Python で辞書をリストに変換する
-
Python の
keys()
メソッドを使って辞書からリストにキーを変換する -
Python の
values()
メソッドを用いて辞書からリストに値を変換する - Python で辞書をタプルのリストに変換する
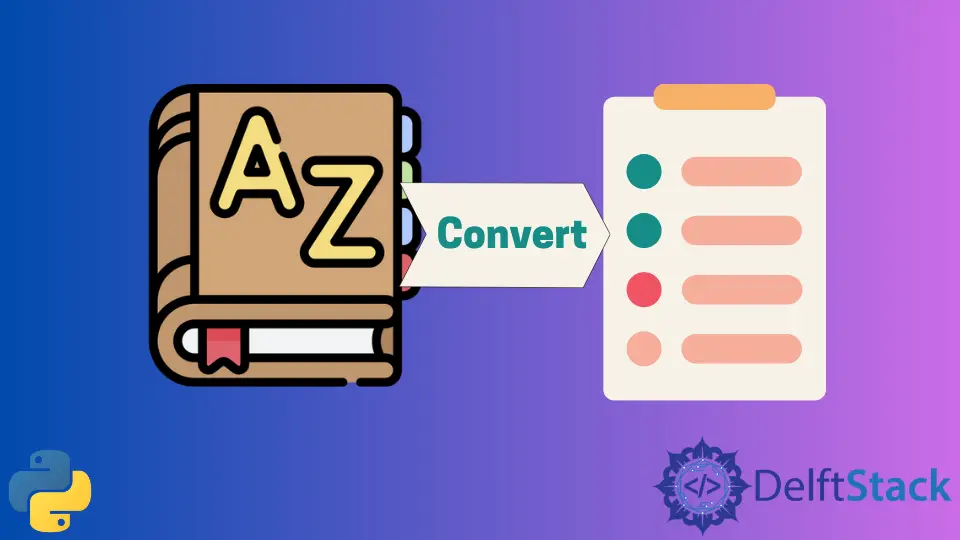
Python の辞書は key-value
のペアを組み合わせたものです。key
の助けを借りて、辞書内の値にアクセスすることができます。さて、Python で辞書をリストに変換することは異なる意味を持ちます。どちらかです。
- キーを分離してリストに変換することができます。
- 値を分離してリストに変換することができます。
- 辞書のキーと値のペアをタプルのリストに変換します。
Python の keys()
メソッドを使って辞書からリストにキーを変換する
4つの key-value
ペアを持つ dictionary
という名前の辞書があるとします。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfKeys = dictionary.keys()
print("Type of variable listOfKeys is: ", type(listOfKeys))
listOfKeys = list(listOfKeys)
print("Printing the entire list containing all Keys: ")
print(listOfKeys)
print("Printing individual Keys stored in the list:")
for key in listOfKeys:
print(key)
出力:
Type of variable listOfKeys is: <class 'dict_keys'>
Printing the entire list containing all Keys:
['a', 'b', 'c', 'd']
Printing individual Keys stored in the list:
a
b
c
d
これは辞書からすべての key
を抽出し、その結果 (すべてのキー) を変数 listOfKeys
に格納します。
ここで、変数 listOfKeys
の型を確認すると <class 'dict_keys'>
となるので、list()
関数の助けを借りて変数 listOfKeys
をリストに変換しなければなりません。この時点で変数 listOfKeys
の型を確認すると、<class 'dict_keys'>
になってしまうからです。
この時点で、keys()
関数を使って辞書からすべてのキーを分離し、リストに変換したことになります。最後に、リスト内の要素を表示するには、for
ループを使用しなければなりません。
Python の values()
メソッドを用いて辞書からリストに値を変換する
values()
メソッドは keys()
メソッドと同じように動作します。唯一の違いは、values()
メソッドがキーの代わりにすべての辞書の値を返すことです。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfValues = dictionary.values()
print("Type of variable listOfValues is: ", type(listOfValues))
listOfValues = list(listOfValues)
print("Printing the entire list containing all values: ")
print(listOfValues)
print("Printing individual values stored in the list:")
for val in listOfValues:
print(val)
出力:
Type of variable listOfValues is: <class 'dict_values'>
Printing the entire list containing all values:
['Apple', 'Banana', 'Cherries', 'Dragon Fruit']
Printing individual values stored in the list:
Apple
Banana
Cherries
Dragon Fruit
関数 values()
を用いて dictionary
の値を取得し、その結果を変数 listOfValues
の中に格納します。
変数 listOfValues
の型を確認すると、その型はクラス <class 'dict_values'>
になります。しかし、あなたはそれをクラス <class 'list'>
にしたいと考えています。したがって、変数 listOfValues
を list()
関数を使ってリストに変換し、その結果を同じ変数 listOfValues
に格納しなければなりません。
最後に、ループを使って listOfValues
のリスト内のすべての値を表示します。
Python で辞書をタプルのリストに変換する
辞書をタプルのリストに変換するには、2つの方法があります。一つは items()
関数であり、もう一つは zip()
関数です。
1. items()
関数
関数 items()
は辞書の要素を <class 'dict_items'>
に変換し、その結果を変数 myList
に格納します。myList
はリストに変換する必要があるので、そのためには list()
関数を使って型キャストし、その結果を同じ変数 myList
に格納する必要があります。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.items()
print(type(myList))
myList = list(myList)
print(myList)
出力:
<class 'dict_items'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
2. zip()
関数
関数 zip()
は 2つの引数を取り、1つ目は keys()
関数を用いたキー、2つ目は辞書の values()
関数を用いた値を受け取ります。そして、zip
オブジェクトを返します。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = zip(dictionary.keys(), dictionary.values())
print(type(myList))
myList = list(myList)
print(myList)
出力:
<class 'zip'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
ここで、zip
オブジェクトを list()
関数を使って list
に変換しなければなりません。そして、最終的な結果を myList
という同じ変数の中に格納するか、必要に応じて別の変数の中に格納し、最後にリスト全体を出力します。
3. Python 2.x の iteritems()
関数
Python v2.x には iteritems()
関数もあり、items()
関数とほぼ同じように動作します。
iteritems()
は dictionary
から各要素を取り出し、異なる型に変換します。変数 myList
の型を調べると、型は <type 'dictionary-itemiterator'>
です。そのため、関数 list()
を用いてリストに変換する必要があります。変数 myList
の型を確認すると、型は <型 'list'>
であり、それを出力すると要素はタプルのリストの形になります。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.iteritems()
print("Type of myList is: ", type(myList))
myList = list(myList)
print(myList)
出力:
('Type of myList is: ', <type 'dictionary-itemiterator'>)
[('a', 'Apple'), ('c', 'Cherries'), ('b', 'Banana'), ('d', 'Dragon Fruit')]
上記のコードを Python v2.x コンパイラで実行していることを確認してください。そうしないと、Python v3.x にこのメソッドがないため、エラーが発生します。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn関連記事 - Python Dictionary
- Python でディレクトリのすべてのファイルを取得する方法
- Python 辞書で最大値を求める
- Python 辞書を値でソートする方法
- Python 2 および 3 で 2つの辞書をマージする方法
- Python 辞書から要素を削除する方法