Python Numpy.std() - Standard Deviation Function
-
Syntax of
numpy.std()
-
Example Codes:
numpy.std()
With 1-D Array -
Example Codes:
numpy.std()
With 2-D Array -
Example Codes:
numpy.std()
Withdtype
Specified
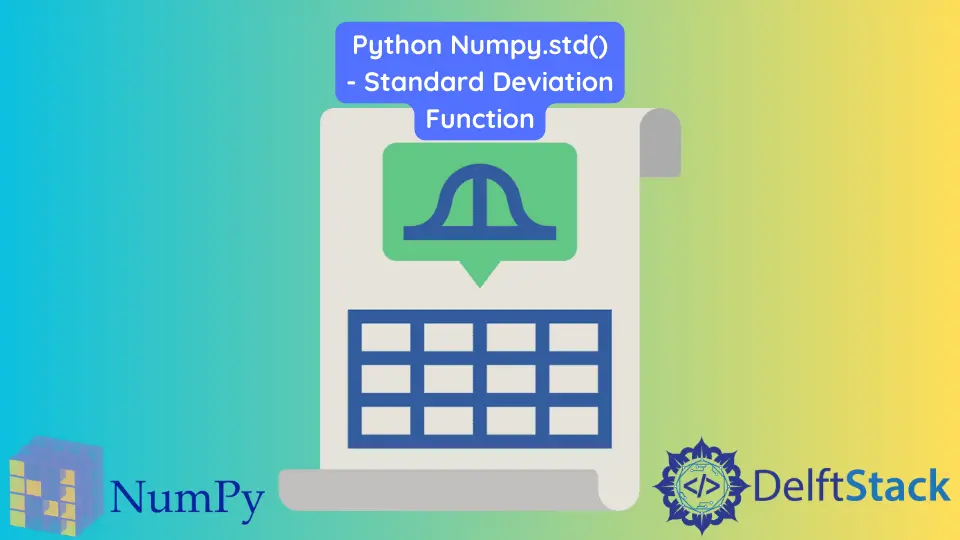
Numpy.std()
function calculates the standard deviation of the given array along the specified axis.
Syntax of numpy.std()
numpy.std(arr, axis=None, dtype=float64)
Parameters
arr |
array_like input array to calculate the standard deviation |
axis |
None , int or tuple of int Axis along which the standard deviation is computed. axis=0 means standard deviation computed along the column, axis=1 means standard deviation along the row. It treats the multiple dimension array as a flattened list if axis is not given. |
dtype |
dtype or None Data type used during the calculation of the standard deviation. |
Return
It returns the standard deviation of the given array, or an array with the standard deviation along the specified axis.
Example Codes: numpy.std()
With 1-D Array
When the Python 1-D array is the input, Numpy.std()
function calculates the standard deviation of all values in the array.
import numpy as np
arr = [10, 20, 30]
print("1-D array :", arr)
print("Standard Deviation of arr is ", np.std(arr))
Output:
1-D array : [10, 20, 30]
Standard deviation of arr is 8.16496580927726
Here, the 1-D array has the elements of 10, 20, and 30; therefore, the value in the returned DataFrame
is the standard deviation without assigning any axis info.
Example Codes: numpy.std()
With 2-D Array
import numpy as np
arr = [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
print("Two Dimension array :", arr)
print("SD of with no axis :", np.std(arr))
print("SD of with axis along column :", np.std(arr, axis=0))
print("SD of with axis aong row :", np.std(arr, axis=1))
Output:
Two Dimension array : [[10, 20, 30], [3, 50, 5], [70, 80, 90], [100, 110, 120]]
SD of with no axis : 41.21960159384798
SD of with axis along column : [40.73312534 33.54101966 45.87687326]
SD of with axis aong row : [ 8.16496581 21.6999744 8.16496581 8.16496581]
np.std(arr)
treats the input array as the flattened array and calculates the standard deviation of this 1-D flattened array.
np.std(arr, axis=0)
calculates the standard deviation along the column. It returns [40.73312534 33.54101966 45.87687326]
as the standard deviation of each column in the input array.
np.std(arr, axis=1)
calculates the standard deviation along the row. It returns [8.16496581 21.6999744 8.16496581 8.16496581]
as the standard deviation of each row in the input array.
Example Codes: numpy.std()
With dtype
Specified
import numpy as np
arr = [10, 20, 30]
print("Single Dimension array :", arr)
print("SD of Single Dimension array :", np.std(arr))
print("SD value with float32 data :", np.std(arr, dtype=np.float32))
print("SD value with float64 data :", np.std(arr, dtype=np.float64))
Output:
Single Dimension array : [10, 20, 30]
SD of Single Dimension array : 8.16496580927726
SD value with float32 data : 8.164966
SD value with float64 data : 8.16496580927726
If dtype
parameter is given in the numpy.std()
function, it uses the specified data type during the computing of standard deviation.
It is obvious to notice that the standard deviation has a lower resolution if we assign dtype
with float32
rather than float64
.
