如何從 Python 字典中刪除元素
Aliaksei Yursha
2023年1月30日
Python
Python Dictionary
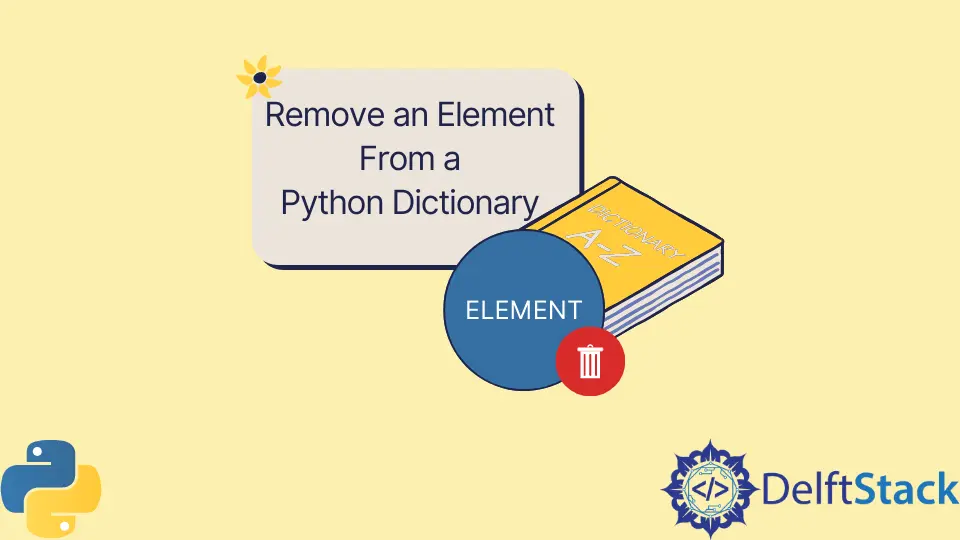
有時,我們需要刪除 Python 字典包含一個或多個鍵值。你可以通過幾種不同的方式來做到這一點。
刪除 Python 字典元素的 del
語句
一種方法是使用 Python 的內建 del
語句。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> del meal['fats']
>>> meal
{'proteins': 10, 'carbohydrates': 80}
請注意,如果你嘗試刪除字典中不存在的鍵,則 Python 執行時將丟擲 KeyError
。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> del meal['water']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'water'
刪除 Python 字典元素的 dict.pop()
方法
另一種方法是使用 dict.pop()
方法。此方法的好處是,如果字典中不存在所請求的鍵,則可以指定以預設值來返回。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> meal.pop('water', 3000)
3000
>>> meal.pop('fats', 3000)
10
>>> meal
{'proteins': 10, 'carbohydrates': 80}
請注意,如果你不提供要返回的預設值,並且所請求的鍵不存在,你還將收到執行時錯誤,如上面的 del
。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> meal.pop('water')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'water'
從 Python 字典中刪除多個元素
如果你需要一次刪除字典中的多個元素,Python 3 提供了方便的字典推導式功能,你可以在此處應用字典推導來刪除字典中的元素。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> [meal.pop(key) for key in ['fats', 'proteins']]
[10, 10]
>>> meal
{'carbohydrates': 80}
請注意,使用上述方法,如果其中給定的鍵值不在字典中,Python 仍將會報錯。為避免此問題,你可以給 dict.pop()
另一個引數作為預設返回值。
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> [meal.pop(key, None) for key in ['water', 'sugars']]
[None, None]
>>> meal
{'fats': 10, 'proteins': 10, 'carbohydrates': 80}
效能特點
使用 del
語句和 dict.pop()
方法具有不同的效能特徵。
>>> from timeit import timeit
>>> timeit("meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}; del meal['fats']")
0.12977536499965936
>>> timeit("meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}; meal.pop('fats', None)")
0.21620816600079706
如下所示,del
語句的速度幾乎快一倍。
但是,dict.pop()
使用預設值會更安全,因為它將幫助你避免執行時錯誤。
如果你確信關鍵字必須存在於字典中,請使用前者,否則,請選擇後者。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe