How to Get the Average of a List in Python
-
Use
statistics
Library to Get the Average of a List -
Find the Average of Python List Using
sum()/len()
-
Find the Average of Python List Using
sum()/float(len())
in Python 2
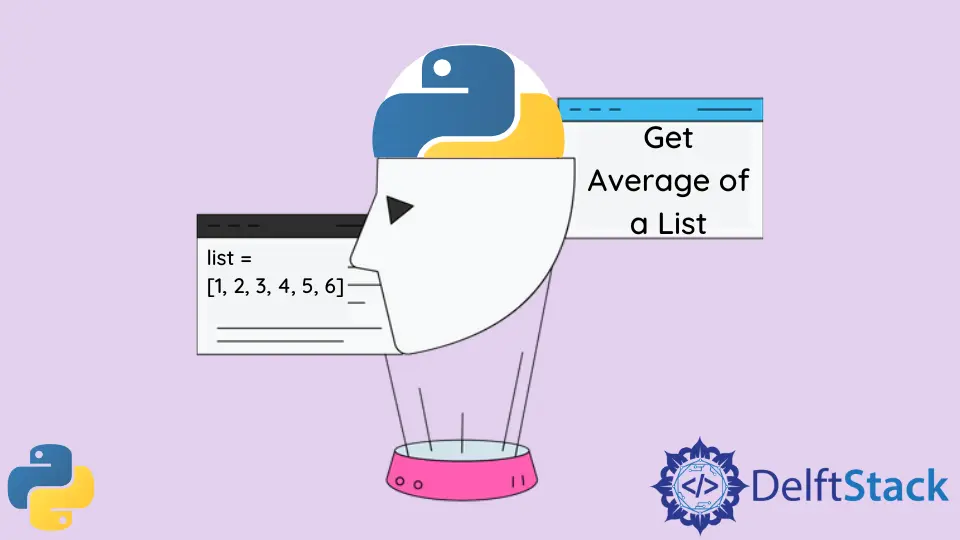
This tutorial introduces how to find the average of a list in Python. It also lists some example codes to further clarify the concept as the methods have changed from previous versions of Python.
Use statistics
Library to Get the Average of a List
If you are using Python 3.4+, you can use newly introduced statistics
library. This library contains multiple mathematical functions that can be used straightforwardly. Since we want to calculate the mean of the given list so we will use statistics.mean(list)
. list
is the list of numbers. This function will return the mean (average) of the given list.
The basis example to use this statistics.mean()
method is shown below,
import statistics
list = [1, 2, 3, 4, 5, 6]
mean = statistics.mean(list)
print(mean)
Output:
3.5
Find the Average of Python List Using sum()/len()
Using statistics
library for calculating the average of a list is not the only option. The average of the list can be calculated by simply dividing the sum of elements by them number of elements.
sum(list)
obtains the sum of the given list, and len(list)
returns the length of the list.
data = [1, 2, 3, 4, 5, 6]
mean = sum(data) / len(data)
print(mean)
Output:
3.5
Find the Average of Python List Using numpy.mean()
We can also use the numpy.mean()
function to get the average of a list in Python. The average is taken over the flattened array by default, otherwise over the specified axis.
However, you need to first install the NumPy
module before using it.
The example code to get the average of a list using numpy.mean()
is as follows.
import numpy
data = [1, 2, 3, 4, 5, 6]
mean = numpy.mean(data)
print(mean)
Output:
3.5
Find the Average of Python List Using sum()/float(len())
in Python 2
If your Python version is 2.x, then you are not able to use the statistics
module and have to use the simple mathematical formula to calculate the average of a given list.
For Python 2 you will need to convert len
to a float to get float division. The code will look like this:
data = [1, 2, 3, 4, 5, 6]
mean = sum(data) / float(len(data))
print(mean)
Output:
3.5
If you do not convert len
to float, you will not get the floating number but an integer, as shown below.
data = [1, 2, 3, 4, 5, 6]
mean = sum(data) / len(data)
print(mean)
Output:
3
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn