在 Python 中将字典转换为列表
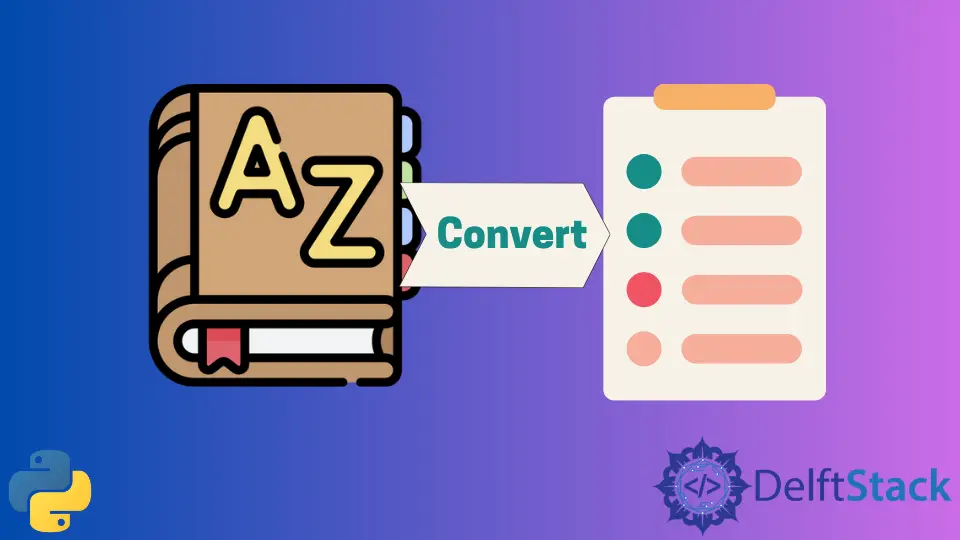
Python 中的字典是由键-值
对组合而成。在 key
的帮助下,你可以访问字典里面的值。现在,在 Python 中把一个字典转换为一个列表可能意味着不同的事情。要么是
- 你可以将键分开,并将其转换为一个列表。
- 你可以将值分开,并将其转换为一个列表。
- 将字典中的键和值对转换为元组列表。
在 Python 中使用 keys()
方法将键从字典转换为列表
假设你有一个名为 dictionary
的字典,有四键值对。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfKeys = dictionary.keys()
print("Type of variable listOfKeys is: ", type(listOfKeys))
listOfKeys = list(listOfKeys)
print("Printing the entire list containing all Keys: ")
print(listOfKeys)
print("Printing individual Keys stored in the list:")
for key in listOfKeys:
print(key)
输出:
Type of variable listOfKeys is: <class 'dict_keys'>
Printing the entire list containing all Keys:
['a', 'b', 'c', 'd']
Printing individual Keys stored in the list:
a
b
c
d
你可以使用 keys()
方法,它将从字典中提取所有 keys
,然后将结果(所有 keys)存储在变量 listOfKeys
中。
现在你必须在 list()
函数的帮助下将变量 listOfKeys
转换成一个列表,因为此时如果你检查变量 listOfKeys
的类型,那么它将是 <class 'dict_keys'>
。这就是为什么我们要将变量的类型改为 List
,然后将其存储在同一个变量中,即 listOfKeys
。
至此,你已经成功地使用 keys()
函数将所有键从字典中分离出来,并将它们转换为一个列表。最后,要打印列表中的元素,你必须使用 for
循环,它遍历每个元素并打印它。
在 Python 中使用 values()
方法将字典中的值转换为列表
values()
方法的工作方式与 keys()
方法相同。唯一的区别是 values()
方法返回所有的字典值而不是键。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfValues = dictionary.values()
print("Type of variable listOfValues is: ", type(listOfValues))
listOfValues = list(listOfValues)
print("Printing the entire list containing all values: ")
print(listOfValues)
print("Printing individual values stored in the list:")
for val in listOfValues:
print(val)
输出:
Type of variable listOfValues is: <class 'dict_values'>
Printing the entire list containing all values:
['Apple', 'Banana', 'Cherries', 'Dragon Fruit']
Printing individual values stored in the list:
Apple
Banana
Cherries
Dragon Fruit
你在 dictionary
上使用 values()
函数,然后将 values()
函数返回的结果存储在变量 listOfValues
中。
如果你检查变量 listOfValues
的类型,那么它的类型将是 <class 'dict_values'>
类。但你希望它是 <class 'list'>
类。因此,你必须使用 list()
函数将变量 listOfValues
转换为一个列表,然后将结果存储在同一个变量中,即 listOfValues
。
最后,使用循环打印列表 listOfValues
内的所有值。
在 Python 中把字典转换为元组列表
一个字典可以通过两种方式转换成一个元组列表。一种是 items()
函数,另一种是 zip()
函数。
1. items()
函数
items()
函数将字典元素转换为 <class 'dict_items'>
,其结果存储在 myList
变量中。myList
需要被转换为一个列表,因此,你必须使用 list()
函数将其类型化,然后将结果存储在同一个变量中,即 myList
。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.items()
print(type(myList))
myList = list(myList)
print(myList)
输出:
<class 'dict_items'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
2. zip()
函数
zip()
函数需要两个参数,第一个是使用 keys()
函数的 key,第二个是使用字典中的 values()
函数的 value。然后它返回一个 zip
对象,你可以将其存储在一个变量中,在本例中,myList
。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = zip(dictionary.keys(), dictionary.values())
print(type(myList))
myList = list(myList)
print(myList)
输出:
<class 'zip'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
现在你必须使用 list()
函数将 zip
对象转换成 list
。然后,你可以将最终的结果存储在同一个名为 myList
的变量中,或者根据你的要求存储在不同的变量中,最后打印整个列表。
3. Python 2.x 中的 iteritems()
在 Python v2.x 中,你还有一个 iteritems()
函数,它的工作方式和 items()
函数几乎一样。
iteritems()
从 dictionary
中获取每个元素并将其转换为不同的类型。如果你检查变量 myList
的类型,它将是 <type 'dictionary-itemiterator'>
类型。所以,你必须使用函数 list()
将其转换为 List。如果你检查变量 myList
的类型,那么它的类型将是 <type 'list'>
,如果你打印它,那么元素将以一个元组列表的形式出现。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.iteritems()
print("Type of myList is: ", type(myList))
myList = list(myList)
print(myList)
输出:
('Type of myList is: ', <type 'dictionary-itemiterator'>)
[('a', 'Apple'), ('c', 'Cherries'), ('b', 'Banana'), ('d', 'Dragon Fruit')]
确保你在 Python v2.x 编译器上运行上述代码;否则,你会得到一个错误,因为在 Python v3.x 中没有此方法。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn