Can Only Concatenate List (Not Int) to List in Python
- Understanding the Error
- Method 1: Convert Integer to a List
-
Method 2: Using the
append()
Method -
Method 3: Using the
extend()
Method - Conclusion
- FAQ
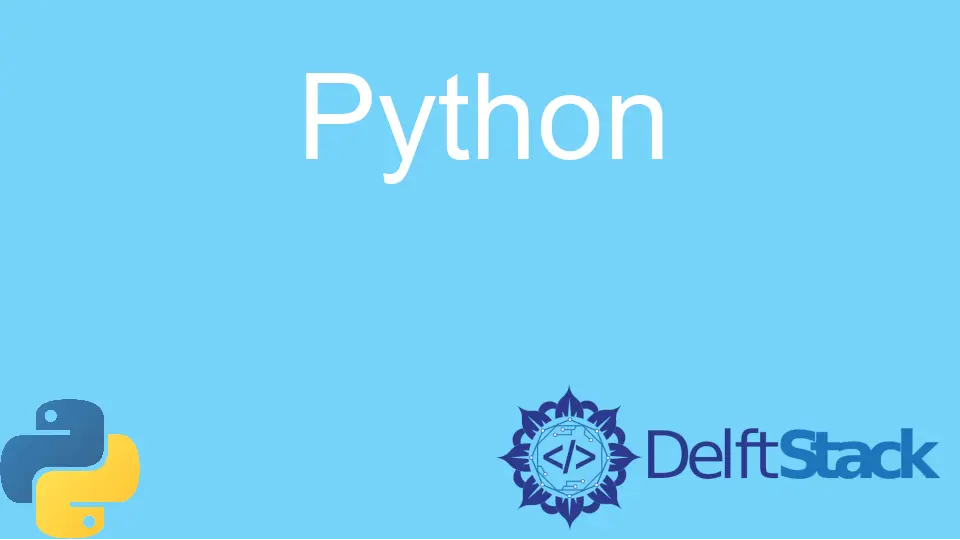
When working with Python, you may encounter a frustrating error: “can only concatenate list (not int) to list.” This error typically arises when you mistakenly try to add an integer to a list using the concatenation operator (+
). Understanding how to correctly manipulate lists in Python is essential for any coder, whether you’re a beginner or an experienced developer.
In this article, we will explore effective methods to resolve this error, ensuring that you can confidently handle list operations. We’ll provide clear code examples and detailed explanations to help you grasp the concept, enabling you to enhance your Python programming skills.
Understanding the Error
Before we dive into solutions, it’s vital to understand why this error occurs. In Python, the +
operator is used for concatenation, which allows you to join two lists together. However, if you attempt to concatenate a list with an integer, Python raises a TypeError since it doesn’t know how to combine these two different data types.
For instance, if you have a list and you try to add an integer to it, like this:
my_list = [1, 2, 3]
result = my_list + 5
You will receive an error message similar to this:
Output:
TypeError: can only concatenate list (not "int") to list
This error highlights the importance of ensuring that both operands in a concatenation operation are of the same type. Let’s explore several methods to fix this issue.
Method 1: Convert Integer to a List
One of the simplest ways to resolve the “can only concatenate list (not int) to list” error is to convert the integer into a list before concatenation. You can achieve this using the []
brackets to create a single-element list from the integer.
Here’s how you can do it:
my_list = [1, 2, 3]
my_int = 5
result = my_list + [my_int]
Output:
[1, 2, 3, 5]
In this example, we create a new list containing the integer 5
by enclosing it in square brackets. Then, we concatenate this new list with my_list
. This method is straightforward and effective, ensuring that both operands are lists, which prevents the TypeError.
By converting the integer to a list, you can easily add it to existing lists without any issues. This approach is particularly useful when you want to append single values to lists dynamically.
Method 2: Using the append()
Method
Another effective method to add an integer to a list is by using the append()
method. This method allows you to add an element to the end of a list without needing to perform concatenation.
Here’s how to do it:
my_list = [1, 2, 3]
my_int = 5
my_list.append(my_int)
Output:
[1, 2, 3, 5]
In this case, the append()
method modifies the original list, my_list
, by adding my_int
to its end. This approach is advantageous because it doesn’t require creating a new list, thus keeping your code cleaner and more efficient.
Using append()
is often preferred when you want to add elements one at a time. It’s a clear and intuitive way to handle list modifications, especially when working with loops or conditional statements.
Method 3: Using the extend()
Method
If you want to add multiple integers or elements to a list at once, the extend()
method is a great option. This method takes an iterable (like a list or tuple) and adds its elements to the end of the specified list.
Here’s how to implement it:
my_list = [1, 2, 3]
my_ints = [4, 5]
my_list.extend(my_ints)
Output:
[1, 2, 3, 4, 5]
In this example, my_ints
is a list of integers. By using the extend()
method, we add all the elements from my_ints
to my_list
. This method is particularly useful when you have multiple items to add, as it allows for a single operation instead of multiple append()
calls.
Using extend()
helps maintain clean and efficient code, especially when dealing with larger datasets or when you need to combine multiple lists.
Conclusion
Encountering the “can only concatenate list (not int) to list” error in Python can be a common hurdle for many programmers. However, by understanding the nature of list operations and employing the methods we’ve discussed—converting integers to lists, utilizing append()
, and leveraging extend()
—you can effectively overcome this issue. Each approach has its advantages, allowing you to choose the one that best fits your specific programming needs. With these techniques in your toolkit, you can confidently manipulate lists in Python without running into data type errors.
FAQ
-
What causes the “can only concatenate list (not int) to list” error?
This error occurs when you try to concatenate a list with an integer using the+
operator, which is not allowed in Python. -
How can I add an integer to a list without causing an error?
You can convert the integer to a list using square brackets or use theappend()
method to add it directly to the list. -
What is the difference between
append()
andextend()
?
Theappend()
method adds a single element to the end of a list, whileextend()
adds multiple elements from an iterable to the list. -
Can I concatenate multiple lists together?
Yes, you can concatenate multiple lists using the+
operator or theextend()
method. -
Is there a performance difference between
append()
andextend()
?
Yes,extend()
can be more efficient when adding multiple elements at once, as it minimizes the number of operations compared to multipleappend()
calls.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedInRelated Article - Python Error
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python