Python Numpy.mean() - Arithmetic Mean
-
Syntax of
numpy.mean()
-
Example Codes:
numpy.mean()
With 1-D Array -
Example Codes:
numpy.mean()
With 2-D Array -
Example Codes:
numpy.mean()
Withdtype
Specified
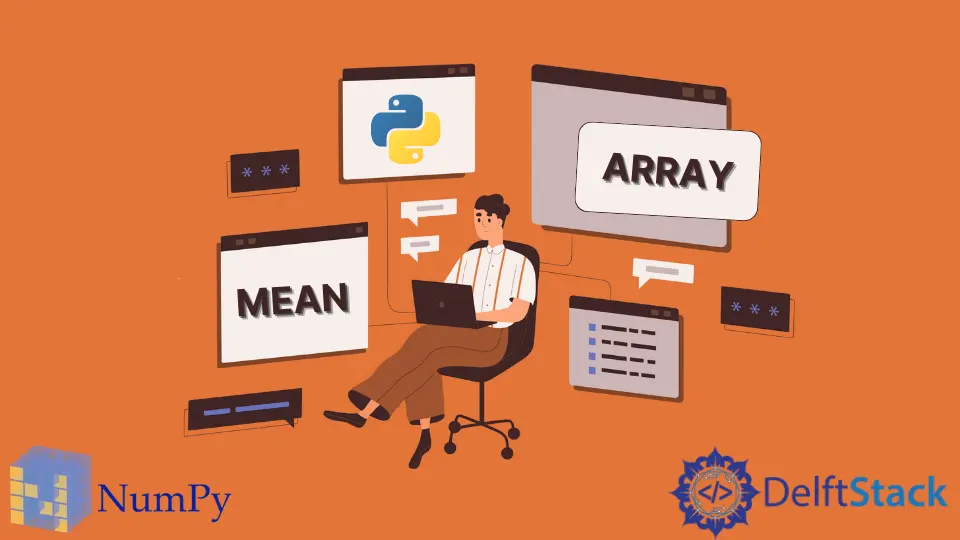
Numpy.mean()
function calculates the arithmetic mean, or in layman words - average, of the given array along the specified axis.
Syntax of numpy.mean()
numpy.mean(arr, axis=None, dtype=float64)
Parameters
arr |
array_like input array to calculate the arithmetic mean |
axis |
None , int or tuple of int Axis along which thearithmetic mean is computed. axis=0 means arithmetic mean computed along the column, axis=1 means arithmetic mean along the row. It treats the multiple dimension array as a flattened list if axis is not given. |
dtype |
dtype or None Data type used during the calculation of the arithmetic mean. Default is float64 |
Return
It returns the arithmetic mean of the given array or an array with the arithmetic mean along the specified axis.
Example Codes: numpy.mean()
With 1-D Array
import numpy as np
arr = [10, 20, 30]
print("1-D array :", arr)
print("Mean of arr is ", np.mean(arr))
Output:
1-D array : [10, 20, 30]
Mean of arr is 20.0
Example Codes: numpy.mean()
With 2-D Array
import numpy as np
arr = [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
print("Two Dimension array :", arr)
print("Mean with no axis :", np.mean(arr))
print("Mean with axis along column :", np.mean(arr, axis=0))
print("Mean with axis aong row :", np.mean(arr, axis=1))
Output:
Two Dimension array : [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
Mean with no axis : 57.333333333333336
Mean with axis along column : [45.75 65. 61.25]
Mean with axis aong row : [ 20. 19.33333333 80. 110. ]
>>
np.mean(arr)
treats the input array as the flattened array and calculates the arithmetic mean of this 1-D flattened array.
np.mean(arr, axis=0)
calculates the arithmetic mean along the column.
np.std(arr, axis=1)
calculates the arithmetic mean along the row.
Example Codes: numpy.mean()
With dtype
Specified
import numpy as np
arr = [10.12, 20.3, 30.28]
print("1-D Array :", arr)
print("Mean of arr :", np.mean(arr))
print("Mean of arr with float32 data :", np.mean(arr, dtype=np.float32))
print("Mean of arr with float64 data :", np.mean(arr, dtype=np.float64))
Output:
1-D Array : [10.12, 20.3, 30.28]
Mean of arr : 20.233333333333334
Mean of arr with float32 data : 20.233332
Mean of arr with float64 data : 20.233333333333334
If dtype
parameter is given in the numpy.mean()
function, it uses the specified data type during the computing of arithmetic mean.
The result has a lower resolution if we use float32
data type rather than the default float64
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook