在 Python 中將字典轉換為列表
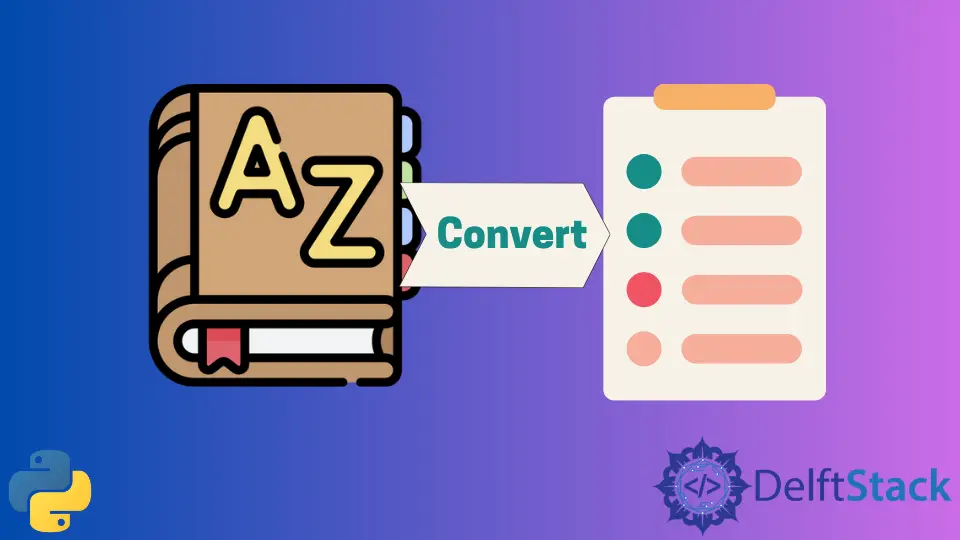
Python 中的字典是由鍵-值
對組合而成。在 key
的幫助下,你可以訪問字典裡面的值。現在,在 Python 中把一個字典轉換為一個列表可能意味著不同的事情。要麼是
- 你可以將鍵分開,並將其轉換為一個列表。
- 你可以將值分開,並將其轉換為一個列表。
- 將字典中的鍵和值對轉換為元組列表。
在 Python 中使用 keys()
方法將鍵從字典轉換為列表
假設你有一個名為 dictionary
的字典,有四鍵值對。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfKeys = dictionary.keys()
print("Type of variable listOfKeys is: ", type(listOfKeys))
listOfKeys = list(listOfKeys)
print("Printing the entire list containing all Keys: ")
print(listOfKeys)
print("Printing individual Keys stored in the list:")
for key in listOfKeys:
print(key)
輸出:
Type of variable listOfKeys is: <class 'dict_keys'>
Printing the entire list containing all Keys:
['a', 'b', 'c', 'd']
Printing individual Keys stored in the list:
a
b
c
d
你可以使用 keys()
方法,它將從字典中提取所有 keys
,然後將結果(所有 keys)儲存在變數 listOfKeys
中。
現在你必須在 list()
函式的幫助下將變數 listOfKeys
轉換成一個列表,因為此時如果你檢查變數 listOfKeys
的型別,那麼它將是 <class 'dict_keys'>
。這就是為什麼我們要將變數的型別改為 List
,然後將其儲存在同一個變數中,即 listOfKeys
。
至此,你已經成功地使用 keys()
函式將所有鍵從字典中分離出來,並將它們轉換為一個列表。最後,要列印列表中的元素,你必須使用 for
迴圈,它遍歷每個元素並列印它。
在 Python 中使用 values()
方法將字典中的值轉換為列表
values()
方法的工作方式與 keys()
方法相同。唯一的區別是 values()
方法返回所有的字典值而不是鍵。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
listOfValues = dictionary.values()
print("Type of variable listOfValues is: ", type(listOfValues))
listOfValues = list(listOfValues)
print("Printing the entire list containing all values: ")
print(listOfValues)
print("Printing individual values stored in the list:")
for val in listOfValues:
print(val)
輸出:
Type of variable listOfValues is: <class 'dict_values'>
Printing the entire list containing all values:
['Apple', 'Banana', 'Cherries', 'Dragon Fruit']
Printing individual values stored in the list:
Apple
Banana
Cherries
Dragon Fruit
你在 dictionary
上使用 values()
函式,然後將 values()
函式返回的結果儲存在變數 listOfValues
中。
如果你檢查變數 listOfValues
的型別,那麼它的型別將是 <class 'dict_values'>
類。但你希望它是 <class 'list'>
類。因此,你必須使用 list()
函式將變數 listOfValues
轉換為一個列表,然後將結果儲存在同一個變數中,即 listOfValues
。
最後,使用迴圈列印列表 listOfValues
內的所有值。
在 Python 中把字典轉換為元組列表
一個字典可以通過兩種方式轉換成一個元組列表。一種是 items()
函式,另一種是 zip()
函式。
1. items()
函式
items()
函式將字典元素轉換為 <class 'dict_items'>
,其結果儲存在 myList
變數中。myList
需要被轉換為一個列表,因此,你必須使用 list()
函式將其型別化,然後將結果儲存在同一個變數中,即 myList
。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.items()
print(type(myList))
myList = list(myList)
print(myList)
輸出:
<class 'dict_items'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
2. zip()
函式
zip()
函式需要兩個引數,第一個是使用 keys()
函式的 key,第二個是使用字典中的 values()
函式的 value。然後它返回一個 zip
物件,你可以將其儲存在一個變數中,在本例中,myList
。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = zip(dictionary.keys(), dictionary.values())
print(type(myList))
myList = list(myList)
print(myList)
輸出:
<class 'zip'>
[('a', 'Apple'), ('b', 'Banana'), ('c', 'Cherries'), ('d', 'Dragon Fruit')]
現在你必須使用 list()
函式將 zip
物件轉換成 list
。然後,你可以將最終的結果儲存在同一個名為 myList
的變數中,或者根據你的要求儲存在不同的變數中,最後列印整個列表。
3. Python 2.x 中的 iteritems()
在 Python v2.x 中,你還有一個 iteritems()
函式,它的工作方式和 items()
函式幾乎一樣。
iteritems()
從 dictionary
中獲取每個元素並將其轉換為不同的型別。如果你檢查變數 myList
的型別,它將是 <type 'dictionary-itemiterator'>
型別。所以,你必須使用函式 list()
將其轉換為 List。如果你檢查變數 myList
的型別,那麼它的型別將是 <type 'list'>
,如果你列印它,那麼元素將以一個元組列表的形式出現。
dictionary = {"a": "Apple", "b": "Banana", "c": "Cherries", "d": "Dragon Fruit"}
myList = dictionary.iteritems()
print("Type of myList is: ", type(myList))
myList = list(myList)
print(myList)
輸出:
('Type of myList is: ', <type 'dictionary-itemiterator'>)
[('a', 'Apple'), ('c', 'Cherries'), ('b', 'Banana'), ('d', 'Dragon Fruit')]
確保你在 Python v2.x 編譯器上執行上述程式碼;否則,你會得到一個錯誤,因為在 Python v3.x 中沒有此方法。
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn