How to Fix Value Error Need More Than One Value to Unpack in Python
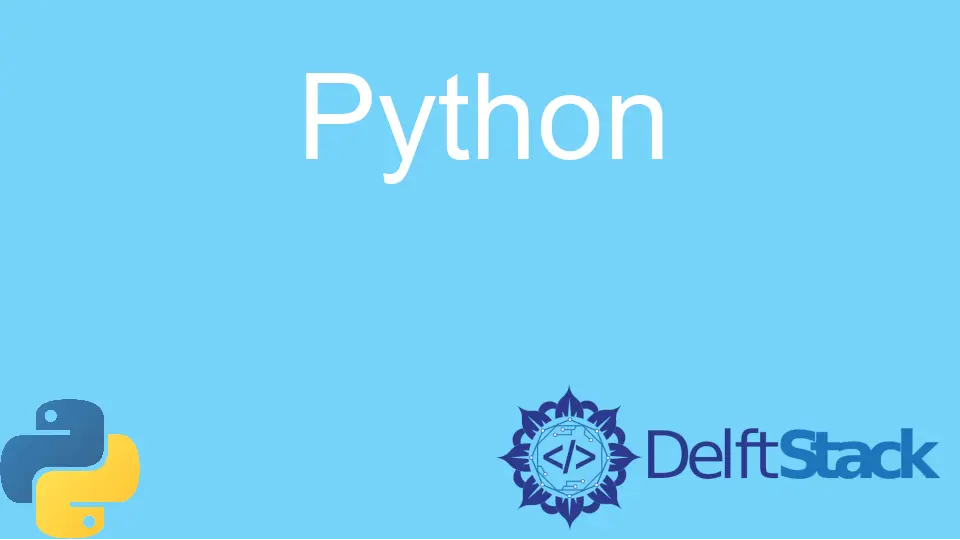
We will introduce the ValueError: Need More Than One Value To Unpack
error message in Python and how to resolve it with examples.
the ValueError: Need More Than One Value To Unpack
in Python
While trying to unpack a sequence in Python, many developers come across the error message "ValueError: Need more than one value to unpack"
.
First, we need to understand what is unpacking
in Python. It is known as unpacking when we try to assign a list or tuple of values to a list or tuple of variables.
This error message occurs when the number of values and variables mismatch. For example, if we try to assign 3 values to 4 variables, it will give this error.
Let’s create a program and try to regenerate this error message, as shown below.
Code Example:
# Python
my_values = ["Apple", "Mango", "Guava"]
a, b, c, d = my_values
Output:
From the above example, our program will throw an error message when we assign fewer values to more variables or more values to fewer variables.
Two solutions can easily resolve this error message. The first solution is to assign the correct number of values to the correct number of variables.
Let’s resolve the above example by adding another variable to our list.
Code Example:
# Python
my_values = ["Apple", "Mango", "Guava", "Banana"]
a, b, c, d = my_values
print(a)
print(b)
print(c)
print(d)
Output:
Now, the code is executed without any error message.
Another way to resolve this error message is by using the *
operator, the unpacking operator. The solution is very simple and easy to use.
Let’s go through an example: we will unpack a list, assign the first three elements to variables, and use the unpacking operator for the rest of the values.
Code Example:
# Python
my_values = ["Apple", "Mango", "Guava", "Banana", "Strawberry"]
a, b, c, *rest_fruits = my_values
print(a)
print(b)
print(c)
print(rest_fruits)
Output:
The first three variables contain only a single fruit name, while the fourth one contains a list of two fruits because the number of variables and values are mismatched. This way, we can solve the error message "ValueError: need more than one value to unpack"
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python