파이썬 2와 3에서 두 개의 사전을 병합하는 방법
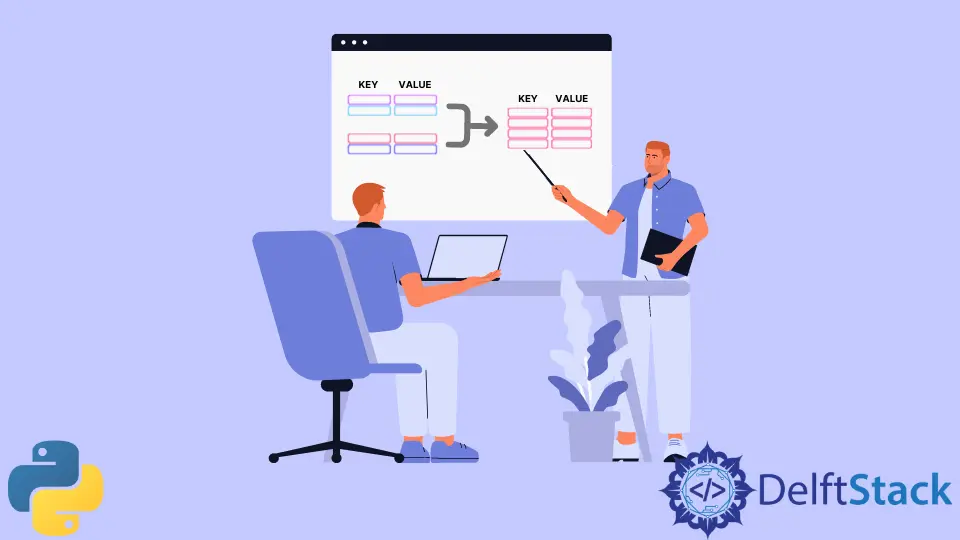
병합 할 두 사전 A
와 B
가 있다고 가정합니다. 여기서 B
의 값은 동일한 key
를 공유하는 경우 A
의 값을 대체합니다.
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
파이썬 사전
객체에는 사전 A
를 B
로 업데이트하는 내장 메소드 update()
메소드가 있습니다.
A.update(B)
그러나 A
와 B
가 포함 된 새 사전을 반환하는 대신 A
의 데이터가 대체됩니다.
두 사전을 병합하고 새로운 사전을 반환하는 방법을 소개합니다.
파이썬 2.7 사전 병합
딕셔너리 컴프리헨션-1
C = {key: value for d in (A, B) for key, value in d.items()}
d.itmes()
는(키, 값)
쌍의 목록을 사전 d
의 2 튜플로 반환합니다.
이 방법은 딕셔너리 컴프리헨션를 사용하여 두 사전을 병합합니다. for
의 올바른 순서는 특별한주의를 기울여야합니다. 그것은해야한다,
flattern_patterns = [planet for sublist in planets for planet in sublist]
딕셔너리 컴프리헨션-2
dict() 메소드를 사용하여 새 사전을 초기화 할 수도 있습니다.
C = dict((key, value) for d in (A, B) for key, value in d.items())
기술적으로 말하면, 위의 방법과 거의 동일하지만 나중에 언급 할 성능이 다릅니다.
itertools.chain
메소드
itertools
모듈은 iterator
빌딩 블록의 핵심 세트를 표준화합니다. 빠르고 메모리 효율적인 기능이 있습니다.
itertools.chain
은.next()
메소드가 첫 번째 iterable 에서 소진 될 때까지 요소를 리턴하고 다음 iterable(s) 이 모두 소진 될 때까지 요소를 리턴하는 체인 오브젝트를 리턴합니다.
dict(itertools.chain(A.iteritems(), B.iteritems()))
iteritems()
는 사전의(key, value)
항목에 대한 반복자를 반환합니다.
따라서 위의 스크립트는 A 와 B 의 항목을 포함하는 사전을 반환합니다.
copy
및업데이트
방법
처음에 언급했듯이 update()
는 A
와 B
를 병합 할 수 있지만 사전을 그 자리에서 대체합니다. copy()
메소드를 사용하여 사전 A
의 사본을 만들 수 있습니다.
m = A.copy()
C = m.update(B)
병합 방법 성능 분석 및 비교
import timeit
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
SETUP_CODE = """
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
{key: value for d in (A, B) for key, value in d.items()}
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
TEST_CODE = """
dict((key, value) for d in (A, B) for key, value in d.items())
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
TEST_CODE = """
dict(itertools.chain(A.iteritems(), B.iteritems()))
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
SETUP_CODE = """
def merge_dicts(a, b):
m = a.copy()
m.update(b)
return m
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
merge_dicts(A, B)
"""
print min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000))
결과는
0.0162378
0.029774
0.019975
0.0110059
방법 | 공연 | 계급 |
---|---|---|
{key: value for d in (A, B) for key, value in d.items()} |
0.0162378 | 2 |
dict((key, value) for d in (A, B) for key, value in d.items()) |
0.029774 | 4 |
dict(itertools.chain(A.iteritems(), B.iteritems())) |
0.019975 | 삼 |
merge_dicts(a, b) |
0.0110059 | 1 |
Python 3.5 이상 사전 병합 방법
Python 3.5부터는 Python 2.7에서와 동일한 방법 외에도 PEP-448. 임의의 수의 품목을 개봉 할 수 있습니다.
d.iteritems()
는 Python 3에서 더 이상 사용되지 않습니다. PEP-469 참조>>> C = {**A, **B}
>>> C
{'x': 10, 'y': 30, 'z': 40}
import timeit
A = {"x": 10, "y": 20}
B = {"y": 30, "z": 40}
SETUP_CODE = """
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
{**A, **B}
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
{key: value for d in (A, B) for key, value in d.items()}
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
dict((key, value) for d in (A, B) for key, value in d.items())
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
TEST_CODE = """
dict(itertools.chain(A.items(), B.items()))
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
SETUP_CODE = """
def merge_dicts(a, b):
m = a.copy()
m.update(b)
return m
A = {'x': 10, 'y': 20}
B = {'y': 30, 'z': 40}
"""
TEST_CODE = """
merge_dicts(A, B)
"""
print(min(timeit.repeat(setup=SETUP_CODE, stmt=TEST_CODE, repeat=3, number=10000)))
0.0017047999999999508
0.009127499999999955
0.0168952
0.01078009999999996
0.005767999999999995
방법 | 공연 | 계급 |
---|---|---|
{**A, **B} |
0.0017047999999999508 | 1 |
{key: value for d in (A, B) for key, value in d.items()} |
0.009127499999999955 | 삼 |
dict((key, value) for d in (A, B) for key, value in d.items()) |
0.0168952 | 5 |
dict(itertools.chain(A.items(), B.items())) |
0.01078009999999996 | 4 |
merge_dicts(a, b) |
0.005767999999999995 | 2 |
병합 방법 결론
파이썬 2.7에서는 copy
와 update
가 최선의 방법입니다.
m = A.copy()
C = m.update(B)
Python 3.5 이상에서는 사전 압축 풀기 방법이 가장 좋습니다.
{**A, **B}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook