON serializable
in Python -
Convert
int64
toint
or Float Data Type in Python -
Convert
int64
to String Data Type in Python
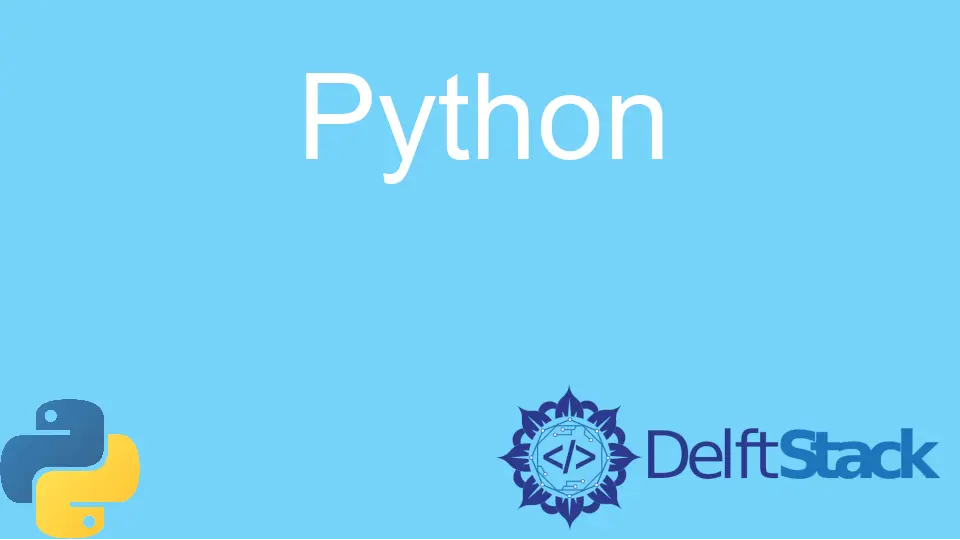
We will introduce how to correctly serialize JSON without getting errors like the Object of type 'int64' is not JSON serializable
with examples in Python.
Fix the TypeError: Object of type 'int64' is not JSON serializable
in Python
Python is a high-level interpreted programming language with many libraries that can be used for various tasks in various fields, such as web development, data analysis, artificial intelligence, scientific computing, and many more. Python is most commonly used to handle JSON format in web development.
While handling JSON format, many programmers face a common error message: Object of type 'int64' is not JSON serializable
.
This article will explore why this error occurs and how we can easily resolve it. First, we must understand what JSON is and why it is vital in web development and modern web applications.
JSON is a lightweight data format that is readable and understandable for humans and machines; it is easy to parse and generate.
It is most commonly used to transmit data from a server to a client or between two servers. The json.dump()
and json.load()
are Python’s most common built-in JSON functions for encoding and decoding JSON data. These functions are part of the json
module in Python.
The json.dump()
is used to convert an object to a JSON format and write them into a file or a string. While the json.load()
is used to parse the JSON format string and decode it back to a Python object.
The error Object of type 'int64' is not JSON serializable
occurs when we try to encode the Python object to a JSON format.
The main reason behind this error is that the JSON format does not support the int64
data type. The int64
data type represents large scientific or computational numbers requiring high precision.
JSON format only supports limited data types such as numbers, strings, Boolean and null values.
Two possible solutions exist to resolve this error without losing the precision of the number we want to convert. The possible solutions are discussed below.
Convert int64
to int
or Float Data Type in Python
An easy way to get rid of this error and make your program work is by just converting the int64
data type to a simple int
or float
data type by using these simple functions int()
will convert it to a simple integer and float()
will convert it to a float data type.
Let’s go through an example in which we will convert a simple number to a data type of int64
, so we can regenerate the error and then apply the solution and see the result below.
import json
import numpy as np
num_data = {"number": np.int64(789)}
json_conversion = json.dumps({"Data": num_data["number"]})
print(json_conversion)
As you can see from the above example, we imported the json
module first, allowing us to use the json.dumps()
function to encode Python objects into JSON format. We also imported the NumPy
module because it allows us to convert a simple number into an int64
data type using its function np.int64()
.
When we tried to convert the Python object to JSON format, it gave us the same error: Object of type 'int64' is not JSON serializable
.
Let’s implement both solutions and check how it resolves the error, as shown below.
import json
import numpy as np
num_data = {"number": np.int64(789)}
json_conversion = json.dumps({"Data": int(num_data["number"])})
print(json_conversion)
Output:
Let’s use float()
to solve the error, as shown below.
import json
import numpy as np
num_data = {"number": np.int64(789)}
json_conversion = json.dumps({"Data": float(num_data["number"])})
print(json_conversion)
Output:
Convert int64
to String Data Type in Python
Let’s discuss the second solution, which is as simple as the first one. We can also use a built-in function to convert the int64
data type to a string.
The str()
function is used to convert any data type into a string and is supported by JSON, and it can also be used to represent numerical values. Let’s use the above example and try this solution, as shown below.
import json
import numpy as np
num_data = {"number": np.int64(789)}
json_conversion = json.dumps({"Data": str(num_data["number"])})
print(json_conversion)
Output:
As we can see from the above examples, it is quite easy to solve this error by just using the built-in functions in Python to convert int64
into an integer, float, or string data type.
We hope this article has helped you resolve the issue.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python