How to Convert a Dictionary to a List in Python
-
Convert Keys From Dictionary to a List Using
keys()
Method in Python -
Convert Values From Dictionary to a List Using
values()
Method in Python - Convert Dictionary Into a List of Tuples in Python
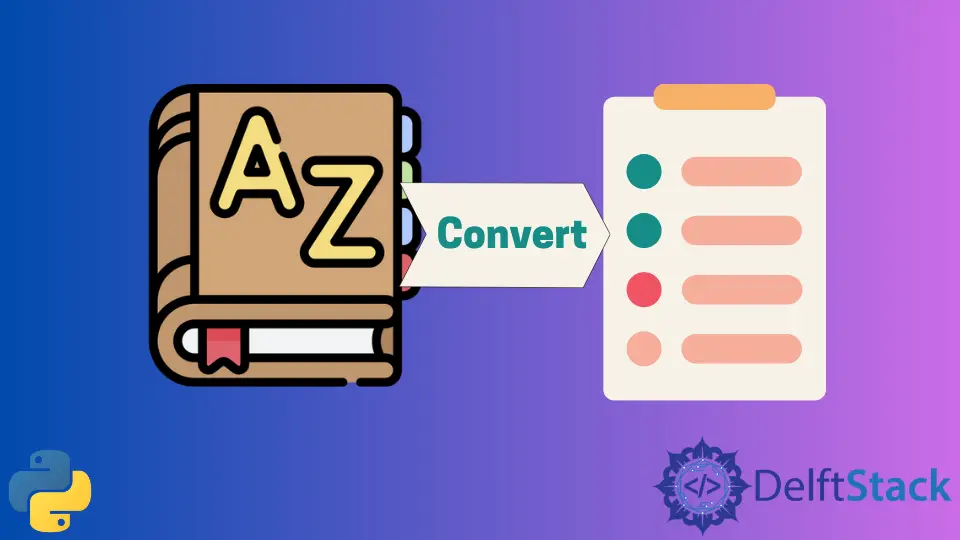
A Dictionary in Python is a combination of key-value
pairs. With the help of the key
, you can access the values inside the dictionary. Now converting a dictionary to a list in Python can mean different things. Either,
- You can separate the keys and convert them into a List
- You can separate the values and convert them into a List
- Convert the key & value pair of the dictionary to a List of Tuple.
Convert Keys From Dictionary to a List Using keys()
Method in Python
Suppose you have a dictionary named as dictionary
with four key-value
pairs.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfKeys = dictionary.keys()
print("Type of variable listOfKeys is: ", type(listOfKeys))
listOfKeys = list(listOfKeys)
print("Printing the entire list containing all Keys: ")
print(listOfKeys)
print("Printing individual Keys stored in the list:")
for key in listOfKeys:
print(key)
Output:
Type of variable listOfKeys is: <class 'dict_keys'>
Printing the entire list containing all Keys:
['a', 'b', 'c', 'd']
Printing individual Keys stored in the list:
a
b
c
d
You can use the keys()
method, which will extract all the keys
from the dictionary and then store the result (all the keys) inside the variable listOfKeys
.
Now you have to convert the variable listOfKeys
into a list with the help of the list()
function because at this point if you check the type of the variable listOfKeys
then it will be <class 'dict_keys'>
. That’s why we are changing the type of the variable to List
and then storing it in the same variable i.e listOfKeys
.
At this point, you have successfully separated all the keys from the dictionary using the keys()
function and have converted them into a list. Finally, To print the elements inside the list, you have to use a for
loop, which iterates over each element and prints it.
Convert Values From Dictionary to a List Using values()
Method in Python
The values()
method works the same way as keys()
method. The only difference is that the values()
method returns all the dictionary values instead of the keys.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfValues = dictionary.values()
print("Type of variable listOfValues is: ", type(listOfValues))
listOfValues = list(listOfValues)
print("Printing the entire list containing all values: ")
print(listOfValues)
print("Printing individual values stored in the list:")
for val in listOfValues:
print(val)
Output:
Type of variable listOfValues is: <class 'dict_values'>
Printing the entire list containing all values:
['Apple', 'Banana', 'Cherries', 'Dragon Fruit']
Printing individual values stored in the list:
Apple
Banana
Cherries
Dragon Fruit
You use the values()
function on the dictionary
and then storing the result returned by the values()
function inside the variable listOfValues
.
If you check the type of the variable listOfValues
then its type will be of the class <class 'dict_values'>
. But you want it to be of class <class 'list'>
. Therefore, you have to convert the variable listOfValues
into a list using the list()
function and then storing the result in the same variable i.e listOfValues
.
At last, print all the values inside the list listOfValues
using a loop.
Convert Dictionary Into a List of Tuples in Python
A dictionary can be converted into a List of Tuples using two ways. One is the items()
function, and the other is the zip()
function.
1. items()
Function
The items()
function converts the dictionary elements into <class 'dict_items'>
and the result of which is stored in the myList
variable. The myList
needs to be converted into a list, so for that, you have to typecast it using list()
function and then store the result in the same variable i.e. myList
.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.items()
print(type(myList))
myList = list(myList)
print(myList)
Output:
<class 'dict_items'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
2. zip()
Function
The zip()
function takes two arguments first is the key using keys()
function and second is the value using values()
function from the dictionary. It then returns a zip
object that you can store inside a variable, in this case, myList
.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = zip(dictionary.keys(), dictionary.values())
print(type(myList))
myList = list(myList)
print(myList)
Output:
<class 'zip'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
Now you have to convert the zip
object into a list
using the list()
function. Then you can store the final result inside the same variable called myList
or inside a different variable depending upon your requirement and at the end print the entire list.
3. iteritems()
in Python 2.x
In Python v2.x, you also have a iteritems()
function, which works almost the same way as the items()
function.
The iteritems()
takes each element from the dictionary
and converts it into a different type. If you check the type of the variable myList
, it will be of type <type 'dictionary-itemiterator'>
. So, you have to convert it into a List using the function list()
. If you check the type of the variable myList
then it will be of type <type 'list'>
and if you print it then the elements will be in the form of a list of tuples.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.iteritems()
print("Type of myList is: ", type(myList))
myList = list(myList)
print(myList)
Output:
('Type of myList is: ', <type 'dictionary-itemiterator'>)
[('a', 'Apple'), ('c', 'Cherries'), ('b', 'Banana'), ('d', 'Dragon Fruit')]
Ensure that you run the above code on the Python v2.x compiler; otherwise, you will get an error because we don’t have this method in Python v3.x.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - Python Dictionary
- How to Check if a Key Exists in a Dictionary in Python
- How to Get All the Files of a Directory
- How to Find Maximum Value in Python Dictionary
- How to Sort a Python Dictionary by Value
- How to Merge Two Dictionaries in Python 2 and 3