How to Remove All the Occurrences of an Element From a List in Python
-
Use the
filter()
Function to Remove All the Instances of an Element From a List in Python - Use List Comprehension to Remove All the Instances of an Element From a List in Python
-
Use the
remove()
Function to Remove All the Instances of an Element From a List in Python - Comparing the Methods
- Conclusion
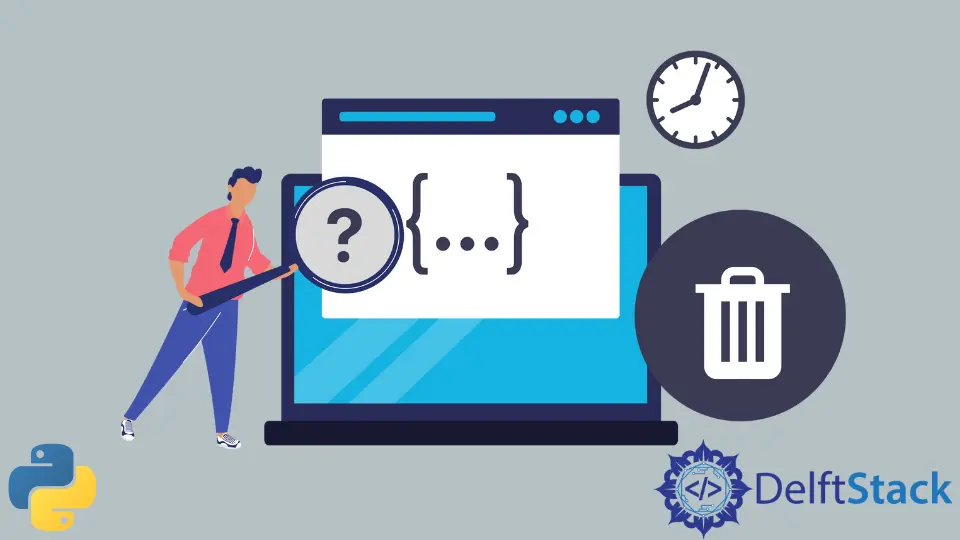
A list in Python allows multiple occurrences of the same element. Even though an element’s value may be the same as others, each element will have a different index.
Using these index numbers, you can easily access whichever elements you want.
But there might be some situations where you don’t want multiple instances of the same element; then, you will surely want to remove all of those occurrences of that particular element from a list. In this article, we’ll explore four different ways in which to achieve this.
Use the filter()
Function to Remove All the Instances of an Element From a List in Python
In Python, filtering elements becomes easier with the help of the filter()
function. The filter()
function takes two arguments; the first argument is a function, and the second argument can be sets, lists, tuples, etc.
The filter()
function in Python is used to filter elements from an iterable based on a specified function or condition.
Syntax:
filter(function, iterable)
function
: This is the function that tests whether each element of the iterable satisfies a certain condition. It is called for each element, and if the function returnsTrue
, the element is included in the result.iterable
: This is the iterable (e.g., a list, tuple, or string) from which elements are tested and selected by thefunction
.
To better understand how to use this function, we will discuss some examples.
Example 1: filter()
Function With __ne__
Consider a scenario where we have a list of prime numbers, and the objective is to remove all occurrences of a specific prime number. Below is a concise Python script achieving this using the filter()
function with the __ne__
method.
Example:
prime_numbers = [2, 3, 5, 7, 2, 11, 2, 13]
prime_numbers = list(filter((2).__ne__, prime_numbers))
print(prime_numbers)
In this example, we start by creating a list of prime numbers named prime_numbers
, which contains repeated instances of the prime number 2
.
Next, the filter()
function is used to create a new list (prime_numbers
) by excluding elements that are equal to the target prime number (2
). The __ne__
method is used to implement the not equal to comparison to check if the list contains 2
that is to be removed from the list.
Finally, the print()
function is used to print the modified list on the console.
Output:
[3, 5, 7, 11, 13]
After executing the script, the output will be [3, 5, 7, 11, 13]
, demonstrating the successful removal of all instances of the target prime number (2
) from the original list.
The filter()
function, coupled with the __ne__
method, provides an elegant and expressive way to selectively include or exclude elements from a list based on a specified condition. As showcased in this article, this approach is valuable for efficient element removal, contributing to the arsenal of techniques available for Python list manipulation.
Example 2: filter()
Function With lambda
Function Code Example
Below is a complete working code example that demonstrates how to use the filter()
function with a lambda
function to remove all instances of an element from a list.
Example:
numbers = [1, 2, 3, 4, 2, 5, 2]
element_to_remove = 2
new_list = list(filter(lambda x: x != element_to_remove, numbers))
print(new_list)
In this example, we start by creating a list of numbers, including the element we want to remove. Then, we define the element we want to remove from the list.
We use the filter()
function with a lambda
function to create a new list that excludes the specified element. The lambda
function checks if each element is not equal to the element we want to remove.
The filter()
function takes two arguments: the function to be applied for each item in the iterable (a lambda
function) and the iterable to be filtered (numbers
). It returns an iterator that yields the items for which the function returns True
.
Finally, we print the new_list
to demonstrate the effectiveness of using the filter()
function with a lambda
function to remove all instances of an element from a list.
Output:
[1, 3, 4, 5]
As seen in the output, we successfully removed the element 2
from the list using the filter()
function.
In this example, we demonstrated how to use the filter()
function with a lambda
function to remove all instances of an element from a list. The resulting list contains only the elements that satisfy the condition specified in the lambda
function, demonstrating the effectiveness of the filter()
function with a lambda
function.
The filter()
function in Python provides a concise and efficient way to extract values from an iterable based on a given condition.
Use List Comprehension to Remove All the Instances of an Element From a List in Python
A list comprehension is a short way of writing code. List comprehension is faster than the normal functions and loops.
The basic syntax of list comprehension is as follows:
new_list = [expression for item in iterable if condition]
expression
: The expression is evaluated for each item in the iterable, and the results are collected into a new list.item
: This represents each element in the iterable (e.g., a list, tuple, or string).iterable
: The iterable from which elements are taken.condition
(optional): This is an optional part of list comprehension. If specified, it filters elements based on a certain condition.
Below is a complete working code example that demonstrates how to use list comprehension to remove all instances of an element from a list.
Example:
original_list = [1, 2, 3, 4, 2, 5, 2]
element_to_remove = 2
new_list = [x for x in original_list if x != element_to_remove]
print(new_list)
In this code snippet, we start by initializing the original_list
with some elements, including the element we want to remove (2
).
List comprehension is then used to create a new list, new_list
, that contains elements from the original_list
that is not equal to the element_to_remove
(2
). The syntax for list comprehension is square brackets enclosing an expression followed by a for
clause and an optional if
clause.
Finally, the new_list
that contains all the elements from the original_list
that are not equal to the element_to_remove
is printed to the console using the print()
function.
Output:
[1, 3, 4, 5]
List comprehension is a powerful and concise way to create a new list in Python. It allows you to filter or transform elements from an existing list, making it a versatile tool for various programming tasks.
In this example, we demonstrated how to use list comprehension to remove all instances of an element from a list. The resulting list contains all the elements from the original list that are not equal to the element we want to remove, demonstrating the effectiveness of list comprehension.
Use the remove()
Function to Remove All the Instances of an Element From a List in Python
The remove()
function is another way to remove all the instances of an element from a list in Python.
However, remove()
only removes the first occurrence of the element. If you want to remove all the occurrences of an element using the remove()
function, you can use a loop, either a for
loop or a while
loop.
Let’s consider a practical scenario where we have a list of numbers and a desire to remove all occurrences of a specific number.
Example:
numbers = [1, 2, 3, 4, 2, 5, 2, 6]
while 2 in numbers:
numbers.remove(2)
print(numbers)
In this example, we start by creating a list of numbers named numbers
, which contains repeated instances of the number 2
.
A while
loop is then used to continually check if the target element (2
) is present in the list. The loop iterates until all occurrences of the target element are removed.
Inside the while
loop, the remove
method is used directly on the list (numbers
) to eliminate the target element (2
). Following the removal operation, the modified list is printed to the console using the print()
function.
Output:
[1, 3, 4, 5, 6]
After executing the script, the output will be [1, 3, 4, 5, 6]
, illustrating the successful removal of all instances of the target element (2
) from the original list.
The remove
method offers a concise and intuitive approach to achieve this task. Whether you’re a novice or an adept Python developer, mastering this method enhances your ability to manipulate lists with precision and confidence.
Comparing the Methods
In the realm of Python list manipulation, three potent methods for element removal have been explored: the remove()
method, list comprehension, and the filter()
function, employed both with the __ne__
method and with a lambda function. Each method offers a unique approach, addressing various scenarios and preferences.
-
remove()
Method:- Strengths:
- Directly modifies the original list.
- Simple and intuitive for single-element removal.
- Considerations:
- Removes only the first occurrence of the specified element.
- Raises a
ValueError
if the element is not present.
- Strengths:
-
List Comprehension:
- Strengths:
- Creates a new list, preserving the original.
- Provides expressive syntax for concise filtering.
- Considerations:
- May consume more memory for large lists.
- Ideal for creating a filtered version of the list.
- Strengths:
-
filter()
Function with__ne__
Method:- Strengths:
- Creates a new list, leaving the original intact.
- Offers a functional programming paradigm.
- Considerations:
- Requires explicit conversion from a filter object to a list.
- Strengths:
-
filter()
Function withlambda
:- Strengths:
- Provides flexibility with custom filtering conditions.
- Supports a concise inline approach.
- Considerations:
- Less readable for complex conditions compared to list comprehension.
- Strengths:
Conclusion
In Python, we explored different ways to remove all instances of an element from a list: the remove()
method, list comprehension, the filter()
function with __ne__
, and the filter()
function with lambda
.
Each method has strengths, so choose based on your needs. Whether you prefer modifying the original list or creating a new one, Python provides versatile tools for efficient list manipulation.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn