How to Find Maximum Value in Python Dictionary
-
Use the
operator.itemgetter()
Method to Get Key With Maximum Value -
Use the
dict.items()
Method for Python 3.x to Get Key With Maximum Value in Dictionary - a General and Memory Efficient Solution to Get Key With Maximum Value in Dictionary
-
Use
max()
anddict.get()
Methods to Obtain Key With Maximum Value in Dictionary
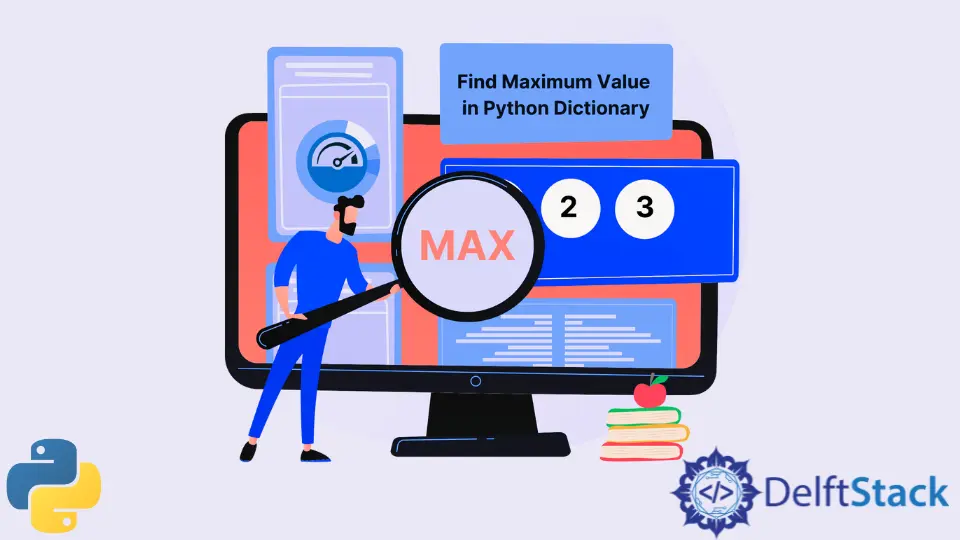
This tutorial describes how to get a key with maximum value in Python dictionary. It also lists some example codes to clarify the concepts as the method has changed from previous Python versions.
Use the operator.itemgetter()
Method to Get Key With Maximum Value
We don’t need to create a new list to iterate over the dictionary’s (key, value)
pair. We can use stats.iteritems()
for this purpose. It returns an iterator over the (key, value)
pair of the dictionary.
You can use the operator.itemgetter(x)
method to get the callable object that will return the x-th
element from the object. Here because the object is the (key, value)
pair, operator.itemgetter(1)
refers to the element at the index of 1
, that is, the value
.
As we want a key with a maximum value, so we will encapsulate the methods in the max
function.
A basic example code for this method is given below:
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats.iteritems(), key=operator.itemgetter(1))[0]
print(max_key)
Output:
key3
Also, please note that iteritems()
can raise a RunTimeException
while adding or removing items from the dictionary, and dict.iteritems
is removed in Python 3.
Use the dict.items()
Method for Python 3.x to Get Key With Maximum Value in Dictionary
In Python 3.x, you can use the dict.items()
method to iterate over key-value
pairs of the dictionary. It is the same method as dict.iteritems()
in Python 2.
An example code for this method is given below:
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats.items(), key=operator.itemgetter(1))[0]
print(max_key)
Output:
key3
a General and Memory Efficient Solution to Get Key With Maximum Value in Dictionary
Interestingly, there is another solution that works for both Python 2 and Python 3. The solution uses the lambda
function to obtain the key, and the max
method to ensure the obtained key
is maximum.
The basic code for this approach is given below:
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats, key=lambda key: stats[key])
print(max_key)
Output:
key3
Use max()
and dict.get()
Methods to Obtain Key With Maximum Value in Dictionary
Another solution to this problem can be simply using the built-in max()
method. It is provided with the stats
to obtain maximum value from, and to return the key
with maximum value, the dict.get()
method is used.
An example code is given below:
import operator
stats = {"key1": 20, "key2": 35, "key3": 44}
max_key = max(stats, key=stats.get)
print(max_key)
Output:
key3
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn