How to Remove an Element From a Python Dictionary
-
Use the
del
Statement to Remove Python Dictionary Element -
the
dict.pop()
Function to Remove Python Dictionary Element - Delete Multiple Elements From a Python Dictionary
- Performance Characteristics
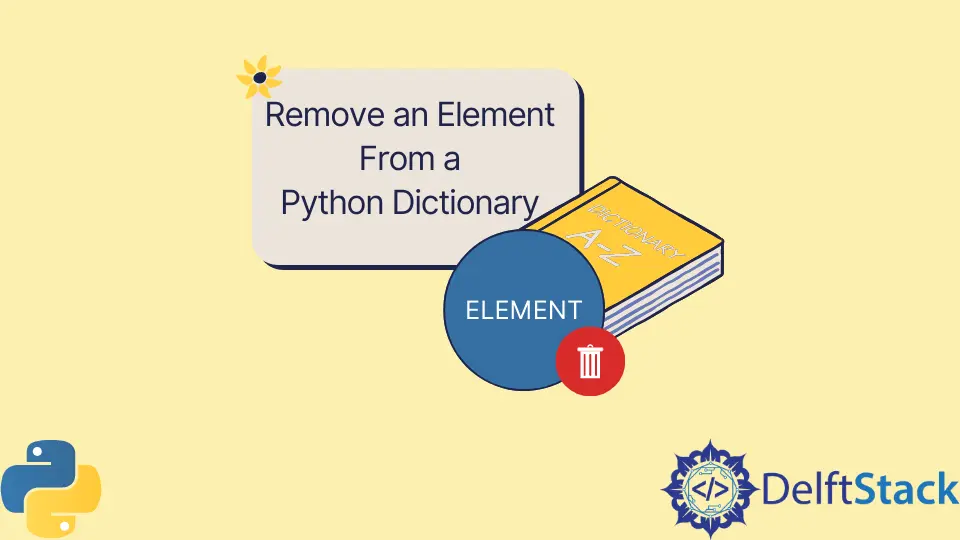
Sometimes your Python dictionary contains a key or several keys that you want to delete. You can do it in several different ways.
Use the del
Statement to Remove Python Dictionary Element
One approach is to use Python’s built-in del
statement.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> del meal['fats']
>>> meal
{'proteins': 10, 'carbohydrates': 80}
Note that if you try to delete an element by a key that is not present in the dictionary, Python runtime will throw a KeyError
.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> del meal['water']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'water'
the dict.pop()
Function to Remove Python Dictionary Element
Another approach is to use the dict.pop()
function.
The upside of this method is that it allows you to specify
the default value to return if the requested key does not exist
in the dictionary.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> meal.pop('water', 3000)
3000
>>> meal.pop('fats', 3000)
10
>>> meal
{'proteins': 10, 'carbohydrates': 80}
Note that if you don’t provide the default value to return and the requested
key doesn’t exist, you will also get a runtime error, like del
above.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> meal.pop('water')
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'water'
Delete Multiple Elements From a Python Dictionary
If you need to delete multiple elements from a dictionary in one go,
Python 3 offers handy list comprehensions, which you can employ for this purpose.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> [meal.pop(key) for key in ['fats', 'proteins']]
[10, 10]
>>> meal
{'carbohydrates': 80}
Note that with the above approach, Python will still crash if one of the passed-in keys is not in the dictionary.
To circumvent this issue, you may give a second argument to dict.pop()
as a default return value.
>>> meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}
>>> [meal.pop(key, None) for key in ['water', 'sugars']]
[None, None]
>>> meal
{'fats': 10, 'proteins': 10, 'carbohydrates': 80}
Performance Characteristics
Using del
statement and dict.pop()
have different performance characteristics.
>>> from timeit import timeit
>>> timeit("meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}; del meal['fats']")
0.12977536499965936
>>> timeit("meal = {'fats': 10, 'proteins': 10, 'carbohydrates': 80}; meal.pop('fats', None)")
0.21620816600079706
As follows from the timings above, the del
statement is almost twice as fast.
However, dict.pop()
with a fallback value is safer as it will help you to avoid runtime errors.
Use the former if you are confident that keys must be present in the dictionary, and prefer the latter otherwise.