Python에서 사전을 목록으로 변환
-
Python에서
keys()
메서드를 사용하여 키를 사전에서 목록으로 변환 -
Python에서
values()
메서드를 사용하여 사전의 값을 목록으로 변환 - Python에서 사전을 튜플 목록으로 변환
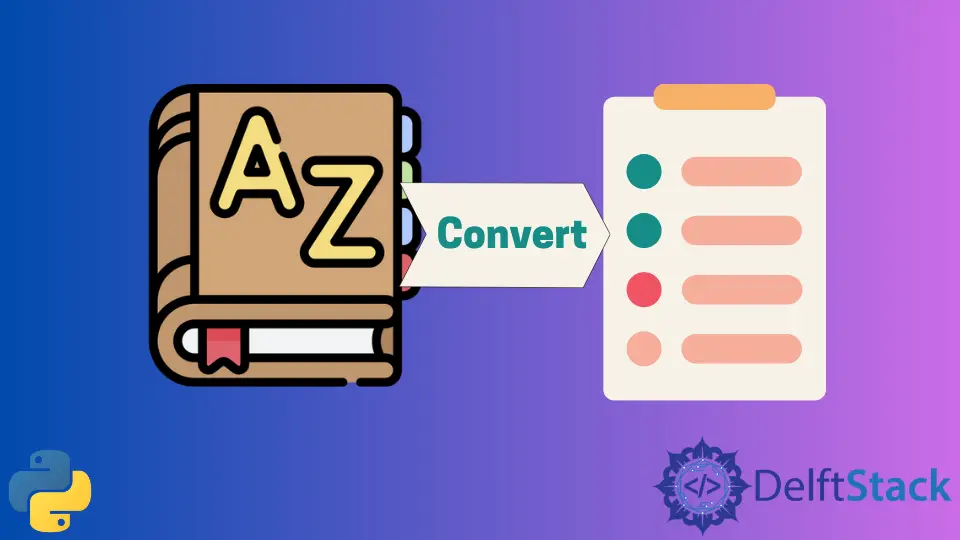
Python의 사전은키-값
쌍의 조합입니다. 키
의 도움으로 사전 내의 값에 액세스 할 수 있습니다. 이제 파이썬에서 사전을 목록으로 변환하는 것은 다른 의미를 가질 수 있습니다. 어느 한 쪽,
- 키를 분리하여 목록으로 변환 할 수 있습니다.
- 값을 분리하여 목록으로 변환 할 수 있습니다.
- 사전의 키 및 값 쌍을 튜플 목록으로 변환합니다.
Python에서keys()
메서드를 사용하여 키를 사전에서 목록으로 변환
네 개의키-값
쌍이있는dictionary
라는 사전이 있다고 가정하십시오.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfKeys = dictionary.keys()
print("Type of variable listOfKeys is: ", type(listOfKeys))
listOfKeys = list(listOfKeys)
print("Printing the entire list containing all Keys: ")
print(listOfKeys)
print("Printing individual Keys stored in the list:")
for key in listOfKeys:
print(key)
출력:
Type of variable listOfKeys is: <class 'dict_keys'>
Printing the entire list containing all Keys:
['a', 'b', 'c', 'd']
Printing individual Keys stored in the list:
a
b
c
d
keys()
메소드를 사용하면 사전에서 모든keys
를 추출한 다음 결과 (모든 키)를listOfKeys
변수에 저장할 수 있습니다.
이제listOfKeys
변수를list()
함수를 사용하여 목록으로 변환해야합니다.이 시점에서 변수listOfKeys
의 유형을 확인하면<class 'dict_keys'>
가됩니다. 이것이 변수의 유형을 List
로 변경 한 다음 동일한 변수 즉 listOfKeys
에 저장하는 이유입니다.
이제keys()
함수를 사용하여 사전에서 모든 키를 성공적으로 분리하고 목록으로 변환했습니다. 마지막으로, 목록 안에있는 요소를 인쇄하려면for
루프를 사용해야합니다.이 루프는 각 요소를 반복하여 인쇄합니다.
Python에서values()
메서드를 사용하여 사전의 값을 목록으로 변환
values()
메소드는keys()
메소드와 동일한 방식으로 작동합니다. 유일한 차이점은values()
메서드가 키 대신 모든 사전 값을 반환한다는 것입니다.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfValues = dictionary.values()
print("Type of variable listOfValues is: ", type(listOfValues))
listOfValues = list(listOfValues)
print("Printing the entire list containing all values: ")
print(listOfValues)
print("Printing individual values stored in the list:")
for val in listOfValues:
print(val)
출력:
Type of variable listOfValues is: <class 'dict_values'>
Printing the entire list containing all values:
['Apple', 'Banana', 'Cherries', 'Dragon Fruit']
Printing individual values stored in the list:
Apple
Banana
Cherries
Dragon Fruit
dictionary
에서values()
함수를 사용한 다음values()
함수가 반환 한 결과를listOfValues
변수에 저장합니다.
변수listOfValues
의 유형을 확인하면 해당 유형은<class 'dict_values'>
클래스가됩니다. 하지만<class 'list'>
클래스가되기를 원합니다. 따라서list()
함수를 사용하여listOfValues
변수를 목록으로 변환 한 다음 결과를 동일한 변수 즉listOfValues
에 저장해야합니다.
마지막으로 루프를 사용하여listOfValues
목록에있는 모든 값을 인쇄합니다.
Python에서 사전을 튜플 목록으로 변환
사전은 두 가지 방법을 사용하여 튜플 목록으로 변환 할 수 있습니다. 하나는items()
함수이고 다른 하나는zip()
함수입니다.
1. items()
함수
items()
함수는 사전 요소를<class 'dict_items'>
로 변환하고 그 결과는myList
변수에 저장됩니다. myList
를 목록으로 변환해야하므로list()
함수를 사용하여 유형 변환 한 다음 결과를 동일한 변수 즉,myList
에 저장해야합니다.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.items()
print(type(myList))
myList = list(myList)
print(myList)
출력:
<class 'dict_items'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
2. zip()
함수
zip()
함수는 먼저keys()
함수를 사용하는 키이고 두 번째는 사전에서values()
함수를 사용하여 값. 그런 다음 변수 (이 경우myList
) 내에 저장할 수있는zip
개체를 반환합니다.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = zip(dictionary.keys(), dictionary.values())
print(type(myList))
myList = list(myList)
print(myList)
출력:
<class 'zip'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
이제list()
함수를 사용하여zip
객체를list
로 변환해야합니다. 그런 다음 최종 결과를myList
라는 동일한 변수에 저장하거나 요구 사항에 따라 다른 변수에 저장하고 마지막에 전체 목록을 인쇄 할 수 있습니다.
3. Python 2.x의iteritems()
Python v2.x에는items()
함수와 거의 동일한 방식으로 작동하는iteritems()
함수도 있습니다.
iteritems()
는dictionary
에서 각 요소를 가져 와서 다른 유형으로 변환합니다. 변수myList
의 유형을 확인하면<type 'dictionary-itemiterator'>
유형이됩니다. 따라서list()
함수를 사용하여 목록으로 변환해야합니다. 변수myList
의 유형을 확인하면<type 'list'>
유형이되고 인쇄하면 요소가 튜플 목록의 형태가됩니다.
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.iteritems()
print("Type of myList is: ", type(myList))
myList = list(myList)
print(myList)
출력:
('Type of myList is: ', <type 'dictionary-itemiterator'>)
[('a', 'Apple'), ('c', 'Cherries'), ('b', 'Banana'), ('d', 'Dragon Fruit')]
Python v2.x 컴파일러에서 위 코드를 실행해야합니다. 그렇지 않으면 Python v3.x에이 메서드가 없기 때문에 오류가 발생합니다.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn관련 문장 - Python Dictionary
- 파이썬에서 키가 사전에 있는지 확인하는 방법
- 디렉토리의 모든 파일을 얻는 방법
- 파이썬 사전에서 최대 값을 찾는 방법
- 값으로 파이썬 사전을 정렬하는 방법
- 파이썬 2와 3에서 두 개의 사전을 병합하는 방법