How to Split String Into Array by Comma in JavaScript
-
Use the
split()
Method to Split String Into Array by Comma in JavaScript -
Use the
split()
Method With theArray
Class Object to Split String Into Array by Comma in JavaScript - Use a User-Defined Function to Split String Into Array by Comma in JavaScript
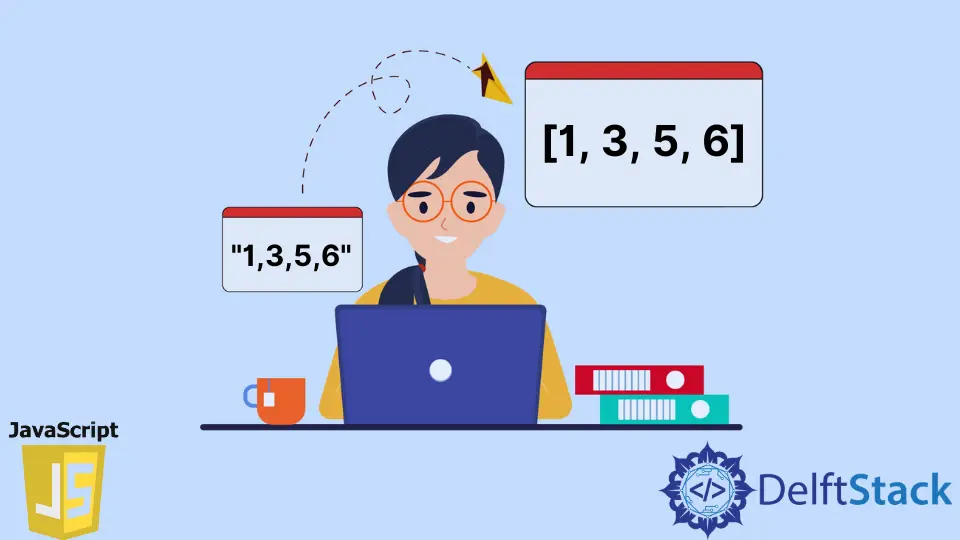
This article shows how to split a string into an array by a comma in JavaScript. For that, we can use the split()
method.
This method divides the specified String into an ordered list of the substring, saves these substrings into an array and returns that array.
It divides the string based on the separator
; it can be a single space
, comma
, colon
, or any other pattern. It means the split()
method splits a string at each point when the separator
is found in the provided string, puts each substring into an array and returns it (array).
Let’s start with the simplest example.
Use the split()
Method to Split String Into Array by Comma in JavaScript
Example Code:
var courses =
'Machine Learning, Python Programming, JavaScript Programming, Data Structures';
var array = courses.split(',');
// returns "object" because array is one of object's type.
console.log(typeof (array));
console.log(array);
Output:
"object"
["Machine Learning", " Python Programming", " JavaScript Programming", " Data Structures"]
In the upper code example, we have a string named courses
split into array by comma and saved the returned array into an array
variable.
To ensure that we have successfully converted it into an array, we can either check the type of the array
using typeof
or print it on the screen. We are using both ways in the above example.
We can also get similar results as given above by using the code given below.
var courses =
'Machine Learning, Python Programming, JavaScript Programming, Data Structures';
var array = courses.split`,`;
console.log(typeof (array));
console.log(array);
Output:
"object"
["Machine Learning", " Python Programming", " JavaScript Programming", " Data Structures"]
Use the split()
Method With the Array
Class Object to Split String Into Array by Comma in JavaScript
Example Code:
var intArray = '1,3,5,6';
var array = new Array();
array = intArray.split(',');
console.log(typeof (array));
console.log(array);
for (var element in array) {
array[element] = parseInt(array[element], 10);
}
console.log(typeof (array));
console.log(array);
Output:
"object"
["1", "3", "5", "6"]
"object"
[1, 3, 5, 6]
We have a string of numbers saved into the intArray
variable in the above snippet. Then, we create an object of the Array class to save the array returned by the split()
method.
Note that the split()
outputs the array of strings, but we are looking for an array of integers.
For that, we can loop over the array returned by the split()
method and convert them into integers using the parseInt()
method.
Remember, the array
variable type will not be changed. It is still an object
; see the above output.
Instead of for
loop, we can also use array’s map()
method with parseInt()
to convert into integers. The map()
method creates a new array by calling another function for each array element.
It calls the defined function once for every element of the array without changing the original array. Remember, it does not call a function for empty elements.
See the following code.
var intArray = '1,3,5,6';
var array = new Array();
array = intArray.split(',').map(element => parseInt(element, 10));
console.log(array);
Output:
[1, 3, 5, 6]
Use a User-Defined Function to Split String Into Array by Comma in JavaScript
Example Code:
var intArray = '1,3,5,6';
function splitTheStringByComma(CommaSeparatedString) {
var resultedArray = null;
if (CommaSeparatedString != null) {
var separator = ',';
if (CommaSeparatedString.indexOf(separator) >= 0) {
resultedArray = CommaSeparatedString.split(separator);
} else {
resultedArray = [CommaSeparatedString];
}
}
return resultedArray;
}
console.log(splitTheStringByComma(intArray));
Output:
["1", "3", "5", "6"]
In this example, we pass intArray
to the splitTheStringByComma()
function, which checks whether the string is null
or not. If it is not, then it moves ahead and sees if the string has a comma or not.
If yes, split each time when comma (,
) occurs and save it into resultedArray
; otherwise, go to else
and save the provided string into resultedArray
because there is a single element in the string.
Related Article - JavaScript String
- How to Get Last Character of a String in JavaScript
- How to Convert a String Into a Date in JavaScript
- How to Get First Character From a String in JavaScript
- How to Convert Array to String in JavaScript
- How to Check String Equality in JavaScript
- How to Filter String in JavaScript
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript